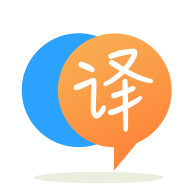
[英]@autowired for JwtBuilder class is not working, error: required a bean of type 'io.jsonwebtoken.JwtBuilder' that could not be found
[英]Autowired not working, error 'required a bean of type...'
我正在嘗試運行 Spring Boot 應用程序。 首先,我沒有用scanBasePackages在Application java中指明包。 所以我的應用程序運行,但我有這樣的問題: Spring 問題:有一個意外錯誤(類型 = 未找到,狀態 = 404)當我在應用程序類添加 scanBasePackages 時,我得到:
fr.umlv.orthopro.controller.UserController 中的字段 userService 需要一個無法找到的類型為“fr.umlv.orthopro.service.UserService”的 bean。
行動:
考慮在您的配置中定義一個“fr.umlv.orthopro.service.UserService”類型的 bean。
所以我找到了答案,這似乎是包裹的安排。 但是就像我在添加 scanBasePackages 注釋但沒有成功之前所說的那樣,即使我嘗試手動重組我的包,它也不起作用。 所以經過幾個小時之后,我無法弄清楚問題來自哪里。
我的包結構:
src/
├── main/
│ └── java/
| ├── fr.umlv.orthopro.app/
| | └── Application.java
| ├── fr.umlv.orthopro.controller/
| | ├── UserController.java
| | ├── SentenceController.java
| | └── RuleController.java
| ├── fr.umlv.orthopro.service/
| | ├── UserService.java
| | ├── UserServiceIT.java
| | ├── SentenceService.java
| | ├── SentenceServiceIT.java
| | ├── RuleService.java
| | └── RuleServiceIT.java
| └── fr.umlv.orthopro.db/
| ├── UserService.java
| ├── UserServiceIT.java
| └── SentenceService.java
這是我的 Application.java 文件(沒有導入)
import fr.umlv.orthopro.controller.UserController;
@SpringBootApplication(scanBasePackages={"fr.umlv.orthopro.app", "fr.umlv.orthopro.controller",
"fr.umlv.orthopro.db", "fr.umlv.orthopro.service"})
@ComponentScan(basePackageClasses=UserController.class)
public class OrthoproApp {
public static void main( String[] args ) throws Exception {
SpringApplication.run(OrthoproApp.class, args);
}
@Bean
public CommandLineRunner commandLineRunner(ApplicationContext ctx) {
return args -> {
System.out.println("Let's inspect the beans provided by Spring Boot:");
String[] beanNames = ctx.getBeanDefinitionNames();
Arrays.sort(beanNames);
for (String beanName : beanNames) {
System.out.println(beanName);
}
};
}
}
@RestController
@Component
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
...
}
package fr.umlv.orthopro.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import fr.umlv.orthopro.db.User;
@Service
@Transactional(propagation = Propagation.SUPPORTS, readOnly = true)
public abstract class UserService implements UserServiceIT{
@Autowired
private UserServiceIT userRepo;
@Override
public List<User> findAll() {
List<User> users = userRepo.findAll();
return users;
}
@Override
public User findById(int id) {
User user = userRepo.findOne(id);
return user;
}
@Override
@Transactional(propagation = Propagation.REQUIRED, readOnly = false)
public User create(String username, String password, String email, String first_name, String last_name, boolean admin)
throws NullPointerException, IllegalArgumentException {
User new_user = new User();
List<User> userLst = findAll();
for(User tmp_u:userLst) {
if(tmp_u.getUsername().equals(username))
throw new IllegalArgumentException("Username alredy exist");
}
new_user.setUsername(username);
new_user.setEmail(email);
new_user.setPassword(password);
new_user.setAdmin(admin);
new_user.setFirstName(first_name);
new_user.setLastName(last_name);
new_user = userRepo.save(new_user);
if(new_user == null)
throw new NullPointerException("Save user has failed");
return new_user;
}
@Override
@Transactional(propagation = Propagation.REQUIRED, readOnly = false)
public User update(int id, String username, String password, String email, String first_name, String last_name, Boolean admin)
throws NullPointerException {
User userToUpdate = findOne(id);
if (userToUpdate == null)
throw new NullPointerException("Update user by id " + id + " has failed");
if (username != null)
userToUpdate.setUsername(username);
if (email != null)
userToUpdate.setEmail(email);
if (password != null)
userToUpdate.setPassword(password);
if (admin != null)
userToUpdate.setAdmin(admin);
if (first_name != null)
userToUpdate.setFirstName(first_name);
if (last_name != null)
userToUpdate.setLastName(last_name);
User updatedUser = userRepo.save(userToUpdate);
if (updatedUser == null)
throw new NullPointerException("Save updated user by id " + id + " has failed");
return updatedUser;
}
@Override
@Transactional(propagation = Propagation.REQUIRED, readOnly = false)
public void delete(int id) throws IllegalArgumentException {
try {
userRepo.delete(id);
}
catch(IllegalArgumentException e) {
throw new IllegalArgumentException("Save updated user by id " + id + " has failed", e);
}
}
}
package fr.umlv.orthopro.service;
import java.util.List;
import org.springframework.data.jpa.repository.JpaRepository;
import fr.umlv.orthopro.db.User;
public interface UserServiceIT extends JpaRepository<User, Integer>{
List<User> findAll();
User findById(int id);
User create(String username, String password, String email, String first_name, String last_name, boolean admin)
throws NullPointerException, IllegalArgumentException;
User update(int id, String username, String password, String email, String first_name, String last_name, Boolean admin)
throws NullPointerException ;
void delete(int id) throws IllegalArgumentException;
}
我的日志
[INFO] Scanning for projects...
