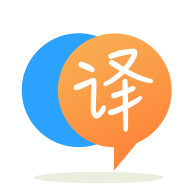
[英]How to read stored file using Multer disk storage option (NodeJs)?
[英]nodejs multer diskstorage to delete file after saving to disk
我正在使用 multer diskstorage 將文件保存到磁盤。 我首先將它保存到磁盤並對文件進行一些操作,然后我使用另一個 function 和 lib 將它上傳到遠程存儲桶。 上傳完成后,我想將其從磁盤中刪除。
var storage = multer.diskStorage({
destination: function (req, file, cb) {
cb(null, '/tmp/my-uploads')
},
filename: function (req, file, cb) {
cb(null, file.fieldname + '-' + Date.now())
}
})
var upload = multer({ storage: storage }).single('file')
這是我如何使用它:
app.post('/api/photo', function (req, res) {
upload(req, res, function (err) {
uploadToRemoteBucket(req.file.path)
.then(data => {
// delete from disk first
res.end("UPLOAD COMPLETED!");
})
})
});
我如何使用 diskStorage remove function 刪除臨時文件夾中的文件? https://github.com/expressjs/multer/blob/master/storage/disk.js#L54
我決定將其模塊化並將其放在另一個文件中:
const fileUpload = function(req, res, cb) {
upload(req, res, function (err) {
uploadToRemoteBucket(req.file.path)
.then(data => {
// delete from disk first
res.end("UPLOAD COMPLETED!");
})
})
}
module.exports = { fileUpload };
你並不需要使用multer
要刪除的文件,此外_removeFile
是一個私人的功能,你不應該使用。
您可以像往常一樣通過fs.unlink
刪除該文件。 因此,無論您在何處可以訪問req.file
,都可以執行以下操作:
const fs = require('fs')
const { promisify } = require('util')
const unlinkAsync = promisify(fs.unlink)
// ...
const storage = multer.diskStorage({
destination(req, file, cb) {
cb(null, '/tmp/my-uploads')
},
filename(req, file, cb) {
cb(null, `${file.fieldname}-${Date.now()}`)
}
})
const upload = multer({ storage: storage }).single('file')
app.post('/api/photo', upload, async (req, res) =>{
// You aren't doing anything with data so no need for the return value
await uploadToRemoteBucket(req.file.path)
// Delete the file like normal
await unlinkAsync(req.file.path)
res.end("UPLOAD COMPLETED!")
})
不需要 Multer。 只需使用此代碼。
const fs = require('fs')
const path = './file.txt'
fs.unlink(path, (err) => {
if (err) {
console.error(err)
return
}
//file removed
})
您也可以考慮使用MemoryStorage用於此目的,使用此存儲,文件永遠不會存儲在磁盤中,而是存儲在內存中,並且在執行離開控制器塊后自動從內存中刪除,即在您在大多數情況下提供響應之后案件。
當您使用此存儲選項時,您將不會得到字段file.destination
、 file.path
和file.filename
,而是會得到一個字段file.buffer
,顧名思義是一個緩沖區,您可以將此緩沖區轉換為所需的格式進行操作,然后使用流對象上傳。
大多數流行的庫都支持流,因此您應該能夠使用流直接上傳文件,將緩沖區轉換為流的代碼:
const Readable = require('stream').Readable;
var stream = new Readable();
stream._read = () => { }
stream.push(file.buffer);
stream.push(null);
// now you can pass this stream object to your upload function
這種方法會更有效,因為文件將存儲在內存中,這將導致更快的訪問,但它確實有multer
文檔中提到的multer
:
警告:在使用內存存儲時,上傳非常大的文件或大量相對較小的文件可能會導致應用程序內存不足。
為了在所有路線上真正自動執行此操作,我使用了以下策略:
當請求結束時,我們刪除所有上傳的文件(req.files)。 在此之前,如果要將文件保留在服務器上,則需要將它們保存在另一個路徑中。
var express = require('express');
var app = express();
var http = require('http');
var server = http.Server(app);
// classic multer instantiation
var multer = require('multer');
var upload = multer({
storage: multer.diskStorage({
destination: function (req, file, cb) {
cb(null, `${__dirname}/web/uploads/tmp/`);
},
filename: function (req, file, cb) {
cb(null, uniqid() + path.extname(file.originalname));
},
}),
});
app.use(upload.any());
// automatically deletes uploaded files when express finishes the request
app.use(function(req, res, next) {
var writeHead = res.writeHead;
var writeHeadbound = writeHead.bind(res);
res.writeHead = function (statusCode, statusMessage, headers) {
if (req.files) {
for (var file of req.files) {
fs.unlink(file.path, function (err) {
if (err) console.error(err);
});
}
}
writeHeadbound(statusCode, statusMessage, headers);
};
next();
});
// route to upload a file
router.post('/profile/edit', access.isLogged(), async function (req, res, next) {
try {
// we copy uploaded files to a custom folder or the middleware will delete them
for (let file of req.files)
if (file.fieldname == 'picture')
await fs.promises.copy(file.path, `${__dirname}/../uploads/user/photo.jpg`);
} catch (err) {
next(err);
}
});
使用 fs-extra 上傳文件后,我刪除了目錄
const fs = require('fs-extra');
// after you uploaded to bucket
await fs.remove('uploads/abc.png'); // remove upload dir when uploaded bucket
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.