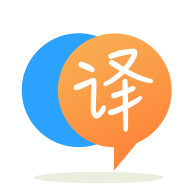
[英]How do I change settings.py to migrate from SQLite3 db to a mysql db?
[英]How do I migrate rows from a local SQLite DB to a remote MySQL DB using SQLAlchemy?
我正在使用SQLAlchemy管理2個數據庫。 一個是本地SQLite數據庫,另一個是Google的雲平台上的遠程MySQL數據庫,它們都共享相同的架構。 我正在嘗試使用將SQLite數據庫中包含的行追加到MySQL數據庫。 我目前有一個文件來定義我的班級,如下所示:
SensorCollection.py
class Readings(Base):
__tablename__ = 'readings'
time = Column(String(250), primary_key=True)
box_name = Column(String(250))
FS = Column(Numeric)
IS = Column(Numeric)
VS = Column(Numeric)
CO = Column(Numeric)
TVOC = Column(Numeric)
cTemp = Column(Numeric)
fTemp = Column(Numeric)
humidity = Column(Numeric)
pressure = Column(Numeric)
然后,我還有另一個文件聲明了我的Base,Session和engine:
base.py
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
engine = create_engine('sqlite:///%s' % 'db/db.db')
Session = sessionmaker(bind=engine)
Base = declarative_base()
最后是我的實際代碼:
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
from sqlalchemy.schema import Table
from SensorCollection import Readings
from sqlalchemy.sql.expression import insert
# uploads MySQL database to Google cloud server
# uses ORM to ensure the databases can communicate with each other
user = ***
password = ***
host = ***
port = ***
dbName = ***
instanceConnectionName = ***
# create the engine for the local db
engineLocal = create_engine('sqlite:///%s' % 'db/db.db')
BaseLocal = Readings(None, None, None, None, None, None, None, None, None, None, None)
# create the engine for the Google db
engineGoogle = create_engine('mysql+mysqldb://%s@%s:%s/%s?unix_socket=/cloudsql/%s' % (user, host, port, dbName, instanceConnectionName))
SessionGoogle = sessionmaker(bind=engineGoogle)
BaseGoogle = Readings(None, None, None, None, None, None, None, None, None, None, None)
# create the local db
BaseLocal.metadata.create_all(engineLocal)
# create the Google db and start the session
BaseGoogle.metadata.create_all(engineGoogle)
sessionGoogle = SessionGoogle()
# create table objects for each db
t1 = Table('readings', BaseLocal.metadata, autoload=True, autoload_with=engineLocal)
t2 = Table('readings', BaseGoogle.metadata, autoload=True, autoload_with=engineGoogle)
# the first subquery, select all ids from SOME_TABLE where some_field is not NULL
s1 = t1.select()
# the second subquery, select all ids from SOME_TABLE where some_field is NULL
s2 = t2.select()
# union s1 and s2 subqueries together and alias the result as "alias_name"
q = s1.union(s2)
insert(t2, s1)
sessionGoogle.query(q)
# sessionGoogle.add_all(s1)
# commit changes and close the session
sessionGoogle.commit()
sessionGoogle.close()
目前,SQLite數據庫已經存在,可以設置遠程服務器,但是當它連接到Google時,該代碼甚至無法創建該表。 似乎連接正確,因為如果我更改任何服務器詳細信息,都會收到MySQL錯誤。 它也不會給我任何錯誤。 它可以編譯並運行,但不追加數據甚至不創建表。 這是我第一次嘗試破解任何一種數據庫,因此我確信它充滿了錯誤,而且一點也不優雅。 任何幫助,將不勝感激。
Edit djkern幫助我創建了表,並且我更新了代碼。 不過,我仍然沒有要附加的數據。
在您的代碼中,您具有:
# create the Google db and start the session
BaseLocal.metadata.create_all(engineLocal)
sessionGoogle = SessionGoogle()
你的意思是這個嗎?
# create the Google db and start the session
BaseGoogle.metadata.create_all(engineGoogle)
sessionGoogle = SessionGoogle()
需要再添加兩個類,
class ReadingsLocal(BaseLocal):
和
class ReadingsGoogle(BaseGoogle):
它們應與以下文件位於同一文件中,並且與
class Readings(Base):
還必須創建新的Base,engine和Session變量。 然后,代碼應如下所示:
# create the local db
BaseLocal.metadata.create_all(engineLocal)
sessionLocal = SessionLocal()
# create the Remote db and start the session
BaseGoogle.metadata.create_all(engineGoogle)
sessionGoogle = SessionGoogle()
for reading in sessionLocal.query(ReadingsLocal):
# reading is already attached to a session, so a tmp variable must be created to avoid conflicts
tmp = ReadingsGoogle(reading.time, reading.box_name, reading.FS, reading.IS, reading.VS, reading.CO, reading.TVOC,
reading.cTemp, reading.fTemp, reading.humidity, reading.pressure)
sessionGoogle.add(tmp)
# commit changes and close the session
sessionGoogle.commit()
sessionGoogle.close()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.