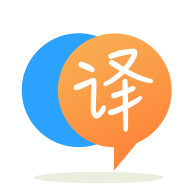
[英]How to check the internet Connection in iPhone without Reachability classes?
[英]iOS: How to test Internet connection in the most easy way, without freezing the app (without Reachability)?
在我的代碼中,我曾經使用三種方式來檢查Internet,但它們有限制:
1 /可達性方法:
- (BOOL)isInternetOk
{
Reachability *curReach = [Reachability reachabilityWithHostName:@"apple.com"];
NetworkStatus netStatus = [curReach currentReachabilityStatus];
if (netStatus != NotReachable) //if internet connexion ok
{
return YES;
}
else
{
return NO;
}
}
限制:它適用於大多數情況,但我的問題是,如果我連接天線Wi-Fi沒有沒有互聯網,它說連接是好的,但事實並非如此。 這不是一個好的解決方案,我需要檢查Reachability上似乎沒有的狀態代碼。
2 / sendSynchronousRequest:
- (BOOL)isInternetOk2
{
NSMutableURLRequest* request = [[NSMutableURLRequest alloc] init];
NSURL* URL = [NSURL URLWithString:@"https://www.google.com"];
NSError *error = nil;
[request setURL:URL];
[request setCachePolicy:NSURLRequestReloadIgnoringLocalCacheData];
[request setTimeoutInterval:15];
NSData* response2 = [NSURLConnection sendSynchronousRequest:request returningResponse:nil error:&error];
if (error)
{
return NO;
}
else
{
return YES;
}
}
限制:它也有效,但我的問題是,如果有一個超時,這可能發生在每個時刻,它會在很長一段時間內凍結應用程序。 如果我把它放在一個線程中,似乎當我在dispatch_async中執行請求時,響應沒有考慮在內。
3 / sendAsynchronousRequest:
NSOperationQueue *myQueue = [[NSOperationQueue alloc] init];
NSMutableURLRequest *request = [[NSMutableURLRequest alloc] initWithURL:[NSURL URLWithString:@"https://www.google.com"]];
request.timeoutInterval = 10;
[NSURLConnection sendAsynchronousRequest:request queue:myQueue completionHandler:^(NSURLResponse *response, NSData *data, NSError *error)
{
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"response status code: %ld, error status : %@", (long)[httpResponse statusCode], error.description);
if ((long)[httpResponse statusCode] >= 200 && (long)[httpResponse statusCode]< 400)
{
// do stuff
NSLog(@"Connected!");
}
else
{
NSLog(@"Not connected!");
}
}];
限制:我認為這是更好的方法,但我的問題是我必須在我的代碼中寫下每個位置,這將是一個污點。 我想知道是否有一個不太重的方法來做到這一點。
你怎么看待這件事? 有沒有其他方法更容易檢查互聯網是否正常工作而不凍結應用程序?
提前致謝。
Nayem是對的 - 您應該在類方法中包裝第三個選項(異步網絡檢查),如下所示:
+ (void)checkInternetConnectivityWithSuccessCompletion:(void (^)(void))completion {
NSOperationQueue *myQueue = [[NSOperationQueue alloc] init];
NSMutableURLRequest *request = [[NSMutableURLRequest alloc] initWithURL:[NSURL URLWithString:@"https://www.google.com"]];
request.timeoutInterval = 10;
[NSURLConnection sendAsynchronousRequest:request queue:myQueue completionHandler:^(NSURLResponse *response, NSData *data, NSError *error)
{
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"response status code: %ld, error status : %@", (long)[httpResponse statusCode], error.description);
if ((long)[httpResponse statusCode] >= 200 && (long)[httpResponse statusCode]< 400)
{
// do stuff
NSLog(@"Connected!");
completion();
}
else
{
NSLog(@"Not connected!");
}
}];
}
然后調用這樣的方法:
[YourClass checkInternetConnectivityWithSuccessCompletion:^{
// your internet is working - add code here
}];
另一種選擇
#import <SystemConfiguration/SCNetworkReachability.h>
.....
+(bool)isNetworkAvailable {
SCNetworkReachabilityFlags flags;
SCNetworkReachabilityRef address;
address = SCNetworkReachabilityCreateWithName(NULL, "www.apple.com");
Boolean success = SCNetworkReachabilityGetFlags(address, &flags);
CFRelease(address);
bool canReach = success
&& !(flags & kSCNetworkReachabilityFlagsConnectionRequired)
&& (flags & kSCNetworkReachabilityFlagsReachable);
return canReach;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.