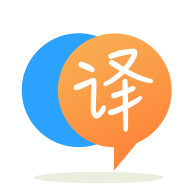
[英]How to check if phone number is already registered in firebase authentication using flutter
[英]How to do Phone Authentication in Flutter using Firebase?
我搜索了很多網站,但沒有找到使用 Firebase 在 Flutter 中實現電話身份驗證的方法。 誰能告訴我怎么做?
有據可查的工作演示項目在這里
下面是詳細流程
AuthCrendential
對象AuthCredential
對象是唯一用於登錄用戶的對象。 據或者從獲得verificationCompleted
回調函數在verifyPhoneNumber
或從PhoneAuthProvider
。(不要擔心它是否令人困惑,繼續閱讀,你會明白的)
phoneNumber
phoneNumber
的 SIM 卡不在當前運行該應用程序的設備中,
AuthCredential
對象AuthCredential
來登入此方法,即使phoneNumber
是在設備verificationCompleted
從回調submitPhoneNumber
函數給出AuthCredential
這是需要登入用戶對象Future<void> _submitPhoneNumber() async {
/// NOTE: Either append your phone number country code or add in the code itself
/// Since I'm in India we use "+91 " as prefix `phoneNumber`
String phoneNumber = "+91 " + _phoneNumberController.text.toString().trim();
print(phoneNumber);
/// The below functions are the callbacks, separated so as to make code more readable
void verificationCompleted(AuthCredential phoneAuthCredential) {
print('verificationCompleted');
...
this._phoneAuthCredential = phoneAuthCredential;
print(phoneAuthCredential);
}
void verificationFailed(AuthException error) {
...
print(error);
}
void codeSent(String verificationId, [int code]) {
...
print('codeSent');
}
void codeAutoRetrievalTimeout(String verificationId) {
...
print('codeAutoRetrievalTimeout');
}
await FirebaseAuth.instance.verifyPhoneNumber(
/// Make sure to prefix with your country code
phoneNumber: phoneNumber,
/// `seconds` didn't work. The underlying implementation code only reads in `milliseconds`
timeout: Duration(milliseconds: 10000),
/// If the SIM (with phoneNumber) is in the current device this function is called.
/// This function gives `AuthCredential`. Moreover `login` function can be called from this callback
verificationCompleted: verificationCompleted,
/// Called when the verification is failed
verificationFailed: verificationFailed,
/// This is called after the OTP is sent. Gives a `verificationId` and `code`
codeSent: codeSent,
/// After automatic code retrival `tmeout` this function is called
codeAutoRetrievalTimeout: codeAutoRetrievalTimeout,
); // All the callbacks are above
}
void _submitOTP() {
/// get the `smsCode` from the user
String smsCode = _otpController.text.toString().trim();
/// when used different phoneNumber other than the current (running) device
/// we need to use OTP to get `phoneAuthCredential` which is inturn used to signIn/login
this._phoneAuthCredential = PhoneAuthProvider.getCredential(
verificationId: this._verificationId, smsCode: smsCode);
_login();
}
Future<void> _login() async {
/// This method is used to login the user
/// `AuthCredential`(`_phoneAuthCredential`) is needed for the signIn method
/// After the signIn method from `AuthResult` we can get `FirebaserUser`(`_firebaseUser`)
try {
await FirebaseAuth.instance
.signInWithCredential(this._phoneAuthCredential)
.then((AuthResult authRes) {
_firebaseUser = authRes.user;
print(_firebaseUser.toString());
});
...
} catch (e) {
...
print(e.toString());
}
}
Future<void> _logout() async {
/// Method to Logout the `FirebaseUser` (`_firebaseUser`)
try {
// signout code
await FirebaseAuth.instance.signOut();
_firebaseUser = null;
...
} catch (e) {
...
