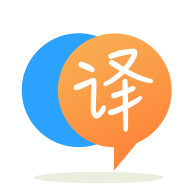
[英]How to have a value decrease or stay the same after a general function call?
[英]How can I determine if the numbers in a list initially increase (or stay the same) and then decrease (or stay the same) with Python?
例如, 123431
和4577852
的數字4577852
增加然后減少。 我寫了一個代碼,將數字分成一個列表,並能夠判斷所有數字是否增加或所有數字是否減少,但我不知道如何檢查數字增加然后減少。 我該如何延長?
x = int(input("Please enter a number: "))
y = [int(d) for d in str(x)]
def isDecreasing(y):
for i in range(len(y) - 1):
if y[i] < y[i + 1]:
return False
return True
if isDecreasing(y) == True or sorted(y) == y:
print("Yes")
找到最大元素。 在該位置將列表分成兩部分。 檢查第一件是否增加,第二件減少。
4577852
,您找到最大的元素, 8
。 4577
和852
(8可以進入任何列表,兩者都可以,或者兩者都沒有)。 4577
是否正在增加(好), 852
正在減少(也沒關系)。 這足以讓你找到解決方案嗎?
似乎是學習使用itertools和生成器管道的好機會。 首先,我們制作一些簡單,分離和可重用的組件:
from itertools import tee, groupby
def digits(n):
"""420 -> 4, 2, 0"""
for char in str(n):
yield int(char)
def pairwise(iterable):
"""s -> (s0,s1), (s1,s2), (s2, s3), ..."""
a, b = tee(iterable)
next(b, None)
return zip(a, b)
def deltas(pairs):
"""2 5 3 4 -> 3, -2, 1"""
for left, right in pairs:
yield right - left
def directions(deltas):
"""3 2 2 5 6 -> -1, 0, 1, 1"""
for delta in deltas:
yield -1 if delta < 0 else +1 if delta > 0 else 0
def deduper(directions):
"""3 2 2 5 6 2 2 2 -> 3, 2, 5, 6, 2"""
for key, group in groupby(directions):
yield key
然后我們將各個部分放在一起以解決檢測“增加然后減少數量”的更廣泛問題:
from itertools import zip_longest
def is_inc_dec(stream, expected=(+1, -1)):
stream = pairwise(stream)
stream = deltas(stream)
stream = directions(stream)
stream = deduper(stream)
for actual, expected in zip_longest(stream, expected):
if actual != expected or actual is None or expected is None:
return False
else:
return True
用法是這樣的:
>>> stream = digits(123431)
>>> is_inc_dec(stream)
True
此解決方案將正確短路,例如:
121111111111111111111111111111111111111111111111111 ... 2
我只解決了“嚴格增加,然后嚴格減少”的案例。 因為這聽起來像是你的家庭作業,所以我將把它作為練習讓你調整代碼以適應問題標題中提到的“不減少然后不增加”的情況。
將列表拆分為最大值,然后取每邊差異的最小值/最大值:
import numpy as np
test1 = [1, 2, 3, 4, 5, 8, 7, 3, 1, 0]
test2 = [1, 2, 3, 4, 5, 8, 7, 3, 1, 0, 2, 5]
test3 = [7, 1, 2, 3, 4, 5, 8, 7, 3, 1, 0]
test4 = [1, 2, 3, 4, 5, 8, 8, 7, 3, 1, 0]
def incdec_test(x):
i = np.array(x).argmax()
return (np.diff(x[0:i]).min() >= 0) and (np.diff(x[i:-1]).max() <= 0)
for test in [test1, test2, test3, test4]:
print 'increase then decrease = {}'.format(incdec_test(test))
結果:
increase then decrease = True
increase then decrease = False
increase then decrease = False
increase then decrease = False
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.