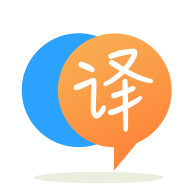
[英]How to parse a tree structure saved in an Excel file using Apache POI
[英]How to parse tree structure from excel file in JAVA
大家好! 我需要從Excel解析樹結構,例如:ABCDEFGKMO等,而文件沒有結束
我有一個實體類科:
@Getter
@Setter
public class Section {
private int depth;
private String text;
private int parentLevel;
private List<Section> children;
public Section(String text, int depth) {
this.text = text;
this.depth = depth;
this.children = new ArrayList<Section>();
this.parentLevel = 0;
}
public Section(String text, int depth, int parent) {
this.text = text;
this.depth = depth;
this.children = new ArrayList<Section>();
this.parentLevel = parent;
}
public boolean addChild(Section section) {
return children.add(section);
}
@Override
public String toString() {
return "Section{" + "depth=" + depth + ", text='" + text + '\''
+ ", children=" + children + '}';
}
}
和從excel讀取數據並嘗試解析的方法,但是我不知道如何以最佳方式解析它。 我無法將孩子名單與他們的父母聯系起來。
和方法:
public void read() throws ResourceNotFoundException {
try {
XSSFWorkbook excelBook = new XSSFWorkbook(new FileInputStream(ExcelConstant.FILE_NAME));
XSSFSheet excelSheet = excelBook.getSheet(ExcelConstant.SHEET_NAME);
Section prev = null;
int prevKey = 0;
for (Row row : excelSheet) {
for (Cell cell : row) {
if (cell.getCellType() == Cell.CELL_TYPE_STRING) {
int current = cell.getColumnIndex();
if (prev == null && current == 0) {
prev = new Section(cell.getStringCellValue(), current);
} else if (prev != null) {
if (current > prevKey) {
Section section = new Section(cell.getStringCellValue(), current, prevKey);
prev.addChild(section);
prevKey = current;
}
if (current == prevKey) {
Section section = new Section(cell.getStringCellValue(), current, prev.getParentLevel());
prev.addChild(section);
} else {
while (current < prev.getDepth()) {
prevKey = prev.getParentLevel();
}
Section t_section = new Section(cell.getStringCellValue(), current, prevKey);
prev.addChild(t_section);
}
}
}
}
}
System.out.println(prev);
} catch (IOException e) {
throw new ResourceNotFoundException("Read data from excel file exception", e);
}
}
解決以下問題:
GoF提供復合模式
這是一個示例Java程序:
Composite.java
package com.test;
import java.util.ArrayList;
import java.util.List;
public class Composite {
private String text;
private List<Composite> children = new ArrayList<>();
public Composite(String text) {
this.text = text;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public void add(Composite child) {
children.add(child);
}
public void remove(Composite child) {
children.remove(child);
}
public List<Composite> getChildren() {
return children;
}
@Override
public String toString() {
return "Composite [text=" + text + ", children=" + children + "]";
}
}
ExcelParser.java
package com.test;
import java.io.FileInputStream;
import java.util.LinkedHashMap;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelParser {
private Map<String, Composite> compositeMap = new LinkedHashMap<>();
public Composite parse() throws Exception {
Composite root = new Composite("Root");
String[][] array = getArrayFromExcel();
for (int i = 0; i < array.length; i++) {
String[] row = array[i];
for (int j = row.length - 1; j > -1; j--) {
Composite current = compositeMap.get("" + i + j);
Composite parent = compositeMap.get("" + i + (j - 1));
if (current != null) {
if (j - 1 < 0) {
parent = root;
} else if (parent == null) {
for (int k = i - 1; k > -1; k--) {
parent = compositeMap.get("" + k + (j - 1));
if (parent != null) {
break;
}
}
}
if (parent == null) {
throw new Exception("Invalid Input");
} else {
parent.getChildren().add(current);
}
}
}
}
return root;
}
public String[][] getArrayFromExcel() throws Exception {
XSSFWorkbook excelBook = new XSSFWorkbook(new FileInputStream("Book1.xlsx"));
XSSFSheet excelSheet = excelBook.getSheet("Sheet1");
int rowNums = excelSheet.getPhysicalNumberOfRows();
int colNums = 0;
for (Row row : excelSheet) {
if (colNums < row.getLastCellNum()) {
colNums = row.getLastCellNum();
}
}
String[][] array = new String[rowNums][colNums];
int i = 0;
for (Row row : excelSheet) {
int j = 0;
for (Cell column : row) {
j = column.getColumnIndex();
String text = column.getStringCellValue();
array[i][j] = text;
compositeMap.put("" + i + j, new Composite(text));
j++;
}
i++;
}
print(array);
return array;
}
private void print(String[][] array) {
for (String[] row : array) {
for (String data : row) {
System.out.print(data + "\t");
}
System.out.println();
}
}
}
Main.java
com.test;
public class Main {
public static void main(String[] args) throws Exception {
ExcelParser parser = new ExcelParser();
Composite root = parser.parse();
System.out.println(root);
}
}
輸出:
A A_1 null null
null A_2 null null
B B_1 null null
C null null null
D D_1 D_1_1 null
null null D_1_2 D_1_2_1
null null null D_1_2_2
null null null D_1_2_3
null D_2 null null
null D_3 null null
null D_4 null null
E null null null
Composite [text=Root, children=[Composite [text=A, children=[Composite [text=A_1, children=[]], Composite [text=A_2, children=[]]]], Composite [text=B, children=[Composite [text=B_1, children=[]]]], Composite [text=C, children=[]], Composite [text=D, children=[Composite [text=D_1, children=[Composite [text=D_1_1, children=[]], Composite [text=D_1_2, children=[Composite [text=D_1_2_1, children=[]], Composite [text=D_1_2_2, children=[]], Composite [text=D_1_2_3, children=[]]]]]], Composite [text=D_2, children=[]], Composite [text=D_3, children=[]], Composite [text=D_4, children=[]]]], Composite [text=E, children=[]]]]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.