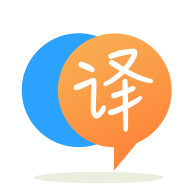
[英]Javascript flatten deeply nested Array with objects and renaming properties
[英]Flatten nested array of objects, renaming keys to an iterator
我有一個對象數組,每個對象如下所示(輪詢響應):
{
"slug": "18-AZ-Gov-GE-DvF",
"name": "2018 Arizona Gubernatorial GE",
"tags": [],
"charts": [],
"election_date": "2018-11-06",
"n_polls": 1,
"created_at": "2017-06-13T13:32:26.000Z",
"responses": [
{
"label": "Ducey",
"name": "Doug Ducey",
"party": "Republican",
"incumbent": true
},
{
"label": "Farley",
"name": "Steve Farley",
"party": "Democrat",
"incumbent": false
},
{
"label": "Other",
"name": "Other",
"party": null,
"incumbent": false
},
{
"label": "Undecided",
"name": "Undecided",
"party": null,
"incumbent": false
}
]
},
我需要展平由responses
鍵訪問的數組,以便將每個對象鍵接到其迭代器。
最終對象應如下所示:
{
"slug": "18-AZ-Gov-GE-DvF",
"name": "2018 Arizona Gubernatorial GE",
"tags": [],
"charts": [],
"election_date": "2018-11-06",
"n_polls": 1,
"created_at": "2017-06-13T13:32:26.000Z",
"label1": "Ducey",
"name1": "Doug Ducey",
"party1": "Republican",
"incumbent1": true
"label2": "Farley",
"name2": "Steve Farley",
"party2": "Democrat",
"incumbent2": false
"label3": "Other",
"name3": "Other",
"party3": null,
"incumbent3": false
"label4": "Undecided",
"name4": "Undecided",
"party4": null,
"incumbent4": false
},
我嘗試了幾種解決方案,但想在真正開始之前先看看是否有一種簡便的es6方法。
在這里使用array .reduce
方法可能效果很好。 由於reduce
回調將接收當前索引作為第三個參數,因此您可以使用該索引創建所需的鍵。
例:
const myArray = [ { "label": "Ducey", "name": "Doug Ducey", "party": "Republican", "incumbent": true }, { "label": "Farley", "name": "Steve Farley", "party": "Democrat", "incumbent": false }, { "label": "Other", "name": "Other", "party": null, "incumbent": false }, { "label": "Undecided", "name": "Undecided", "party": null, "incumbent": false } ] const flattened = myArray.reduce((flat, item, index) => ({ ...flat, ...Object.keys(item).reduce((numbered, key) => ({ ...numbered, [key + (index+1)]: item[key], }), {}), }), {}); console.log(flattened);
您可以使用forEach
遍歷responses
數組,並在對象上為每個對象中的entries
分配一個新鍵。 之后,只需delete
原始responses
數組即可:
let obj = {"slug": "18-AZ-Gov-GE-DvF","name": "2018 Arizona Gubernatorial GE","tags": [],"charts": [],"election_date": "2018-11-06","n_polls": 1,"created_at": "2017-06-13T13:32:26.000Z","responses": [ { "label": "Ducey", "name": "Doug Ducey", "party": "Republican", "incumbent": true }, { "label": "Farley", "name": "Steve Farley", "party": "Democrat", "incumbent": false }, { "label": "Other", "name": "Other", "party": null, "incumbent": false }, { "label": "Undecided", "name": "Undecided", "party": null, "incumbent": false }]} obj.responses.forEach((item, i) => { // item is one object from responses // i is the index starting at 0. Concat that on the key Object.entries(item).forEach(([k, v]) => obj[k+(i+1)] = v) }) // no need for obj.responses any more delete obj.responses console.log(obj)
一種方法是使用一些較新的功能,包括spread
, destructuring assignment
, template literals
, entries
以及最重要的是reduce
。
主要要點是使用化簡器將responses
數組轉換為每個元素對象的新對象,並使用輔助化簡器用迭代器計數修改鍵,並使用新鍵將值分配給外部對象。
這種方法的主要好處是原始對象(包括其子對象)沒有被修改(請閱讀:無副作用)。
const flattened = responses.reduce((o, g, i) => {
Object.entries(g).reduce((t, [k, v]) => {
t[`${k}${i + 1}`] = v;
return t;
}, o);
return o;
},
{});
完整的工作示例:
const orig = { "slug": "18-AZ-Gov-GE-DvF", "name": "2018 Arizona Gubernatorial GE", "tags": [], "charts": [], "election_date": "2018-11-06", "n_polls": 1, "created_at": "2017-06-13T13:32:26.000Z", "responses": [{ "label": "Ducey", "name": "Doug Ducey", "party": "Republican", "incumbent": true }, { "label": "Farley", "name": "Steve Farley", "party": "Democrat", "incumbent": false }, { "label": "Other", "name": "Other", "party": null, "incumbent": false }, { "label": "Undecided", "name": "Undecided", "party": null, "incumbent": false } ] }; const {responses, ...foo} = orig; const flattened = responses.reduce((o, g, i) => { Object.entries(g).reduce((t, [k, v]) => { t[`${k}${i + 1}`] = v; return t; }, o); return o; }, {}); console.log({flattened: {...foo, ...flattened}}); console.log({orig});
您可以分解響應和其他數據,然后使用索引鍵將每個響應減少到一個對象,然后通過對象散布運算符組合結果:
const { responses, ...other } = data
const indexedResponses = responses.reduce((acc, r, i) => {
Object.entries(r).forEach(([key, value]) => {
acc[`${key}${i + 1}`] = value
})
return acc
}, {})
const result = { ...other, ...indexedResponses }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.