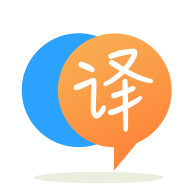
[英]How to delete a record from MySQL database using AJAX and PHP in Codeigniter
[英]How to delete Record from MySQL Table with AJAX?
我想用圖像X做一個按鈕,當您單擊按鈕時,僅在此刻它才刪除數據庫中的一行。
teste-checkbox.php
<style>
table {
font-family: verdana;
font-size: 12px;
}
table th {
text-align: left;
background: #D3D3D3;
padding: 2px;
}
table tr:hover {
background: #EFEFEF;
}
table tr {
text-align: left;
}
table td {
padding: 5px;
}
table td a {
color: #0454B5;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script>
<body>
<!--
T A B E L A «» C O M E Ç A
!-->
<?php
error_reporting(0);
$servername = "localhost";
$username = "PAP_Login";
$password = "pap123";
$dbname = "PAP_Login";
$datatable = "registo_anomalias"; // MySQL table name
$results_per_pagina = 5; // number of results per pagina
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
?>
<div class="um-bocado-para-cima">
<?php
if (isset($_GET["pagina"])) { $pagina = $_GET["pagina"]; } else { $pagina=1; };
$start_from = ($pagina-1) * $results_per_pagina;
$sql = "SELECT * FROM ".$datatable." ORDER BY deleted ASC LIMIT $start_from, ".$results_per_pagina;
$rs_result = $conn->query($sql);
?>
<table width="40%" border="0" class="hover">
<tr>
<th bgcolor="#CCCCCC"><strong>ID</strong></th>
<th bgcolor="#CCCCCC"><strong>Tipo de Sala</strong></th>
<th bgcolor="#CCCCCC"><strong>Tipo de Avaria</strong></th>
<th bgcolor="#CCCCCC"><strong>Data de Submissão</strong></th>
<th bgcolor="#CCCCCC"><strong>Hora de Submissão</strong></th>
<th bgcolor="#CCCCCC"><strong>Estado</strong></th>
<th bgcolor="#CCCCCC"><strong>Editar</strong></th>
</tr>
<?php
while($row = $rs_result->fetch_assoc()) {
?>
<?php
$tipo_de_sala = array(
'I' => 'Informática',
'N' => 'Normal',
'O' => 'Outro'
);
$deleted = array(
'0' => 'Não arranjado',
'1' => 'Arranjado'
);
?>
<tr id="<?php echo $row['id_avaria']; ?>" >
<td>
<a href="AvariaDocumento.php?id=<?php echo $row['id_avaria']; ?>"><?php echo $row['id_avaria']; ?></a>
</td>
<td><? echo strtr($row["tipo_sala"], $tipo_de_sala); ?></td>
<td><? echo $row["tipo_avaria"]; ?></td>
<td><? echo $row["data_subm"]; ?></td>
<td><? echo $row["hora_subm"]; ?></td>
<td>
<!-- consultar em https://pt.stackoverflow.com/questions/5675/como-verificar-se-um-checkbox-est%C3%A1-checado-com-php -->
<? echo strtr($row["deleted"], $deleted); ?>
</td>
<td></td>
<!--
<td>
<a href="EdicaoAvaria.php?id=<?php echo $row['id_avaria']; ?>"><img src="img/lapis.png"></a>
</td>
-->
</tr>
<?php
};
?>
</table>
</div>
<div class="pagination um-bocado-para-cima">
<?php
$sql = "SELECT COUNT(id_avaria) AS total FROM ".$datatable;
$result = $conn->query($sql);
$row = $result->fetch_assoc();
$total_paginas = ceil($row["total"] / $results_per_pagina); // calculate total paginas with results
if($row['total'] > 5){
for ($i=1; $i<=$total_paginas; $i++) { // print links for all paginas
echo "<a href='Administrador.php?pagina=".$i."'";
if ($i==$pagina) echo " class='curpage'";
echo ">".$i."</a> ";
}
}
?>
</div>
<?php
$id = $row['id_avaria'];
?>
<!--
T A B E L A «» T E R M I N A
!-->
</body>
<script>
$(document).ready(function() {
// show buttons on tr mouseover
$(".hover tr").live("mouseenter", function() {
