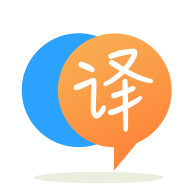
[英]Swift: How can I pass data from child viewcontroller prepareForSegue to change the TextLabel of a cell of parent viewcontroller tableview?
[英]How i can fill two different tableview in one viewcontroller in swift 3
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
if tableView == table1{
let cell = tableView.dequeueReusableCell(withIdentifier: "cell") as! acntTableViewCell
cell.account.text = account[indexPath.row].email
return cell
}
else if tableView == table2 {
let cell2 = tableView.dequeueReusableCell(withIdentifier: "cell2")
as! popTableViewCell
cell2.pop.text = pop[indexPath.row].answer
return cell2
}
}//its give me error here Missing return in function
我將在一個視圖控制器中填充兩個不同的表。 當我返回單元格時,它給我錯誤我做錯的函數中缺少返回值,任何人都可以告訴我這段代碼有什么問題
首先,您應該使用===
(引用)而不是==
來比較表。
這是斷言失敗是告訴編譯器不存在其他函數方式的好方法的情況之一,例如:
if tableView === table1 {
return ...
} else if tableView === table2 {
return ...
} else {
fatalError("Invalid table")
}
您還可以使用switch
:
switch tableView {
case table1:
return ...
case table2:
return ...
default:
fatalError("Invalid table")
}
兩個答案都是正確的,但我相信最好的方法是將每個表視圖分開以擁有自己的數據源對象,而不是視圖控制器。 放置多個 tableview 數據源協議會增加大量不必要的代碼,如果將它們重構為單獨的對象,則可以幫助避免 Massive View Controller。
class FirstTableViewDataSource : NSObject, UITableViewDataSource {
var accounts: [ObjectTypeHere]
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return accounts.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell") as! AcntTableViewCell
cell.account.text = accounts[indexPath.row].email
return cell
}
}
class SecondTableViewDataSource : NSObject, UITableViewDataSource {
var pops: [ObjectTypeHere]
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return pops.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell") as! PopTableViewCell
cell.account.text = pops[indexPath.row].answer
return cell
}
}
從那里,只需更新 tableview 即可從這些對象中提取
override func viewDidLoad() {
super.viewDidLoad()
self.table1.dataSource = FirstTableViewDataSource()
self.table2.dataSource = SecondTableViewDataSource()
}
編譯器正在分析如果tableView
既不是table1
也不是table2
會發生什么。 如果發生這種情況,該函數將退出,沒有任何返回。
那是一個錯誤。
你的cellForRowAt
方法應該總是返回一個單元格,所以
試試這個方法
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
if tableView == table1{
let cell = tableView.dequeueReusableCell(withIdentifier: "cell") as! acntTableViewCell
cell.account.text = account[indexPath.row].email
return cell
}
//if tableView is not table1 then
let cell2 = tableView.dequeueReusableCell(withIdentifier: "cell2")
as! popTableViewCell
cell2.pop.text = pop[indexPath.row].answer
return cell2
}
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var table1: UITableView!
@IBOutlet weak var table2: UITableView!
let firstClassRef = FirstTableView()
let secondClassRef = SecondTableView()
override func viewDidLoad() {
super.viewDidLoad()
firstClassRef.array1 = ["1","2","3"]
secondClassRef.array2 = ["1","2","3","1","2","3"]
self.table1.dataSource = firstClassRef
self.table2.dataSource = secondClassRef
self.table1.delegate = firstClassRef
self.table2.delegate = secondClassRef
self.table1.reloadData()
self.table2.reloadData()
}
}
class FirstTableView: NSObject, UITableViewDataSource, UITableViewDelegate {
var array1 = Array<Any>()
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return array1.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
{
let cell = tableView.dequeueReusableCell(withIdentifier: "cell1", for: indexPath) as UITableViewCell
cell.textLabel?.text = array1[indexPath.row] as? String
cell.backgroundColor = UIColor.yellow
return cell
}
}
class SecondTableView: NSObject, UITableViewDataSource, UITableViewDelegate {
var array2 = Array<Any>()
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return array2.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
{
let cell = tableView.dequeueReusableCell(withIdentifier: "cell2", for: indexPath) as UITableViewCell
cell.textLabel?.text = array2[indexPath.row] as? String
cell.backgroundColor = UIColor.yellow
return cell
}
}
使用 Switch 語句
import UIKit
class ViewController: UIViewController , UITableViewDelegate , UITableViewDataSource {
@IBOutlet weak var topTableView: UITableView!
@IBOutlet weak var downTableview: UITableView!
var topData : [String] = []
var downData = [String]()
override func viewDidLoad() {
super.viewDidLoad()
topTableView.delegate = self
downTableview.delegate = self
topTableView.dataSource = self
downTableview.dataSource = self
for index in 0...20 {
topData.append("Top Table Row \(index)")
}
for index in 10...45 {
downData.append("Down Table Row \(index)")
}
}
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
var numberOfRow = 1
switch tableView {
case topTableView:
numberOfRow = topData.count
case downTableview:
numberOfRow = downData.count
default:
print("Some things Wrong!!")
}
return numberOfRow
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = UITableViewCell()
switch tableView {
case topTableView:
cell = tableView.dequeueReusableCell(withIdentifier: "topCell", for: indexPath)
cell.textLabel?.text = topData[indexPath.row]
cell.backgroundColor = UIColor.green
case downTableview:
cell = tableView.dequeueReusableCell(withIdentifier: "downCell", for: indexPath)
cell.textLabel?.text = downData[indexPath.row]
cell.backgroundColor = UIColor.yellow
default:
print("Some things Wrong!!")
}
return cell
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.