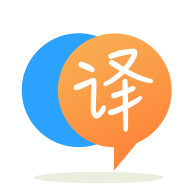
[英]unit testing FaceDetector Google mobile vision java.lang.UnsupportedOperationException
[英]Testing Google Cloud Vision with Java in Eclipse
我正在Eclipse中使用Java測試Google Cloud Vision。
我已經從https://cloud.google.com/vision/docs/reference/libraries#client-libraries-install-java復制了Java代碼
// Imports the Google Cloud client library
import com.google.cloud.vision.v1.AnnotateImageRequest;
import com.google.cloud.vision.v1.AnnotateImageResponse;
import com.google.cloud.vision.v1.BatchAnnotateImagesResponse;
import com.google.cloud.vision.v1.EntityAnnotation;
import com.google.cloud.vision.v1.Feature;
import com.google.cloud.vision.v1.Feature.Type;
import com.google.cloud.vision.v1.Image;
import com.google.cloud.vision.v1.ImageAnnotatorClient;
import com.google.protobuf.ByteString;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
public class QuickstartSample {
public static void main(String... args) throws Exception {
// Instantiates a client
try (ImageAnnotatorClient vision = ImageAnnotatorClient.create()) {
// The path to the image file to annotate
String fileName = "./resources/wakeupcat.jpg";
// Reads the image file into memory
Path path = Paths.get(fileName);
byte[] data = Files.readAllBytes(path);
ByteString imgBytes = ByteString.copyFrom(data);
// Builds the image annotation request
List<AnnotateImageRequest> requests = new ArrayList<>();
Image img = Image.newBuilder().setContent(imgBytes).build();
Feature feat = Feature.newBuilder().setType(Type.LABEL_DETECTION).build();
AnnotateImageRequest request = AnnotateImageRequest.newBuilder()
.addFeatures(feat)
.setImage(img)
.build();
requests.add(request);
// Performs label detection on the image file
BatchAnnotateImagesResponse response = vision.batchAnnotateImages(requests);
List<AnnotateImageResponse> responses = response.getResponsesList();
for (AnnotateImageResponse res : responses) {
if (res.hasError()) {
System.out.printf("Error: %s\n", res.getError().getMessage());
return;
}
for (EntityAnnotation annotation : res.getLabelAnnotationsList()) {
annotation.getAllFields().forEach((k, v) ->
System.out.printf("%s : %s\n", k, v.toString()));
}
}
}
}
}
從哪里可以下載.JAR,以便所有文件都能正確編譯?
Google自己說(請參閱上面的鏈接)
如果使用的是Maven,請將其添加到pom.xml文件中:
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-vision</artifactId>
<version>1.14.0</version> </dependency>
但是,我沒有使用Maven(他們的其他建議也不是Gradle或SBT)。
因此,我想在Eclipse中打開一個New Maven項目,然后將其自動下載所有JAR,然后將它們復制到我的項目中。
因此,我在Eclipse中執行了“新的Maven項目”,然后當它說“為工件輸入一個組ID”時,我在上面粘貼時從Google輸入了詳細信息,但沒有下載任何內容。
有什么想法可以獲取JAR,以便代碼可以編譯嗎?
Eclipse自動生成的pom.xml文件是
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-vision</artifactId>
<version>1.14.0</version>
<packaging>jar</packaging>
<name>google-cloud-vision</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
現在,有了Maven后,就可以添加依賴項了。
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<!-- THIS IS YOUR APP RELATED STUFF -->
<groupId>com.your.app.group.id</groupId> <!-- YOU SHOULD PROVIDE THIS -->
<artifactId>your-app-name</artifactId> <!-- YOU SHOULD PROVIDE THIS -->
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- THIS ARE YOUR APP dependencies -->
<!-- Google lib should be declared here -->
<dependencies>
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-vision</artifactId>
<version>1.14.0</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
如果此依賴關系在Maven Central中-Maven將下載它。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.