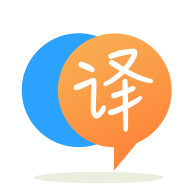
[英]django.db.utils.OperationalError: no such table Django 2
[英]Getting a django.db.utils.OperationalError: no such table With a Custom User Model
好吧,我在一個名為帳戶的應用程序中有一個自定義用戶模型。 accounts.User
看起來像這樣:
from django.contrib.auth.models import AbstractBaseUser
from django.core.validators import RegexValidator
from django.db import models
from django.db.models.signals import post_save
from django.dispatch import receiver
from accounts.managers import UserManager
from base.models import Model
from accounts.models.profiles import Profile
class User(Model, AbstractBaseUser):
objects = UserManager()
phone_regex = RegexValidator(
regex=r'^\+?1?\d{9,15}$',
message="Phone number must be entered in the format: '+999999999'. 9-15 digits allowed.",
)
phone = models.CharField(
validators=[phone_regex],
max_length=17,
blank=True
)
email = models.EmailField(
max_length=255,
unique=True,
)
active = models.BooleanField(
default=True
)
staff = models.BooleanField(
default=False
)
superuser = models.BooleanField(
default=False
)
USERNAME_FIELD = 'email'
REQUIRED_FIELDS = []
def __str__(self):
return self.email
def has_perm(self, perm, obj=None):
return True
def has_module_perms(self, app_label):
return True
@property
def is_active(self):
return self.active
@property
def is_staff(self):
return self.staff
@property
def is_superuser(self):
return self.superuser
def serialize(self):
data = {
'id': self.id,
'email': self.email,
}
return data
@receiver(post_save, sender=User)
def create_profile(sender, instance, created, **kwargs):
if created:
Profile.objects.create(user=instance)
@receiver(post_save, sender=User)
def save_profile(sender, instance, **kwargs):
instance.profile.save()
UserManager
看起來像這樣:
from django.contrib.auth.models import BaseUserManager
class UserManager(BaseUserManager):
def create_user(self, email, password):
if not email:
raise ValueError('Users must have an email')
if not password:
raise ValueError('Users must have a password')
user = self.model(
email=self.normalize_email(email),
)
user.set_password(password)
user.save(using=self._db)
return user
def create_staffuser(self, email, password):
user = self.create_user(
email=email,
password=password,
)
user.staff = True
user.save(using=self._db)
return user
def create_superuser(self, email, password):
user = self.create_user(
email=email,
password=password,
)
user.staff = True
user.superuser = True
user.save(using=self._db)
return user
我的帳戶應用程序是這樣注冊的:
...
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'base',
'accounts',
]
AUTH_USER_MODEL = 'accounts.User'
...
我運行python manage.py makemigrations
和python manage.py migrate
並且一切都按預期順利運行。 當我開始python manage.py createsuperuser
時會出現問題。 它提示按預期提供電子郵件輸入,但是當輸入電子郵件並單擊輸入時,它會返回一個錯誤。
回溯(最近一次調用最后一次):
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/utils.py", line 85, in _execute
return self.cursor.execute(sql, params)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/sqlite3/base.py", line 303, in execute
return Database.Cursor.execute(self, query, params)
sqlite3.OperationalError: no such table: accounts_user
上述異常是以下異常的直接原因:
回溯(最近一次調用最后一次):
File "manage.py", line 15, in <module>
execute_from_command_line(sys.argv)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/core/management/__init__.py", line 371, in execute_from_command_line
utility.execute()
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/core/management/__init__.py", line 365, in execute
self.fetch_command(subcommand).run_from_argv(self.argv)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/core/management/base.py", line 288, in run_from_argv
self.execute(*args, **cmd_options)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/contrib/auth/management/commands/createsuperuser.py", line 59, in execute
return super().execute(*args, **options)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/core/management/base.py", line 335, in execute
output = self.handle(*args, **options)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/contrib/auth/management/commands/createsuperuser.py", line 117, in handle
self.UserModel._default_manager.db_manager(database).get_by_natural_key(username)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/contrib/auth/base_user.py", line 44, in get_by_natural_key
return self.get(**{self.model.USERNAME_FIELD: username})
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/models/manager.py", line 82, in manager_method
return getattr(self.get_queryset(), name)(*args, **kwargs)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/models/query.py", line 397, in get
num = len(clone)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/models/query.py", line 254, in __len__
self._fetch_all()
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/models/query.py", line 1179, in _fetch_all
self._result_cache = list(self._iterable_class(self))
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/models/query.py", line 54, in __iter__
results = compiler.execute_sql(chunked_fetch=self.chunked_fetch, chunk_size=self.chunk_size)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/models/sql/compiler.py", line 1064, in execute_sql
cursor.execute(sql, params)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/utils.py", line 100, in execute
return super().execute(sql, params)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/utils.py", line 68, in execute
return self._execute_with_wrappers(sql, params, many=False, executor=self._execute)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/utils.py", line 77, in _execute_with_wrappers
return executor(sql, params, many, context)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/utils.py", line 85, in _execute
return self.cursor.execute(sql, params)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/utils.py", line 89, in __exit__
raise dj_exc_value.with_traceback(traceback) from exc_value
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/utils.py", line 85, in _execute
return self.cursor.execute(sql, params)
File "/Users/zulwiyozaputra/Developer/Environments/poros/lib/python3.6/site-packages/django/db/backends/sqlite3/base.py", line 303, in execute
return Database.Cursor.execute(self, query, params)
django.db.utils.OperationalError: no such table: accounts_user
我正在嘗試的是我嘗試通過刪除數據庫並進行遷移來重現錯誤,但我發現我的應用程序中沒有按預期創建遷移文件,因為它應該在數據庫中創建了我的自定義用戶的遷移文件。 以前有人遇到過這個錯誤嗎? 你是怎么解決的?
通過在我的應用程序中手動重新創建migrations/
文件夾解決的問題。 當我刪除數據庫和遷移文件時,該文件夾被刪除。 該文件夾不會使用python manage.py makemigrations
和python manage.py migrate
自動重新創建。
從AbstractBaseUser
創建自定義用戶模型時,您不需要將 Model 類添加為父類,只需保留AbstractBaseUser
:
class User(AbstractBaseUser)
在清除遷移文件並重新運行makemigrations
和migrate
。
我在使用 AbstractUser 時遇到了同樣的問題。 創建這些菜單字段。 然后添加到用戶
id
password
last_login
is_superuser
username
email
first_name
last_name
is_staff
is_active
date_joined
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.