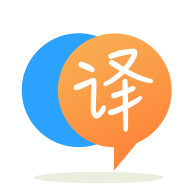
[英]how to apply mongo db update operators as $inc in spring boot
[英]How to update data in mongowith spring boot and mongo and react
我正在編寫具有MongoDB集成的Spring Boot應用程序。 我有幾個文件正在寫在這里。
ProductController.java
package com.spring.restapi.controllers;
import com.spring.restapi.models.product;
import com.spring.restapi.repositories.ProductRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ProductController {
@Autowired
ProductRepository productRepository;
@CrossOrigin(origins = "http://localhost:8080")
@RequestMapping(method = RequestMethod.GET, value ="/products")
public Iterable<product> product(){
return productRepository.findAll();
}
@CrossOrigin(origins = "http://localhost:8080")
@RequestMapping(method = RequestMethod.POST, value= "/products", consumes = MediaType.APPLICATION_JSON_VALUE)
public String save(@RequestBody product product)
{
System.out.print("The productName is" +product.getProdName());
productRepository.save(product);
return product.getId();
}
@CrossOrigin(origins = "http://localhost:8080")
@RequestMapping(method = RequestMethod.GET, value="/products/{id}")
public product show(@PathVariable String id){
return productRepository.findOne(id);
}
@CrossOrigin(origins = "http://localhost:8080")
@RequestMapping(method = RequestMethod.PUT, value="/products/{id}")
public product update(@PathVariable String id, @RequestBody product product){
product prod = productRepository.findOne(id);
if(product.getProdName()!= null)
prod.setProdName(product.getProdName());
if(product.getProdDesc()!= null)
prod.setProdDesc(product.getProdDesc());
if(product.getProdPrice()!=null)
prod.setProdPrice(product.getProdPrice());
if(product.getProdImage()!=null)
prod.setProdImage(product.getProdImage());
if(product.getProdManufacturer()!=null)
prod.setProdManufacturer(product.getProdManufacturer());
return prod;
}
@CrossOrigin(origins = "http://localhost:8080")
@RequestMapping(method = RequestMethod.DELETE, value="/products/{id}")
public String delete(@PathVariable String id)
{
product product = productRepository.findOne(id);
productRepository.delete(product);
return "product deleted";
}
}
我的模型文件為:
產品.java
package com.spring.restapi.models;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "products")
public class product {
@Id
String id;
String prodName;
String prodDesc;
Double prodPrice;
String prodImage;
String prodManufacturer;
public product() {
}
public product (String prodName, String prodDesc, Double prodPrice, String prodImage, String prodManufacturer)
{
this.prodName = prodName;
this.prodDesc = prodDesc;
this.prodPrice = prodPrice;
this.prodImage = prodImage;
this.prodManufacturer = prodManufacturer;
}
public String getId()
{
return id;
}
public void setId(String id){
this.id =id;
}
public String getProdName(){
return prodName;
}
public void setProdName(String prodName){
this.prodName = prodName;
}
public String getProdDesc(){
return prodDesc;
}
public void setProdDesc(String prodDesc){
this.prodDesc = prodDesc;
}
public Double getProdPrice(){
return prodPrice;
}
public void setProdPrice(Double prodPrice)
{
this.prodPrice = prodPrice;
}
public String getProdImage(){
return prodImage;
}
public void setProdImage(String prodImage){
this.prodImage = prodImage;
}
public String getProdManufacturer(){
return prodManufacturer;
}
public void setProdManufacturer(String prodManufacturer){
this.prodManufacturer = prodManufacturer;
}
}
最后一個產品存儲庫.java為
package com.spring.restapi.repositories;
import com.spring.restapi.models.product;
import org.springframework.data.repository.CrudRepository;
public interface ProductRepository extends CrudRepository<product, String> {
@Override
product findOne(String id);
@Override
void delete(product deleted);
}
現在,我嘗試使用前端代碼中的所有字段進行發布請求,如下所示:
import React, { Component } from 'react';
import jquery from 'jquery';
import $ from 'jquery';
import logo from './logo.svg';
import './App.css';
// import '../node_modules/jquery/jquery.min.js';
class App extends Component {
addProduct()
{
var productObject = {
"name": document.getElementById('productName').value,
