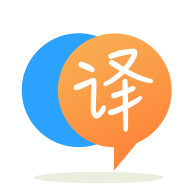
[英]Extract Google Drive multi zip from Google colab notebook
[英]Extract Google Drive zip from Google colab notebook
我已經在谷歌驅動器上有一個(2K 圖像)數據集的壓縮包。 我必須在 ML 訓練算法中使用它。 下面的代碼以字符串格式提取內容:
from pydrive.auth import GoogleAuth
from pydrive.drive import GoogleDrive
from google.colab import auth
from oauth2client.client import GoogleCredentials
import io
import zipfile
# Authenticate and create the PyDrive client.
# This only needs to be done once per notebook.
auth.authenticate_user()
gauth = GoogleAuth()
gauth.credentials = GoogleCredentials.get_application_default()
drive = GoogleDrive(gauth)
# Download a file based on its file ID.
#
# A file ID looks like: laggVyWshwcyP6kEI-y_W3P8D26sz
file_id = '1T80o3Jh3tHPO7hI5FBxcX-jFnxEuUE9K' #-- Updated File ID for my zip
downloaded = drive.CreateFile({'id': file_id})
#print('Downloaded content "{}"'.format(downloaded.GetContentString(encoding='cp862')))
但是我必須將其提取並存儲在一個單獨的目錄中,因為它更容易處理(以及理解)數據集。
我試圖進一步提取它,但得到“不是 zipfile 錯誤”
dataset = io.BytesIO(downloaded.encode('cp862'))
zip_ref = zipfile.ZipFile(dataset, "r")
zip_ref.extractall()
zip_ref.close()
注意:數據集僅供參考,我已經將這個 zip 下載到我的 google 驅動器中,我指的是驅動器中的文件。
你可以簡單地使用這個
!unzip file_location
要將文件解壓縮到目錄:
!unzip path_to_file.zip -d path_to_directory
要從 Google colab 筆記本中提取 Google Drive zip:
import zipfile
from google.colab import drive
drive.mount('/content/drive/')
zip_ref = zipfile.ZipFile("/content/drive/My Drive/ML/DataSet.zip", 'r')
zip_ref.extractall("/tmp")
zip_ref.close()
Colab 研究團隊有一個筆記本可以幫助您。
不過,簡而言之,如果您正在處理一個 zip 文件,就像我一樣,它主要是數千張圖像,我想將它們存儲在驅動器內的一個文件夾中,然后執行此操作 -
!unzip -u "/content/drive/My Drive/folder/example.zip" -d "/content/drive/My Drive/folder/NewFolder"
-u
部分僅在新/必要時控制提取。 如果您突然失去連接或硬件關閉,這一點很重要。
-d
創建目錄並將提取的文件存儲在那里。
當然,在執行此操作之前,您需要安裝驅動器
from google.colab import drive
drive.mount('/content/drive')
我希望這有幫助! 干杯!!
首先,在 colab 上安裝 unzip:
!apt install unzip
然后使用 unzip 解壓縮您的文件:
!unzip source.zip -d destination.zip
安裝 GDrive:
from google.colab import drive
drive.mount('/content/gdrive')
打開鏈接 -> 復制授權碼 -> 將其粘貼到提示符中,然后按“Enter”
檢查 GDrive 訪問:
!ls "/content/gdrive/My Drive"
從 GDrive 解壓縮(q 代表“安靜”)文件:
!unzip -q "/content/gdrive/My Drive/dataset.zip"
首先新建一個目錄:
!mkdir file_destination
現在,是時候用解壓后的文件來擴充目錄了:
!unzip file_location -d file_destination
對於 Python
連接到驅動器,
from google.colab import drive
drive.mount('/content/drive')
檢查目錄
!ls
和!pwd
用於解壓
!unzip drive/"My Drive"/images.zip
在驅動器上安裝后,使用shutil.unpack_archive 。 它適用於幾乎所有的存檔格式(例如,“zip”、“tar”、“gztar”、“bztar”、“xztar”),而且很簡單:
import shutil
shutil.unpack_archive("filename", "path_to_extract")
請在 google colab 中使用此命令
解壓要解壓的文件,然后解壓位置
!unzip "drive/My Drive/Project/yourfilename.zip" -d "drive/My Drive/Project/yourfolder"
而不是GetContentString()
,而是使用 GetContentFile() 。 它將保存文件而不是返回字符串。
downloaded.GetContentFile('images.zip')
然后您可以稍后使用unzip
壓縮它。
簡單的連接方式
1)您必須驗證身份驗證
from google.colab import auth
auth.authenticate_user()
from oauth2client.client import GoogleCredentials
creds = GoogleCredentials.get_application_default()
2)融合谷歌驅動器
!apt-get install -y -qq software-properties-common python-software-properties module-init-tools
!add-apt-repository -y ppa:alessandro-strada/ppa 2>&1 > /dev/null
!apt-get update -qq 2>&1 > /dev/null
!apt-get -y install -qq google-drive-ocamlfuse fuse
3) 驗證憑據
import getpass
!google-drive-ocamlfuse -headless -id={creds.client_id} -secret={creds.client_secret} < /dev/null 2>&1 | grep URL
vcode = getpass.getpass()
!echo {vcode} | google-drive-ocamlfuse -headless -id={creds.client_id} -secret={creds.client_secret}
4)創建一個驅動器名稱以在colab('gdrive')中使用它並檢查它是否工作
!mkdir gdrive
!google-drive-ocamlfuse gdrive
!ls gdrive
!cd gdrive
嘗試這個:
!unpack file.zip
如果它現在工作或文件是 7z 試試下面
!apt-get install p7zip-full
!p7zip -d file_name.tar.7z
!tar -xvf file_name.tar
或者
!pip install pyunpack
!pip install patool
from pyunpack import Archive
Archive(‘file_name.tar.7z’).extractall(‘path/to/’)
!tar -xvf file_name.tar
我們假設您已經將您的 googleDrive 安裝到 googleColab。 如果您只想提取包含 .csv 擴展名的 zip 文件。 只需調用 pandas 屬性 read_csv
pd.read_csv('/content/drive/My Drive/folder/example.zip')
如何在 Google Colab 中提取帶有密碼的 zip 文件? 我嘗試了很多不同的事情,但沒有任何運氣。
這對我有用。
!apt install unzip
然后我使用此代碼解壓縮文件
!unzip /content/file.zip -d /content/
如果不先在 Colab 上安裝unzip
,您將始終收到錯誤消息。
在我的想法中,您必須走一條特定的道路,例如:
從 google.colab 導入驅動器 drive.mount('/content/drive/') cd drive/MyDrive/f/
然后 :
!apt install unzip !unzip zip_folder.zip -d unzip_folder在此處輸入圖像描述
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.