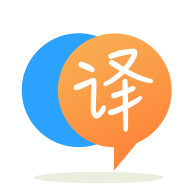
[英]Storing and reusing results of functions in Insert/Select statement
[英]Storing the result of a SELECT query in an integer and reusing it in an INSERT statement
我有一個查詢,用於將部門插入department
表中。 但是, Address
表有一個名為Address_ID
的外鍵。 每當我想插入一個新部門時,我還想檢查該地址是否存在,如果不存在,我想創建一個新部門。 如果確實存在,我想找到正確的ID並將其存儲在變量中以備后用。 該變量已在代碼開頭聲明為BIGINT
。
-- If the address already exists, we will select its ID and store it in a variable.
BEGIN
SET @Address_ID =
(
SELECT TOP 1 [Address_ID]
FROM [Address]
WHERE
[Building] = @Building_Param
AND [Factory] = @Factory_Param
)
END
上面的代碼是較大語句的一部分。 您可以在下面找到完整的代碼。
此段代碼失敗,並顯示以下錯誤消息:
死@ Address_ID-Tabellenvariable muss deklariert werden。
翻譯:
@Address_ID表變量必須聲明。
這是完整的代碼:
/**
* Inserts a new entry into the department table.
*
* @author Rubbel Die Katz
*/
DECLARE @The_Date DATETIME2
SET @The_Date = GETDATE()
DECLARE @Department_Name_Param NVARCHAR(MAX)
SET @Department_Name_Param = ?
DECLARE @Department_Description_Param NVARCHAR(MAX)
SET @Department_Description_Param = ?
DECLARE @Factory_Param NVARCHAR(MAX)
SET @Factory_Param = ?
DECLARE @Building_Param NVARCHAR(MAX)
SET @Building_Param = ?
DECLARE @Address_ID BIGINT
SET @Address_ID = 0
-- If the address does not exist, we will need to create it automagically.
IF NOT EXISTS (
SELECT *
FROM [Address]
WHERE
[Building] = @Building_Param
AND [Factory] = @Factory_Param
)
BEGIN
INSERT INTO [Department] (
[Department_Name],
[Department_Description],
[Time_Created],
[Time_Modified]
)
OUTPUT INSERTED.[Address_ID] INTO @Address_ID
VALUES (
@Department_Name_Param,
@Department_Description_Param,
@The_Date,
@The_Date
)
END
ELSE
-- If the address already exists, we will select its ID and store it in a variable.
BEGIN
SET @Address_ID =
(
SELECT TOP 1 [Address_ID]
FROM [Address]
WHERE
[Building] = @Building_Param
AND [Factory] = @Factory_Param
)
END
-- If the department does not exist, we will create it using the Address_ID provided by our previous operations.
IF NOT EXISTS (SELECT * FROM [Department] WHERE [Department_Name] = @Department_Name_Param)
BEGIN
INSERT INTO [Department] (
[Department_Name],
[Department_Description],
[Address_ID],
[Time_Created],
[Time_Modified]
)
VALUES (
@Department_Name_Param,
@Department_Description_Param,
@Address_ID,
@The_Date,
@The_Date
)
END
我正在使用的表都是空的。
如何從表中獲取BIGINT
作為BIGINT
而不是表變量?
錯誤非常明顯,在這里,您尚未聲明@Address_ID
。 在批處理開始時,您需要對其進行DECLARE
:
DECLARE @Address_ID TABLE (AddressID BIGINT);
然后,您可以使用以下方法將該值放入數據類型BIGINT
的變量中:
DECLARE @Address_ID_BI BIGINT;
SELECT @Address_ID_BI = AddressID
FROM @Address_ID;
該ASSUMES您僅插入一個值。
出現錯誤的原因是您沒有插入地址表並獲得該標識值。
如果您從以下位置更改代碼段:
INSERT INTO [Department] (
[Department_Name],
[Department_Description],
[Time_Created],
[Time_Modified]
)
OUTPUT INSERTED.[Address_ID] INTO @Address_ID
VALUES (
@Department_Name_Param,
@Department_Description_Param,
@The_Date,
@The_Date
)
成為:
insert into [Address] ( Building, Factory ) values ( @Building_Param, @Factory_Param )
set @Address_ID = ( SELECT SCOPE_IDENTITY() )
然后,您將獲得插入的地址ID。
這是易於測試的完整代碼:
/**
* Inserts a new entry into the department table.