$(this).find("td:last-child").html('<a href="javascript:void(0);" onClick="editrow(' + $(this).attr("id") + ')"><img src="img/lapis.png"></a> <a href="javascript:void(0);" onClick="deleterow(' + $(this).attr("id") + ') <span class="delete" id="del_<?php echo $row['id_avaria']; ?>"><img src="img/remover.png"></span></a>');
}); //
// remove button on tr mouseleave
$(".hover tr").live("mouseleave", function() {
$(this).find("td:last-child").html(" ");
});
// TD click event
$(".hover tr").live("click", function(event) {
if (event.target.nodeName == "TD") {
alert("You can track TD click event too....Isnt it amazing !!!");
}
});
});
editrow = function(itemId) {
alert("You clicked 'Edit' link with row id :" + itemId);
}
deleterow = function(itemId) {
}
</script>
<script>
$(document).ready(function(){
// Delete
$('.delete').click(function(){
var el = this;
var id = this.id;
var splitid = id.split("_");
// Delete id
var deleteid = splitid[1];
// AJAX Request
$.ajax({
url: 'remover-dados.php',
type: 'POST',
data: { id:deleteid },
success: function(response){
// Removing row from HTML Table
$(el).closest('tr').css('background','tomato');
$(el).closest('tr').fadeOut(800, function(){
$(this).remove();
});
}
});
});
});
</script>
<!--
http://makitweb.com/how-to-delete-record-from-mysql-table-with-ajax/
-->
remover-dados.php
<?php
error_reporting(0);
$servername = "localhost";
$username = "PAP_Login";
$password = "pap123";
$dbname = "PAP_Login";
$datatable = "registo_anomalias"; // MySQL table name
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
?>
<?php
$id = $_POST['id_avaria'];
// Delete record
$query = "DELETE FROM ".$datatable." WHERE id_avaria=".$id;
mysqli_query($con,$query);
echo 1;
我究竟做錯了什么?
您的問題是您通過GET方法發送了ID,並且想通過POST檢索它。
而不是使用
$id = $_POST['id_avaria'];
采用
$id = intval($_POST['id_avaria']);
還可以將class delete
添加到tag元素
刪除此功能,因為您根本不使用它
deleterow = function(itemId) {
}
通過調用刪除將代碼更改為:
<a class="delete"><span class="delete" data_id="<?php echo $row['id_avaria']; ?>"><img src="img/remover.png"></span></a>
最后一件事是防止click的默認行為並添加geting data-id
屬性
$('.delete').click(function(e){
e.preventDefault();
var deleteid = $(this).attr('data-id');
並且最好檢查是否通過了此變量,例如通過isset()函數+使用准備好的語句
完整代碼:
$id = intval($_GET['id_avaria']);
if (!empty($_GET['id_avaria']) {
$id = intval($_GET['id_avaria']);
$stmt = $mysqli->prepare("DELETE FROM ".$datatable." WHERE id_avaria=?");
$stmt->bind_param($stmt, "d", $id);
$stmt->execute($stmt);
if ($stmt->affected_rows($stmt) > 0) {
echo 'deleted';
} else {
echo 'failed to delete';
}
$stmt->close();
}
$conn->close();
在您的AJAX調用中,您調用參數id
// Delete id
var deleteid = splitid[1];
// AJAX Request
$.ajax({
url: 'remover-dados.php',
type: 'POST',
data: { id:deleteid },
// here ^^
success: function(response){
// Removing row from HTML Table
$(el).closest('tr').css('background','tomato');
$(el).closest('tr').fadeOut(800, function(){
$(this).remove();
});
}
});
因此,您的PHP應該在$_POST['id']
尋找該參數值
$id = $_POST['id'];
只需更換
$id = $_POST['id'];
嘗試這個-
$id = mysql_real_escape_string($_POST['id']);
$query ="DELETE FROM $datatable WHERE id_avaria='$id'";
mysqli_query($con,$query);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.