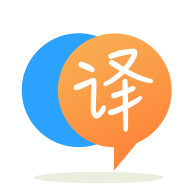
[英]Stored value disappears when setting a struct pointer to null in C++
[英]c++ Struct pointer unable to read memory when initialised as NULL
我正在創建一個包含二進制搜索樹算法的程序,但是遇到了一個不確定如何解決的問題。 這是我的代碼的相關位。
struct node {
string data;
node *left = NULL;
node *right = NULL;
};
多數民眾贊成在我的節點結構。
void Insert_Rec(string word, node* ptr) {
if (ptr->data == "") {
ptr->data = word;
ptr->left = NULL;
ptr->right = NULL;
cout << "overwitten!" << endl;
}
else if (word < ptr->data) {
if (ptr->left != NULL) {
cout << "Recursing left!";
Insert_Rec(word, ptr->left);
}
else {
ptr->data = word;
ptr->left = NULL;
ptr->right = NULL;
cout << "Inserted!";
}
}
問題就在這里,程序永遠不會輸入if(ptr-> left!= NULL)語句。 看着我的Visual Studio調試器,ptr-> left顯示“”而不是NULL。 我該如何解決! 我在這里嘗試了其他一些解決方案,但它們要么不相關,要么就不起作用!
程序永遠不會輸入if(ptr-> left!= NULL)語句
好ptr->left
開頭為NULL,並且您從不分配其他任何內容,因此它將永遠保持NULL。
if (ptr->left) {
cout << "Recursing left!";
Insert_Rec(word, ptr->left);
}
else {
/* this just overwrites the existing node in-place
but you should be creating a new node for the left child
ptr->data = word;
ptr->left = NULL;
ptr->right = NULL;
*/
ptr->left = new node{word, nullptr, nullptr};
cout << "Inserted!";
}
您的代碼還有很多其他問題(Vlad-from-Moscow的答案顯示了此函數的更好設計,但您確實需要在容器類級別對其進行修復),但這是立即阻止程序。
您的函數實現沒有整體意義。
該功能可以通過以下方式定義
void Insert_Rec( node **head, const std::string &word )
{
if ( *head == nullptr )
{
*head = new node { word, nullptr, nullptr };
}
else if ( word < ( *head )->data )
{
Insert_Rec( &( *head )->left, word );
}
else
{
Insert_Rec( &( *head )->right, word );
}
}
如果需要,此功能應分配一個新節點。
如果您希望BST不包含重復項,則將最后一個else
語句更改為else if
語句,例如
else if ( ( *head )->data < word )
該函數可以通過以下方式調用
node *head = nullptr;
//...
Insert_Rec( &head, "Hello" );
另外,您可以使用引用的類型作為第一個函數參數的類型,而不是“ double”指針。
例如
void Insert_Rec( node * &head, const std::string &word )
{
if ( head == nullptr )
{
head = new node { word, nullptr, nullptr };
}
else if ( word < head->data )
{
Insert_Rec( head->left, word );
}
else
{
Insert_Rec( head->right, word );
}
}
您已經在結構中設置了節點* left = NULL和節點* right = NULL。 刪除該NULL。 只留下兩個指針。 否則,它為空。 無論如何,請記住,很少要初始化結構體中的指針。
struct node {
// simple is better (-:
string data;
struct node *left;
struct node *right;
};
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.