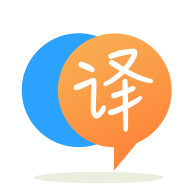
[英]Using validate.js to validate my TextInput results in undefined
[英]Error using validate.js on React Native - Unknown validator minimum
我在本機登錄屏幕上使用來自http://validatejs.org/ 的validate.js。
import React, { Component } from 'react';
import {
View,
Text,
TextInput,
TouchableOpacity } from 'react-native';
// Validate.js validates your values as an object
import validate from 'validate.js'
const constraints = {
email: {
presence: {
message: "Cannot be blank."
},
email: {
message: 'Please enter a valid email address'
}
},
password: {
presence: {
message: "Cannot be blank."
},
length: {
minimum: 5,
message: 'Your password must be at least 5 characters'
}
}
}
const validator = (field, value) => {
// Creates an object based on the field name and field value
// e.g. let object = {email: 'email@example.com'}
let object = {}
object[field] = value
let constraint = constraints[field]
console.log(object, constraint)
// Validate against the constraint and hold the error messages
const result = validate(object, constraint)
console.log(object, constraint, result)
// If there is an error message, return it!
if (result) {
// Return only the field error message if there are multiple
return result[field][0]
}
return null
}
export default class Login extends Component {
state = {
email: '',
emailError: null,
password: '',
passwordError: null,
}
logIn = () => {
let { email, password } = this.state;
console.log( email, password)
let emailError = validator('email', email)
let passwordError = validator('password', password)
console.log( emailError, passwordError)
this.setState({
emailError: emailError,
passwordError: passwordError,
})
}
render() {
const {emailError, passwordError } = this.state
return (
<View>
<TextInput
onChangeText={(email) => this.setState({email})}
placeholder="Email Address"
keyboardType='email-address'
autoCapitalize='none'
/>
<Text> {emailError ? emailError : null }</Text>
<TextInput
onChangeText={(password) => this.setState({password})}
placeholder="Password"
secureTextEntry={true}
autoCapitalize='none'
type="password"
/>
<Text> {passwordError ? passwordError : null }</Text>
<TouchableOpacity onPress={this.logIn}>
<Text>LOG IN</Text>
</TouchableOpacity>
</View>
);
}
}
運行上面的代碼會記錄以下內容並拋出錯誤“未知驗證器消息”
.. I ReactNativeJS: 't@t.cl', 'j'
.. I ReactNativeJS: { email: 't@t.cl' }, { presence: { message: 'Cannot be blank.' },
.. I ReactNativeJS: email: { message: 'Please enter a valid email address' } }
.. E ReactNativeJS: Unknown validator message
您的validate
調用是錯誤的。 應該是:
const result = validate(object, { [field]: constraint })
請注意,您的對象是:
{email: ...}
因此傳遞給驗證的約束也需要采用以下形式:
{email: emailConstraints }
發生的事情是驗證器查看email
並在那里尋找驗證器(約束),但它找到的只是message
並打印
未知驗證器“消息”
( "message"
是未知約束的名稱)。
試試這個,我在下面的函數中進行了更改,它可以工作。
const validator = (field, value) => {
// Creates an object based on the field name and field value
// e.g. let object = {email: 'email@example.com'}
let object = new Object()
object[field] = value
let constraint = new Object()
constraint[field] = constraints[field]
console.log(object, constraint)
// Validate against the constraint and hold the error messages
const result = validate({}, constraint)//
if (value != '' && value != null) {//if null value it will return with the presence validation
result = validate(object, constraint)
}
// If there is an error message, return it!
if (result) {
// Return only the field error message if there are multiple
return result[field][0]
}
return null
}
我設法讓它像這樣工作:
let emailConstraints;
<TextInput
onChangeText={(emailConstraints) => this.setState({email: emailConstraints })}
placeholder="Email Address"
keyboardType='email-address'
autoCapitalize='none'
/>
<Text> {emailError ? emailError : null }</Text>
我有這個特定的問題,但我可以說出問題是什么。 我的錯誤是Unknown validator checked
。 該問題由該錯誤消息中檢查的單詞指示。 這意味着屬性檢查(在我的情況下)和最小值(在你的情況下)在約束的 json 中的錯誤位置使用。 或者,它在正確的 json 塊中,但該塊中的一個或多個其他屬性不應該在那里,並且validate.js
解析器使用該塊中的第一個屬性報告錯誤,而不管它是否在正確的屬性中。 我有這個
policy: {
presence: true,
checked: true}
用於復選框驗證,但庫不允許在該塊中checked
未知屬性。 在你的情況下,我認為message
屬性不應該在length
塊中,但validate.js
使用該塊中的第一個屬性minimum
報告錯誤,否則在正確的位置。 所以你可能應該考慮刪除message
屬性,一切都會好起來的。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.