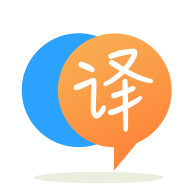
[英]How to return the correct day of the week given a date - year month and day
[英]How to calculate a Date given only month, week number within that month, and day of week
我正在調用一個返回以下對象的 api,告訴我夏令時從 3 月 11 日開始,適用於給定的時區:
{
dayOfWeek: "Sunday",
month: 3,
timeOfDay: "0001-01-01T02:00:00.000Z",
week: 2
}
在這種情況下,week:2 代表三月的第二周。
我如何從中創建 3 月 11 日? 我有以下功能在 3 月份運行良好,但意識到如果說 api 返回以下內容,這將在 4 月份失敗:
{
dayOfWeek: "Saturday",
month: 4,
timeOfDay: "0001-01-01T02:00:00.000Z",
week: 2
}
代碼:
/**
* Given the year, month, week day, week number of the month, and time, calculate a date
- set the date to the first day of the month
- then adjust the date backwards to the proper weekday
- then move it forward by 7 * week number to get it to the proper date
*/
calcDateFromDaylightSavingRulesAPI: function(year, month, time, dayOfWeek, weekNum) {
const weekDays = {
Sunday: 0,
Monday: 1,
Tuesday: 2,
Wednesday: 3,
Thursday: 4,
Friday: 5,
Saturday: 6
};
const date = new Date(year, month, 1, time);
date.setDate(date.getDate() + weekDays[dayOfWeek] - date.getDay());
date.setDate(date.getDate() + (weekNum * constants.DAYS_PER_WEEK));
return date;
如果您只想要一個月中某個特定日期的第 n 次出現,您可以獲取該月的第 1 次,移至該特定日期的第一次出現,然后添加(n-1) * 7
天以獲得第 n 次出現,例如
function getNthDay(year, month, dayName, n) { var weekDays = { Sunday: 0, Monday: 1, Tuesday: 2, Wednesday: 3, Thursday: 4, Friday: 5, Saturday: 6 }; // validate input values here, throw errors if required, eg // dayName must be in weekdays, 0 < n < 6 // Create date for first day of required month var d = new Date(year, month - 1, 1); // Set to first instance of particular day d.setDate((8 - (d.getDay() - weekDays[dayName]))%7); // Add (n-1)*7 days d.setDate(d.getDate() + (n-1) * 7); // Check final date is still in required month return d.getMonth() == month - 1? d : 'fail'; } // Second Sunday in March, 2018 console.log(getNthDay(2018,3,'Sunday',2).toString())
如果您想要第二周的星期日,則計算起來有點困難,因為有些地方從星期日開始,有些地方從星期一開始。 此外,一個月中一周的算法可能會因地而異(就像一年中的一周一樣)。
我認為這現在工作正常。 謝謝大家的幫助!
/**
* Given an object which contains month, time, week day, and week number in a month, calculate a date
- set the date to the first day of the month
- then adjust the date backwards to the proper weekday
- then move it forward by 7 * week number to get it to the proper date
*/
calcDateFromDaylightSavingRulesAPI: function(ruleSet) {
const today = new Date();
const year = today.getFullYear();
const month = ruleSet.month - 1;
const time = new Date(ruleSet.timeOfDay).getUTCHours();
const dayOfWeek = ruleSet.dayOfWeek;
const weekNum = ruleSet.week;
const weekDays = {
Sunday: 0,
Monday: 1,
Tuesday: 2,
Wednesday: 3,
Thursday: 4,
Friday: 5,
Saturday: 6
};
const date = new Date(year, month, 1, time);
const diff = weekDays[dayOfWeek] - date.getDay();
date.setDate(date.getDate() + (diff >= 0 ? diff - 7 : diff));
date.setDate(date.getDate() + (weekNum * constants.DAYS_PER_WEEK));
return date;
},
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.