"price": document.getElementById('productPrice').value,
"description":document.getElementById('productDescription').value,
"manufacturer":document.getElementById('productManufacturer').value
};
var dataPushed ={
productName: productObject.name,
productPrice:productObject.price,
productDescription:productObject.description,
productManufacturer:productObject.manufacturer
};
console.log("The Data is ",JSON.stringify(dataPushed));
var dataPushedForFinal = JSON.stringify(dataPushed);
//var dataForPost = JSON.stringify(productObject);
$.ajax({
type:"POST",
url:'http://localhost:8080/products',
data:dataPushedForFinal,
contentType:"application/json",
dataType:"json",
crossOrigin:true,
crossDomain:true,
});
//console.log("The value collected is",ProductName + "and " , ProductPrice+"and", ProductManufacturer);
}
render() {
return (
<div className="mainPageDiv">
<div className="App">
<header className="App-header">
<h1 className="App-title">Welcome to Stock Maintenance</h1>
</header>
</div>
<div className = "container-fluid">
<div className="row">
<div className ="col-md-6">
<div className="myFormDiv">
<div className="form_main">
<h4 className="heading"><strong>Product </strong> Details <span></span></h4>
<div className="form">
<input type="text" id="productName" required="" placeholder="Please Enter Product Name" name="name" className="txt"/>
<input type="text" id="productPrice"required="" placeholder="Please Enter Product Price" name="mob" className="txt"/>
<input type="text" id="productManufacturer"required="" placeholder="Please Enter Product Manufacturer" name="email" className="txt"/>
<textarea placeholder="Please Enter product description" id="productDescription" name="mess" type="text" className="txt_3"></textarea>
<center> <input type="submit" value="submit" name="submit" className="txt2" onClick ={this.addProduct}/> </center>
</div>
</div>
</div>
</div>
<div className = "col-md-6">
<div className = "myFormDiv">
<h4 className="heading"><strong>Product </strong> table <span></span></h4>
<div class="table-responsive col-md-12">
<table id="sort2" class="grid table table-bordered table-sortable">
<thead>
<tr>
<th>ID</th>
<th>Product Name</th>
<th>Product Price</th>
<th>Product Manufacturer</th>
<th>Product Description</th></tr>
</thead>
<tbody>
<tr>
<td>1</td>
<td><input type="text" value="name 1" class="form-control"/></td>
<td><input type="text" value="email 1" class="form-control"/></td>
<td><input type="text" value="email 1" class="form-control"/></td>
<td><input type="text" value="email 1" class="form-control"/></td>
<td><button class="btn btn-primary ">update</button> <button class="btn btn-danger">delete</button></td>
</tr>
<tr>
<td>2</td>
<td><input type="text" value="name 2" class="form-control"/></td>
<td><input type="text" value="email 2" class="form-control"/></td>
<td><input type="text" value="email 1" class="form-control"/></td>
<td><input type="text" value="email 1" class="form-control"/></td>
<td><button class="btn btn-primary ">update</button><button class="btn btn-danger">delete</button></td>
</tr>
<tr>
<td>3</td>
<td><input type="text" value="name 3" class="form-control"/></td>
<td><input type="text" value="email 3" class="form-control"/></td>
<td><input type="text" value="email 1" class="form-control"/></td>
<td><input type="text" value="email 1" class="form-control"/></td>
<td><button class="btn btn-primary ">update</button> <button class="btn btn-danger">delete</button></td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</div>
);
}
}
export default App;
但是數據並沒有被添加到mongodb中,它只是generatinf ID,但是當我嘗試與郵遞員添加它時,它就會被添加。
請有人能幫我在springboot和mongodb中是全新的。
您的REST服務需要一個可以模仿product
類的JSON對象
product
包括類型)相同(因此,您需要確保price是Number
而不是字符串) JSON.stringify
就像是
$.ajax({
type: 'POST',
...
data: JSON.stringify({
id: null,
prodName: document.getElementById('productName').value,
prodDesc: document.getElementById('productDescription').value,
prodPrice: Number(document.getElementById('productPrice').value),
prodImage: '...',
prodManufacturer: document.getElementById('productManufacturer').value
}),
...
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.