[WARNING]
[WARNING] Some problems were encountered while building the effective model for fr.umlv.orthopro:OrthoPro_brain:jar:1.0-SNAPSHOT
[WARNING] 'dependencies.dependency.(groupId:artifactId:type:classifier)' must be unique: org.hibernate:hibernate-c3p0:jar -> duplicate declaration of version 5.2.12.Final @ line 138, column 19
[WARNING]
[WARNING] It is highly recommended to fix these problems because they threaten the stability of your build.
[WARNING]
[WARNING] For this reason, future Maven versions might no longer support building such malformed projects.
[WARNING]
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] Building OrthoPro_brain 1.0-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO]
[INFO] >>> spring-boot-maven-plugin:1.5.9.RELEASE:run (default-cli) > test-compile @ OrthoPro_brain >>>
[INFO]
[INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ OrthoPro_brain ---
[INFO] Using 'UTF-8' encoding to copy filtered resources.
[INFO] Copying 0 resource
[INFO] Copying 1 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.7.0:compile (default-compile) @ OrthoPro_brain ---
[INFO] Nothing to compile - all classes are up to date
[INFO]
[INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ OrthoPro_brain ---
[INFO] Using 'UTF-8' encoding to copy filtered resources.
[INFO] skip non existing resourceDirectory /Users/Unconnu/Desktop/orthopro/OrthoPro_brain/src/test/resources
[INFO]
[INFO] --- maven-compiler-plugin:3.7.0:testCompile (default-testCompile) @ OrthoPro_brain ---
[INFO] Nothing to compile - all classes are up to date
[INFO]
[INFO] <<< spring-boot-maven-plugin:1.5.9.RELEASE:run (default-cli) < test-compile @ OrthoPro_brain <<<
[INFO]
[INFO]
[INFO] --- spring-boot-maven-plugin:1.5.9.RELEASE:run (default-cli) @ OrthoPro_brain ---
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v1.5.9.RELEASE)
2017-12-30 19:51:29.818 INFO 13291 --- [ main] fr.umlv.orthopro.OrthoproApp : Starting OrthoproApp on MacBook-Pro-de-Unconnu.local with PID 13291 (/Users/Unconnu/Desktop/orthopro/OrthoPro_brain/target/classes started by Unconnu in /Users/Unconnu/Desktop/orthopro/OrthoPro_brain)
2017-12-30 19:51:29.821 INFO 13291 --- [ main] fr.umlv.orthopro.OrthoproApp : No active profile set, falling back to default profiles: default
2017-12-30 19:51:29.944 INFO 13291 --- [ main] ationConfigEmbeddedWebApplicationContext : Refreshing org.springframework.boot.context.embedded.AnnotationConfigEmbeddedWebApplicationContext@17fd8a8c: startup date [Sat Dec 30 19:51:29 CET 2017]; root of context hierarchy
2017-12-30 19:51:35.325 INFO 13291 --- [ main] org.xnio : XNIO version 3.3.8.Final
2017-12-30 19:51:35.359 INFO 13291 --- [ main] org.xnio.nio : XNIO NIO Implementation Version 3.3.8.Final
2017-12-30 19:51:35.448 WARN 13291 --- [ main] io.undertow.websockets.jsr : UT026009: XNIO worker was not set on WebSocketDeploymentInfo, the default worker will be used
2017-12-30 19:51:35.449 WARN 13291 --- [ main] io.undertow.websockets.jsr : UT026010: Buffer pool was not set on WebSocketDeploymentInfo, the default pool will be used
2017-12-30 19:51:35.532 INFO 13291 --- [ main] io.undertow.servlet : Initializing Spring embedded WebApplicationContext
2017-12-30 19:51:35.533 INFO 13291 --- [ main] o.s.web.context.ContextLoader : Root WebApplicationContext: initialization completed in 5596 ms
2017-12-30 19:51:35.813 INFO 13291 --- [ main] o.s.b.w.servlet.ServletRegistrationBean : Mapping servlet: 'dispatcherServlet' to [/]
