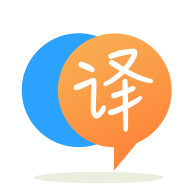
[英]How can I test for — void add(Object val) and Object at(int index) using jUnit testing(java)?
[英]how can I use fill(int[] a, int from_Index, int to_Index, int val) for matrix in Java?
我想編寫一個程序來計算不同窗口在屏幕上覆蓋的面積。 我有窗口的數量,每個窗口的x1,y1,x2,y2作為輸入。 我想在Java中使用填充功能。 我想創建一個桌面大小的矩陣,全為零。 對於每個窗口,我想獲取窗口的寬度並將其輸入到矩陣中,獲取與窗口高度一樣多的行,並針對所有窗口執行此操作,然后通過對所得的矩陣進行計數來匯總得出的矩陣,並為我提供面積覆蓋在屏幕上,而不必處理重疊的窗口。 但我不知道如何使用填充矩陣。
假設您有一個2維數組,例如matrix[WIDTH][HEIGHT]
。
您可以使用Arrays.fill()
方法通過一次調用來填充整列。 但是,您仍然需要遍歷各列。
我創建了一個小程序來說明。 希望能幫助到你:
import java.util.*;
public class TestArray
{
public static final int SCREEN_WIDTH = 100;
public static final int SCREEN_HEIGHT = 100;
class WindowCoords
{
public int top;
public int left;
public int bottom;
public int right;
public WindowCoords(int top,
int left,
int bottom,
int right)
{
this.top = top;
this.left = left;
this.bottom = bottom;
this.right = right;
}
} // class WindowCoords
public List<WindowCoords> getWindows()
{
List<WindowCoords> result;
result = new ArrayList<WindowCoords>();
result.add(new WindowCoords(4, 67, 23, 89));
result.add(new WindowCoords(18, 12, 65, 30));
result.add(new WindowCoords(45, 3, 95, 15));
result.add(new WindowCoords(67, 40, 93, 59));
return (result);
}
public void run()
{
// Initialize matrix
// Setting its contents to 0 not strictly necessary though I prefer to do so
int[][] matrix;
int column;
matrix = new int[SCREEN_WIDTH][SCREEN_HEIGHT];
//for (column = 0; column < SCREEN_WIDTH; column++)
// Arrays.fill(matrix[column], 0);
// Get windows
List<WindowCoords> windows;
windows = getWindows();
// Fill covered screen
Iterator<WindowCoords> it;
WindowCoords window;
it = windows.iterator();
while (it.hasNext())
{
window = it.next();
for (column = window.left; column <= window.right; column++)
Arrays.fill(matrix[column], window.top, window.bottom, 1);
}
// Show result
int row;
for (row = 0; row < SCREEN_HEIGHT; row++)
{
for (column = 0; column < SCREEN_WIDTH; column++)
System.out.print(matrix[column][row]);
System.out.println();
}
} // run
public static void main(String[] args)
{
TestArray test;
test = new TestArray();
test.run();
}
} // class TestArray
只需一個簡單的嵌套循環即可:
public static void main(String[] args) {
int[][] matrix = new int[10][10];
fill(matrix, 2, 2, 4, 4);
System.out.println(Arrays.deepToString(matrix));
}
private static void fill(int[][] matrix, int x1, int y1, int x2, int y2) {
for (int y = y1; y <= y2; y++) {
for (int x = x1; x <= x2; x++) {
matrix[y][x] = 1;
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.