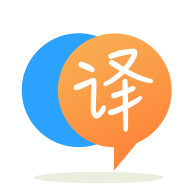
[英]How to POST/GET/PATCH/DELETE in SensorThings API Using JavaScript/jQuery
[英]How to store SensorThings query result into a variable in JavaScript/jQuery
我想讀從SensorThings服務器(事實體https://scratchpad.sensorup.com/OGCSensorThings/v1.0/由通過JavaScript / jQuery的使用GET方法),結果存儲到一個名為件事變量,以便我可以在腳本的另一部分中使用變量。
這是我的代碼:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Read Thing</title>
<link rel="stylesheet" href="main.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<h1>Read Thing</h1>
<h2 id="thing_name"></h2>
<script>
var thing = $.ajax({
url: "https://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)",
type: "GET",
contentType: "application/json; charset=utf-8",
success: function(data){
console.log(data);
return data;
},
error: function(response, status){
console.log(response);
console.log(status);
}
});
</script>
</body>
</html>
在瀏覽器中運行它之后,得到的結果如下:
{@iot.id: 1785996, @iot.selfLink: "http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)", description: "Thing Description 15", name: "Thing Name 16", properties: {…}, …}
@iot.id
:
1785996
@iot.selfLink
:
"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)"
Datastreams@iot.navigationLink
:
"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)/Datastreams"
HistoricalLocations@iot.navigationLink
:
"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)/HistoricalLocations"
Locations@iot.navigationLink
:
"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)/Locations"
description
:
"Thing Description 15"
name
:
"Thing Name 16"
properties
:
{Thing Property Name 16: "Thing Property Description 16", Thing Property Name 15: "Thing Property Description 15"}
__proto__
:
Object
基於該結果,我嘗試進行一些查詢:
>>> thing
{readyState: 4, getResponseHeader: ƒ, getAllResponseHeaders: ƒ, setRequestHeader: ƒ, overrideMimeType: ƒ, …}abort: ƒ (a)always: ƒ ()complete: ƒ ()done: ƒ ()error: ƒ ()fail: ƒ ()getAllResponseHeaders: ƒ ()getResponseHeader: ƒ (a)overrideMimeType: ƒ (a)pipe: ƒ ()progress: ƒ ()promise: ƒ (a)readyState: 4responseJSON: {@iot.id: 1785996, @iot.selfLink: "http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)", description: "Thing Description 15", name: "Thing Name 16", properties: {…}, …}responseText: "{"@iot.id":1785996,"@iot.selfLink":"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)","description":"Thing Description 15","name":"Thing Name 16","properties":{"Thing Property Name 16":"Thing Property Description 16","Thing Property Name 15":"Thing Property Description 15"},"Datastreams@iot.navigationLink":"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)/Datastreams","HistoricalLocations@iot.navigationLink":"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)/HistoricalLocations","Locations@iot.navigationLink":"http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)/Locations"}"setRequestHeader: ƒ (a,b)state: ƒ ()status: 200statusCode: ƒ (a)statusText: "OK"success: ƒ ()then: ƒ ()__proto__: Object
>>> thing.responseJSON
{@iot.id: 1785996, @iot.selfLink: "http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)", description: "Thing Description 15", name: "Thing Name 16", properties: {…}, …}
>>> thing.responseJSON["name"]
"Thing Name 16"
>>> var thing_name = thing.responseJSON["name"]
undefined
>>> thing_name
"Thing Name 16"
看來代碼成功運行了。 但是,當我將查詢插入腳本並嘗試在控制台中顯示輸出時(我僅在此處顯示script標記,其他行與上面的代碼相同),例如:
<script>
var thing = $.ajax({
url: "https://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)",
type: "GET",
contentType: "application/json; charset=utf-8",
success: function(data){
console.log(data);
return data;
},
error: function(response, status){
console.log(response);
console.log(status);
}
});
var thing_name = thing.responseJSON["name"];
console.log("Thing Name: " + thing_name);
// I want to insert the variable into a h2 tag in the page
document.querySelector("#thing_name").innerHTML = thing_name;
</script>
我收到這樣的錯誤:
Uncaught TypeError: Cannot read property 'responseJSON' of undefined
at read_thing.htm:36
(anonymous) @ read_thing.htm:36
read_thing.htm:27 {@iot.id: 1785996, @iot.selfLink: "http://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)", description: "Thing Description 15", name: "Thing Name 16", properties: {…}, …}
腳本似乎沒有將結果成功存儲到變量事物中 。 當我嘗試在控制台中顯示變量的內容時,輸出未定義 。
因此,如何修改腳本以將結果轉換為變量? 提前致謝。
干杯,
將腳本末尾附近的代碼移動到ajax調用的success
方法中,如下所示:
<script>
var thing = $.ajax({
url: "https://scratchpad.sensorup.com/OGCSensorThings/v1.0/Things(1785996)",
type: "GET",
contentType: "application/json; charset=utf-8",
success: function(data){
console.log(data);
var thing_name = thing.responseJSON["name"];
console.log("Thing Name: " + thing_name);
// I want to insert the variable into a h2 tag in the page
document.querySelector("#thing_name").innerHTML = thing_name;
return data;
},
error: function(response, status){
console.log(response);
console.log(status);
}
});
</script>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.