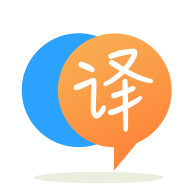
[英]Jackson/RestTemplate Springboot to deserialize an array of arrays JSON
[英]Spring RestTemplate Jackson Deserialize Array of Arrays
我正在使用 Spring RestTemplate 從 API 中提取數據,該 API 以以下格式返回未命名的數組數組:
[
[
1518230040,
8863.96,
8876.95,
8863.96,
8876.94,
1.81986441
],
[
1518229980,
8851.03,
8862.53,
8853.79,
8862.53,
2.95533163
],
[
1518229920,
8853.79,
8855.3,
8855.29,
8853.8,
3.0015851299999996
]
]
嵌套數組中的索引代表Candlestick的以下屬性:
0 => time
1 => low
2 => high
3 => open
4 => close
5 => volume
例如,在第一個嵌套數組中,燭台的時間為 1518230040,最低價為 8863.96,最高價為 8876.95,開盤價為 8863.96,收盤價為 8876.94,成交量為 1.81986441。
我想將這些嵌套的數組元素映射到 Candlestick 對象。 下面是我的燭台課。
import java.math.BigDecimal;
public class Candlestick {
private long time;
private BigDecimal low;
private BigDecimal high;
private BigDecimal open;
private BigDecimal close;
private BigDecimal volume;
public long getTime() {
return time;
}
public void setTime(long time) {
this.time = time;
}
public BigDecimal getLow() {
return low;
}
public void setLow(BigDecimal low) {
this.low = low;
}
public BigDecimal getHigh() {
return high;
}
public void setHigh(BigDecimal high) {
this.high = high;
}
public BigDecimal getOpen() {
return open;
}
public void setOpen(BigDecimal open) {
this.open = open;
}
public BigDecimal getClose() {
return close;
}
public void setClose(BigDecimal close) {
this.close = close;
}
public BigDecimal getVolume() {
return volume;
}
public void setVolume(BigDecimal volume) {
this.volume = volume;
}
@Override
public String toString() {
return "Candle [time=" + time + ", low=" + low + ", high=" + high + ", open=" + open + ", close=" + close
+ ", volume=" + volume + "]";
}
}
如何使用 Spring RestTemplate 和 Jackson 將嵌套數組映射到 Candlestick 對象?
您需要執行以下操作;
為 Candlestick pojo 配置編寫自定義解串器
public class CandlestickDeserializer extends StdDeserializer<Candlestick> { public CandlestickDeserializer() { this(null); } public CandlestickDeserializer(Class<?> vc) { super(vc); } @Override public Item deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException, JsonProcessingException { JsonNode node = jp.getCodec().readTree(jp); // Read the Array or Arrays return new Candlestick(// Return with correct values read from Array); } }
ObjectMapper 使用該自定義反序列化器
// This will get the Spring ObjectMapper singleton instance. @Autowired ObjectMapper mapper; @PostConstruct public void init() throws Exception { SimpleModule module = new SimpleModule(); module.addDeserializer(Candlestick.class, new CandlestickDeserializer()); mapper.registerModule(module); } Candlestick readValue = mapper.readValue(json, Candlestick.class);
設計自定義解串器將是解決此問題的合理解決方案。 但是將數組映射到對象似乎是一項棘手的工作。 以@shazin 的想法為基礎,將數組數組映射到對象列表的自定義反序列化器可以寫成如下:
public class CStickListDeserializer extends StdDeserializer<ArrayList<Candlestick>> {
public CStickListDeserializer() {
this(null);
}
public CStickListDeserializer(Class<?> c) {
super(c);
}
@Override
public ArrayList<Candlestick> deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException {
Candlestick cs ;
ArrayList<Candlestick> cList = new ArrayList<Candlestick>();
BigDecimal[][] a = jp.readValueAs(BigDecimal[][].class);
for(BigDecimal[] a1 : a){
cs = new Candlestick();
cs.setTime(a1[0].longValue());
cs.setLow(a1[1]);
cs.setHigh(a1[2]);
cs.setOpen(a1[3]);
cs.setClose(a1[4]);
cs.setVolume(a1[5]);
cList.add(cs);
}
return cList;
}
}
用法:
ObjectMapper om = new ObjectMapper();
SimpleModule mod = new SimpleModule();
mod.addDeserializer(ArrayList.class, new CStickListDeserializer());
om.registerModule(mod);
ArrayList<Candlestick> CandlestickList = om.readValue(json, ArrayList.class);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.