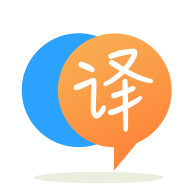
[英]Properly formatting a string given a python dictionary with keys that are tuples
[英]Convert a string to keys of a given dictionary in python
有沒有一種方法可以將字符串轉換為python中給定字典的鍵?
這是一個例子。 我有字典和字符串
dict = {0:'this', 1:'is', 2:'a', 3:'sentence', 4:'another'}
sentence = 'this is a sentence. this is another'
我想用字典將這個字符串轉換成這樣的東西:
[0, 1, 2, 3, 0, 1, 4]
是否有比嵌套循環遍歷句子中的單詞和詞典中的項更簡單的方法?
謝謝
首先,您需要反轉字典,然后使用re.findall
搜索字符串中的每個單詞:
import re
d = {0:'this', 1:'is', 2:'a', 3:'sentence', 4:'another'}
new_dict = {b:a for a, b in d.items()}
sentence = 'this is a sentence. this is another'
final_words = [new_dict[i] for i in re.findall('[a-zA-Z]+', sentence)]
輸出:
[0, 1, 2, 3, 0, 1, 4]
另外, dict
是內置函數,當用於存儲變量時,其原始功能將被覆蓋。 因此,最好不要使用內置函數的名稱,以免產生陰影。
另一個正則表達式解決方案(通過re.split()
函數):
import re
d = {0:'this', 1:'is', 2:'a', 3:'sentence', 4:'another'}
sentence = 'this is a sentence. this is another'
flipped = {v:k for k,v in d.items()}
result = [flipped.get(w) for w in re.split(r'\W+', sentence)]
print(result)
輸出:
[0, 1, 2, 3, 0, 1, 4]
\\W+
-匹配一個或多個空格字符 首先,不要用新的變量名隱藏dict
。
from re import findall
d = {0:'this', 1:'is', 2:'a', 3:'sentence', 4:'another'}
new_d = {v: k for k, v in d.iteritems()} # or .items() in Python 3.X
sentence = 'this is a sentence. this is another'
print(map(lambda x: new_d[x], findall(r'\w+', sentence))) # [0, 1, 2, 3, 0, 1, 4]
這是我使用清單推導在Python3中提出的一線風格建議。 此外,代碼的可讀性似乎被廣泛接受。
請注意, dict
是Python中內置類的名稱,一定不能將其用作變量名。 在以下代碼中,我用d
替換了您的dict
:
import re
d = {0:'this', 1:'is', 2:'a', 3:'sentence', 4:'another'}
sentence = 'this is a sentence. this is another'
result = [a for word in re.findall('\w+', sentence) for a,b in d.items() if word == b])
print(result)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.