2017-12-30 19:51:35.883 INFO 13291 --- [ main] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'characterEncodingFilter' to: [/*]
2017-12-30 19:51:35.884 INFO 13291 --- [ main] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'hiddenHttpMethodFilter' to: [/*]
2017-12-30 19:51:35.884 INFO 13291 --- [ main] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'httpPutFormContentFilter' to: [/*]
2017-12-30 19:51:35.885 INFO 13291 --- [ main] o.s.b.w.servlet.FilterRegistrationBean : Mapping filter: 'requestContextFilter' to: [/*]
2017-12-30 19:51:37.559 INFO 13291 --- [ main] j.LocalContainerEntityManagerFactoryBean : Building JPA container EntityManagerFactory for persistence unit 'default'
2017-12-30 19:51:37.649 INFO 13291 --- [ main] o.hibernate.jpa.internal.util.LogHelper : HHH000204: Processing PersistenceUnitInfo [
name: default
...]
2017-12-30 19:51:37.989 INFO 13291 --- [ main] org.hibernate.Version : HHH000412: Hibernate Core {5.2.12.Final}
2017-12-30 19:51:37.991 INFO 13291 --- [ main] org.hibernate.cfg.Environment : HHH000206: hibernate.properties not found
2017-12-30 19:51:38.127 INFO 13291 --- [ main] o.hibernate.annotations.common.Version : HCANN000001: Hibernate Commons Annotations {5.0.1.Final}
2017-12-30 19:51:38.513 INFO 13291 --- [ main] org.hibernate.dialect.Dialect : HHH000400: Using dialect: org.hibernate.dialect.H2Dialect
2017-12-30 19:51:38.691 INFO 13291 --- [ main] o.h.e.j.e.i.LobCreatorBuilderImpl : HHH000423: Disabling contextual LOB creation as JDBC driver reported JDBC version [3] less than 4
2017-12-30 19:51:41.379 INFO 13291 --- [ main] o.h.t.schema.internal.SchemaCreatorImpl : HHH000476: Executing import script 'org.hibernate.tool.schema.internal.exec.ScriptSourceInputNonExistentImpl@23fb847f'
2017-12-30 19:51:41.410 INFO 13291 --- [ main] j.LocalContainerEntityManagerFactoryBean : Initialized JPA EntityManagerFactory for persistence unit 'default'
2017-12-30 19:51:41.508 WARN 13291 --- [ main] ationConfigEmbeddedWebApplicationContext : Exception encountered during context initialization - cancelling refresh attempt: org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'userController': Unsatisfied dependency expressed through field 'userService'; nested exception is org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type 'fr.umlv.orthopro.service.UserServiceIT' available: expected at least 1 bean which qualifies as autowire candidate. Dependency annotations: {@org.springframework.beans.factory.annotation.Autowired(required=true)}
2017-12-30 19:51:41.508 INFO 13291 --- [ main] j.LocalContainerEntityManagerFactoryBean : Closing JPA EntityManagerFactory for persistence unit 'default'
2017-12-30 19:51:41.509 INFO 13291 --- [ main] .SchemaDropperImpl$DelayedDropActionImpl : HHH000477: Starting delayed drop of schema as part of SessionFactory shut-down'
2017-12-30 19:51:41.572 INFO 13291 --- [ main] utoConfigurationReportLoggingInitializer :
Error starting ApplicationContext. To display the auto-configuration report re-run your application with 'debug' enabled.
2017-12-30 19:51:42.193 ERROR 13291 --- [ main] o.s.b.d.LoggingFailureAnalysisReporter :
***************************
APPLICATION FAILED TO START
***************************
Description:
Field userService in fr.umlv.orthopro.controller.UserController required a bean of type 'fr.umlv.orthopro.service.UserServiceIT' that could not be found.