*
* @author Rubbel Die Katz
*/
DECLARE @The_Date DATETIME2
SET @The_Date = GETDATE()
DECLARE @Department_Name_Param NVARCHAR(MAX)
SET @Department_Name_Param = ?
DECLARE @Department_Description_Param NVARCHAR(MAX)
SET @Department_Description_Param = ?
DECLARE @Factory_Param NVARCHAR(MAX)
SET @Factory_Param = ?
DECLARE @Building_Param NVARCHAR(MAX)
SET @Building_Param = ?
DECLARE @Address_ID BIGINT
SET @Address_ID = 0
-- If the address does not exist, we will need to create it automagically.
IF NOT EXISTS (
SELECT *
FROM [Address]
WHERE
[Building] = @Building_Param
AND [Factory] = @Factory_Param
)
BEGIN
insert into [Address] ( Building, Factory ) values ( @Building_Param, @Factory_Param )
set @Address_ID = ( SELECT SCOPE_IDENTITY() )
END
ELSE
-- If the address already exists, we will select its ID and store it in a variable.
BEGIN
SET @Address_ID =
(
SELECT TOP 1 [Address_ID]
FROM [Address]
WHERE
[Building] = @Building_Param
AND [Factory] = @Factory_Param
)
END
-- If the department does not exist, we will create it using the Address_ID provided by our previous operations.
IF NOT EXISTS (SELECT * FROM [Department] WHERE [Department_Name] = @Department_Name_Param)
BEGIN
INSERT INTO [Department] (
[Department_Name],
[Department_Description],
[Address_ID],
[Time_Created],
[Time_Modified]
)
VALUES (
@Department_Name_Param,
@Department_Description_Param,
@Address_ID,
@The_Date,
@The_Date
)
END
我對您的腳本進行了一些小的更改。 嘗試這個:
DECLARE @The_Date DATETIME2
SET @The_Date = GETDATE()
DECLARE @Department_Name_Param NVARCHAR(MAX)
SET @Department_Name_Param = ?
DECLARE @Department_Description_Param NVARCHAR(MAX)
SET @Department_Description_Param = ?
DECLARE @Factory_Param NVARCHAR(MAX)
SET @Factory_Param = ?
DECLARE @Building_Param NVARCHAR(MAX)
SET @Building_Param = ?
DECLARE @Address_ID BIGINT
SET @Address_ID = 0
-- If the address does not exist, we will need to create it automagically.
IF NOT EXISTS(
SELECT 1
FROM [Address]
WHERE [Building] = @Building_Param
AND [Factory] = @Factory_Param
)
BEGIN
INSERT INTO [Address]
(
[Factory],
[Building],
[Time_Created],
[Time_Modified]
)
VALUES
(
@Factory_Param,
@Building_Param_Param,
@The_Date,
@The_Date
)
END
--Else Part is Not Neccessary
SELECT @Address_ID = [Address_ID]
--Top 1 is not needed since the variable can hold only 1 value it will take only the Top 1 by Default
FROM [Address]
WHERE
[Building] = @Building_Param
AND [Factory] = @Factory_Param;
-- If the department does not exist, we will create it using the Address_ID provided by our previous operations.
IF NOT EXISTS(SELECT 1
FROM [Department]
WHERE [Department_Name] = @Department_Name_Param)
BEGIN
INSERT INTO [Department]
(
[Department_Name],
[Department_Description],
[Address_ID],
[Time_Created],
[Time_Modified]
)
VALUES
(
@Department_Name_Param,
@Department_Description_Param,
@Address_ID,
@The_Date,
@The_Date
)
END
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.