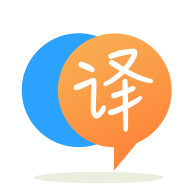
[英]How to read a specific row and column from a text file and base decision on numeric value with python?
[英]Read specific column value from a text file using Python
我有一個文本文件,如下所示:
1 1 2 1 1e8
2 1 2 3 1e5
3 2 3 2 2000
4 2 5 6 1000
5 2 4 3 1e4
6 3 6 4 5000
7 3 5 2 2000
8 3 2 3 5000
9 3 4 5 1e9
10 3 2 3 1e6
在我的文字中(比這個示例大得多),第二列是層數,最后一列是該層中的能量,我想提取每一層中的能量,例如第二列中的數字2,我需要從上一列中獲得與該圖層有關的能量,並且我想將文本文件的這一部分分開
3 2 3 2 2000
4 2 5 6 1000
5 2 4 3 1e4
如何在python中完成這項工作?
您可以像這樣從文本文件中獲取層次和能量
layers = []
energies = []
with open(file) as f:
for line in f:
linesplit = line.strip().split() # splits by whitespace
layers.append(int(linesplit[1])) # 2nd index
energies.append(float(linesplit[-1])) # last index
編輯:如果您有標題行(例如,第1行),則可以使用以下命令跳過它:
header_line = 1 # or whatever it is
with open(file) as f:
for line_number, line in enumerate(f, 1):
if line_number <= header_line:
continue
linesplit = line.strip().split()
layers.append(int(linesplit[1]))
energies.append(float(linesplit[-1]))
我不知道您的文件是什么樣子,因為您還沒有發布完整的內容,因此在看不到整個內容的情況下(即在pastebin.com上),我將為您提供更多幫助。
最后嘗試:
layers = []
energies = []
with open(file) as f:
for lineno, line in enumerate(f, 1):
linesplit = line.strip().split() # splits by whitespace
if not linesplit: # empty
continue
try:
layers.append(int(linesplit[1])) # 2nd inde
except (TypeError, IndexError):
print("Skipping line {}: {!r}".format(lineno, line))
continue
try:
energies.append(float(linesplit[-1])) # last index
except TypeError:
layers.pop()
print("Skipping and reverting line {}: {!r}".format(lineno, line)):
為什么不首先創建CSV文件? 因此,您可以使用“;”分隔每個值/列。 在每一行中,您都會在該CSV文件中打印新行。
如果是CSV,則只需使用“拆分”
line.split(';')[column you want]
例:
line = '1;1;2;1;1e8'
print(line.split(';')[5])
>> 1e8
編輯:從文件中讀取所有行並將其放入數組中。 注意:此代碼未經測試,並且編寫迅速。 它應該顯示您必須走的方向。
elements = []
f.open('filename')
lines = f.readlines()
for x, line in lines:
elemenets.append([])
for y in range(0,5):
elements[x].append(line.split()[y])
如果您已經知道需要什么行,則可以使用:
f.open('filename')
lines = f.readlines()
print(lines[index_of_line].split()[index_of_item])
不帶任何參數的split方法將在空白處分割字符串。 a.txt-是數據文件名。
#!/usr/bin/env python
with open ('a.txt') as f:
for line in f:
line.strip() # Removes \n and spaces on the end
var1, var2, var3, var4, var5 = line.split()
print(var1, var2, var3, var4, var5)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.