Action:
Consider defining a bean of type 'fr.umlv.orthopro.service.UserServiceIT' in your configuration.
[WARNING]
java.lang.reflect.InvocationTargetException
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:564)
at org.springframework.boot.maven.AbstractRunMojo$LaunchRunner.run(AbstractRunMojo.java:527)
at java.base/java.lang.Thread.run(Thread.java:844)
Caused by: org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'userController': Unsatisfied dependency expressed through field 'userService'; nested exception is org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type 'fr.umlv.orthopro.service.UserServiceIT' available: expected at least 1 bean which qualifies as autowire candidate. Dependency annotations: {@org.springframework.beans.factory.annotation.Autowired(required=true)}
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor$AutowiredFieldElement.inject(AutowiredAnnotationBeanPostProcessor.java:588)
at org.springframework.beans.factory.annotation.InjectionMetadata.inject(InjectionMetadata.java:88)
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor.postProcessPropertyValues(AutowiredAnnotationBeanPostProcessor.java:366)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.populateBean(AbstractAutowireCapableBeanFactory.java:1264)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean(AbstractAutowireCapableBeanFactory.java:553)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean(AbstractAutowireCapableBeanFactory.java:483)
at org.springframework.beans.factory.support.AbstractBeanFactory$1.getObject(AbstractBeanFactory.java:306)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton(DefaultSingletonBeanRegistry.java:230)
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:302)
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:197)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.preInstantiateSingletons(DefaultListableBeanFactory.java:761)
at org.springframework.context.support.AbstractApplicationContext.finishBeanFactoryInitialization(AbstractApplicationContext.java:867)
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:543)
at org.springframework.boot.context.embedded.EmbeddedWebApplicationContext.refresh(EmbeddedWebApplicationContext.java:122)
at org.springframework.boot.SpringApplication.refresh(SpringApplication.java:693)
at org.springframework.boot.SpringApplication.refreshContext(SpringApplication.java:360)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:303)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:1118)
at org.springframework.boot.SpringApplication.run(SpringApplication.java:1107)
at fr.umlv.orthopro.OrthoproApp.main(OrthoproApp.java:15)
... 6 more
Caused by: org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type 'fr.umlv.orthopro.service.UserServiceIT' available: expected at least 1 bean which qualifies as autowire candidate. Dependency annotations: {@org.springframework.beans.factory.annotation.Autowired(required=true)}
at org.springframework.beans.factory.support.DefaultListableBeanFactory.raiseNoMatchingBeanFound(DefaultListableBeanFactory.java:1493)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.doResolveDependency(DefaultListableBeanFactory.java:1104)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.resolveDependency(DefaultListableBeanFactory.java:1066)
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor$AutowiredFieldElement.inject(AutowiredAnnotationBeanPostProcessor.java:585)
... 25 more
[INFO] ------------------------------------------------------------------------
[INFO] BUILD FAILURE
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 24.029 s
[INFO] Finished at: 2017-12-30T19:51:42+01:00
[INFO] Final Memory: 34M/112M
[INFO] ------------------------------------------------------------------------
[ERROR] Failed to execute goal org.springframework.boot:spring-boot-maven-plugin:1.5.9.RELEASE:run (default-cli) on project OrthoPro_brain: An exception occurred while running. null: InvocationTargetException: Error creating bean with name 'userController': Unsatisfied dependency expressed through field 'userService'; nested exception is org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type 'fr.umlv.orthopro.service.UserServiceIT' available: expected at least 1 bean which qualifies as autowire candidate. Dependency annotations: {@org.springframework.beans.factory.annotation.Autowired(required=true)} -> [Help 1]
[ERROR]
[ERROR] To see the full stack trace of the errors, re-run Maven with the -e switch.
[ERROR] Re-run Maven using the -X switch to enable full debug logging.
[ERROR]
[ERROR] For more information about the errors and possible solutions, please read the following articles:
[ERROR] [Help 1] http://cwiki.apache.org/confluence/display/MAVEN/MojoExecutionException
謝謝,迪維亞
Remove 在UserService
類中implements UserServiceIt
,因為@Service
不是存儲庫。 我建議也改變名稱UserServiceIT
到UserRepository
並添加@Repository
注解。
嘗試添加此注釋:
@SpringBootApplication(com.package.pathTotheBean/*)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.