print(e.toString());
}
}
有關實現的更多詳細信息,請參閱此處的lib/main.dart
文件。
如果您發現問題,歡迎對此答案和此repo README 進行編輯
目前_signInPhoneNumber
已棄用,因此請使用:
try {
AuthCredentialauthCredential = PhoneAuthProvider.getCredential(verificationId: verificationId, verificationsCode: smsCode);
await _firebaseAuth
.signInWithCredential(authCredential)
.then((FirebaseUser user) async {
final FirebaseUser currentUser = await _firebaseAuth.currentUser();
assert(user.uid == currentUser.uid);
print('signed in with phone number successful: user -> $user');
}
}
我有同樣的問題,但我正在構建一個 Ionic 應用程序。
您可以在網上進行 Firebase 電話身份驗證。 這個想法是:
創建一個 recaptchaVerifier
var recaptchaVerifier = new firebase.auth.RecaptchaVerifier('recaptcha-container');
使用電話號碼登錄用戶:
firebase.auth().signInWithPhoneNumber(phoneNumber, recaptchaVerifier) .then(confirmationResult => { myVerificationId = confirmationResult.verificationId; }) .catch(error => { console.log('error', error); })
通過深層鏈接將確認 ID 發送回 Flutter 應用程序
Firebase 將發送短信代碼。
使用任何將確認 ID 和代碼作為參數的方法登錄用戶。 對於網絡,它是這樣的:
let signinCredintial = firebase.auth.PhoneAuthProvider.credential(this.verificationId, this.code); firebase.auth().signInWithCredential(signinCredintial) .then(response => { // user is signed in })
以下是步驟:-
根據電話號碼獲取 OTP:-
void sendOTP(String phoneNumber, PhoneCodeSent codeSent, PhoneVerificationFailed verificationFailed) { if (!phoneNumber.contains('+')) phoneNumber = '+91' + phoneNumber; _firebaseAuth.verifyPhoneNumber( phoneNumber: phoneNumber, timeout: Duration(seconds: 30), verificationCompleted: null, verificationFailed: verificationFailed, codeSent: codeSent, codeAutoRetrievalTimeout: null); }
使用 codeSent 函數檢索 verify_id 並將其傳遞給 OTP 屏幕
void codeSent(String verificationId, [int forceResendingToken]) { Navigator.push( context, MaterialPageRoute( builder: (context) => Otp( phoneNumber: _phoneNumber, verificationId: verificationId, ))); }
根據 verify_id 和 OTP 獲取用戶
Future<bool> verifyOTP(String verificationId, String otp) async { AuthCredential credential = PhoneAuthProvider.getCredential( verificationId: verificationId, smsCode: otp, ); AuthResult result; try { result = await _firebaseAuth.signInWithCredential(credential); } catch (e) { // throw e; return false; } print(result); (await result.user.getIdToken()).claims.forEach((k, v) { print('k= $k and v= $v'); }); if (result.user.uid != null) return true; return false; }
欲了解更多詳情,請觀看以下視頻
我已經實現了帶有 firebase 的 phone auth singnIn in flutter 它很容易,只需導入 firebase_auth 庫並驗證電話號碼的格式是否正確,即它的開頭有一個“+”號,然后是國家/地區代碼,然后是電話號碼,然后是代碼像這樣
if (phoneExp.hasMatch(phon))
{
final PhoneVerificationCompleted verificationCompleted=(FirebaseUser user){
setState(() {
_message=Future<String>.value("auto sign in succedded $user");
debugPrint("Sign up succedded");
_pref.setString("phonkey",user.phoneNumber.toString());
MyNavigator.goToDetail(context);
//called when the otp is variefied automatically
});
};
final PhoneVerificationFailed verificationFailed=(AuthException authException){
setState(() {
_message=Future<String>.value("verification failed code: ${authException.code}. Message: ${authException.message}");
});
};
final PhoneCodeSent codeSent=(String verificationId,[int forceResendingToken]) async {
this.verificationId=verificationId;
};
final PhoneCodeAutoRetrievalTimeout codeAutoRetrievalTimeout = (String verificationId){
this.verificationId=verificationId;
};
await _auth.verifyPhoneNumber(
phoneNumber: phon,
timeout: Duration(seconds: 60),
verificationCompleted: verificationCompleted,
verificationFailed: verificationFailed,
codeSent: codeSent,
codeAutoRetrievalTimeout: codeAutoRetrievalTimeout
);
}
如果手機無法檢測到自動發送的 otp,則獲取字符串中的 otp 並實現此功能
void _signInWithOtp() async{
final FirebaseUser user = await _auth.signInWithPhoneNumber(
verificationId: verificationId,
smsCode: _otpController.text,
);
使用flutter_otp包輕松發送帶有 OTP 的短信。 優點是:
使用示例:
import 'package:flutter_otp/flutter_otp.dart';
...
FlutterOtp myOtpObj = FlutterOtp();
myOtpObj.sendOtp('7975235555');
...
// you can check as follows
bool correctOrNot = myOtpObj.resultChecker(<OTP entered by user>);
在此處查看 flutter_otp 存儲庫
我一步一步解釋了存儲庫的源代碼檢查下面的存儲庫可以幫助你https://github.com/myvsparth/flutter_otp_auth
在 firebase 的幫助下,我在 flutter 中實現移動驗證時也遇到了困難。 經過這么多的研究和理解,我制作了一個視頻並上傳到我的頻道,以便它可以幫助其他人。
請看看這個視頻可能對你有幫助。 https://youtu.be/9U-BNxZbZXY
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.