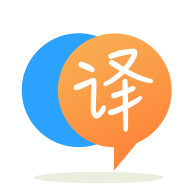
[英]Uncaught TypeError: Cannot read property 'state' of undefined - React.js
[英]React.js cannot read my state
我無法解決的問題是狀態...會嘗試標記點,通常是關於pause(),resume()(如果您有其他建議的話)。
提前致謝!
enter code here
import ReactDOM from 'react-dom';
import React from "react";
import {Router, Route, Link, IndexLink, IndexRoute, hashHistory} from 'react-router';
let BODY = 1, FOOD = 2;
let KEYS = {left: 37, up: 38, right: 39, down: 40};
let DIRS = {37: true, 38: true, 39: true, 40: true};
export class SnakeGames extends React.Component {
constructor(props) {
super(props);
const start = this.props.startIndex || 21;
const snake = [start], board = [];
board[start] = BODY;
this.state = {
snake: snake,
board: board,
growth: 0,
paused: true,
gameOver: false,
direction: KEYS.right
}
}
componentDidMount() {
this.resume();
}
reset(something) {
console.log(something);
this.setState(this.getInitialState());
this.resume();
}
pause() {
if ***(this.state.gameOver || this.state.paused)*** {
return;
}
this.setState({paused: true});
}
resume() {
if ***(this.state.gameOver || !this.state.paused)*** {
return;
}
this.setState({paused: false});
this.refs.board.focus();
this.tick();
}
tick() {
if (this.state.paused) {
return;
}
const snake = this.state.snake;
const board = this.state.board;
const growth = this.state.growth;
const direction = this.state.direction;
const numRows = this.props.numRows || 20;
const numCols = this.props.numCols || 20;
const head = getNextIndex(snake[0], direction, numRows, numCols);
if (snake.indexOf(head) !== -1) {
this.setState({gameOver: true});
return;
}
const needsFood = board[head] === FOOD || snake.length === 1;
if (needsFood) {
let ii, numCells = numRows * numCols;
do {
ii = Math.floor(Math.random() * numCells);
} while (board[ii]);
board[ii] = FOOD;
this.state.growth += 2;
} else if (growth) {
this.state.growth -= 1;
} else {
board[snake.pop()] = null;
}
snake.unshift(head);
board[head] = BODY;
if (this.nextDirection) {
this.state.direction = this.nextDirection;
this.nextDirection = null;
}
this.setState({
snake: snake,
board: board,
growth: growth,
direction: direction
});
setTimeout(this.tick, 100);
}
handleKey(event) {
var direction = event.nativeEvent.keyCode;
var difference = Math.abs(this.state.direction - direction);
// if key is invalid, or the same, or in the opposite direction
if (DIRS[direction] && difference !== 0 && difference !== 2) {
this.nextDirection = direction;
}
}
render() {
let cells = [];
const numRows = this.props.numRows || 20;
const numCols = this.props.numCols || 20;
const cellSize = this.props.cellSize || 30;
for (let row = 0; row < numRows; row++) {
for (let col = 0; col < numCols; col++) {
let code = this.state.board[numCols * row + col];
let type = code === BODY ? 'body' : code === FOOD ? 'food' : 'null';
cells.push(<div className={type + '-cell'}/>);
}
}
return (
<div className="snake-game">
<h1 className="snake-score">Score: {this.state.snake.length}</h1>
<div
ref="board"
className={'snake-board' + (this.state.gameOver ? ' game-over' : '')}
tabIndex={0}
onBlur={this.pause}
onFocus={this.resume}
onKeyDown={this.handleKey}
style={{width: numCols * cellSize, height: numRows * cellSize}}>
{cells}
</div>
<div className="snake-controls">
{this.state.paused ? <button onClick={this.resume}>Resume</button> : null}
{this.state.gameOver ? <button onClick={this.reset}>New Game</button> : null}
</div>
<button className="btn"><Link to="/snakemenu">RETURN</Link></button>
<button className="btn"><Link to="/snakegameover">GAME OVER</Link></button>
</div>
);
}
}
function getNextIndex(head, direction, numRows, numCols) {
let x = head % numCols;
let y = Math.floor(head / numCols);
switch (direction) {
case KEYS.up:
y = y <= 0 ? numRows - 1 : y - 1;
break;
case KEYS.down:
y = y >= numRows - 1 ? 0 : y + 1;
break;
case KEYS.left:
x = x <= 0 ? numCols - 1 : x - 1;
break;
case KEYS.right:
x = x >= numCols - 1 ? 0 : x + 1;
break;
default:
return;
}
// translate new x/y coords back into array index
return (numCols * y) + x;
}
您需要將resume和pause方法綁定到“ this”對象,以便引用該對象上的所有字段。 代替:
<button onClick={this.resume}>Resume</button>
您將使用:
<button onClick={this.resume.bind(this)}>Resume</button>
其他函數綁定也是如此:
<div
ref="board"
className={'snake-board' + (this.state.gameOver ? ' game-over' : '')}
tabIndex={0}
onBlur={this.pause.bind(this)}
onFocus={this.resume.bind(this)}
onKeyDown={this.handleKey.bind(this)}
style={{width: numCols * cellSize, height: numRows * cellSize}}>
{cells}
</div>
正如John Weisz在評論中提到的那樣,如果在render方法中進行所有的綁定調用,則往往會降低性能。 最好將這些調用移至構造函數:
constructor(props) {
super(props);
const start = this.props.startIndex || 21;
const snake = [start], board = [];
board[start] = BODY;
this.state = {
snake: snake,
board: board,
growth: 0,
paused: true,
gameOver: false,
direction: KEYS.right
}
this.pause = this.pause.bind(this);
this.resume = this.resume.bind(this);
this.handleKey = this.handleKey.bind(this);
}
然后,您無需觸摸渲染中的任何現有代碼即可添加綁定。
您可能要考慮的另一件事是利用ES6中的箭頭功能。 所以這:
resume() {
if ***(this.state.gameOver || !this.state.paused)*** {
return;
}
this.setState({paused: false});
this.refs.board.focus();
this.tick();
}
...變為:
resume = () => {
if ***(this.state.gameOver || !this.state.paused)*** {
return;
}
this.setState({paused: false});
this.refs.board.focus();
this.tick();
}
這樣可以避免為每個函數將.bind(this)
添加到構造函數中,因此有點DRYer。
請記住,您不能像.bind(this)
那樣更改箭頭函數的上下文。 因此,如果需要更改函數上下文,則必須堅持使用.bind(this)
或(甚至更好)使用閉包。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.