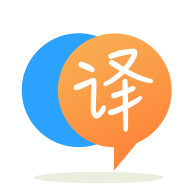
[英]Flutter - how to remove default padding (48 px as per doc) from widgets (IconButton, CheckBox, FlatButton)
[英]How do I remove Flutter IconButton big padding?
我想要一排 IconButton,它們都相鄰,但實際圖標和 IconButton 限制之間似乎有很大的填充。 我已經將按鈕上的填充設置為 0。
這是我的組件,非常簡單:
class ActionButtons extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
color: Colors.lightBlue,
margin: const EdgeInsets.all(0.0),
padding: const EdgeInsets.all(0.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
IconButton(
icon: new Icon(ScanrIcons.reg),
alignment: Alignment.center,
padding: new EdgeInsets.all(0.0),
onPressed: () {},
),
IconButton(
icon: new Icon(Icons.volume_up),
alignment: Alignment.center,
padding: new EdgeInsets.all(0.0),
onPressed: () {},
)
],
),
);
}
}
我想擺脫大部分淺藍色空間,讓我的圖標在左側更早開始,並且彼此更接近,但我找不到調整 IconButton 本身大小的方法。
我幾乎可以肯定這個空間是由按鈕本身占用的,因為如果我將它們的對齊方式更改為centerRight
和centerLeft
它們看起來像這樣:
使實際圖標變小也無濟於事,按鈕仍然很大:
謝謝您的幫助
只需將一個空的BoxConstrains
傳遞給constraints
屬性並填充零。
IconButton(
padding: EdgeInsets.zero,
constraints: BoxConstraints(),
)
您必須傳遞空約束,因為默認情況下 IconButton 小部件假定最小大小為 48px。
解決此問題的兩種方法。
將 IconButton 包裹在具有寬度的 Container 內。
例如:
Container(
padding: const EdgeInsets.all(0.0),
width: 30.0, // you can adjust the width as you need
child: IconButton(
),
),
您還可以使用 GestureDetector 代替 IconButton,這是 Shyju Madathil 推薦的。
GestureDetector( onTap: () {}, child: Icon(Icons.volume_up) )
將IconButton
包裝在容器中根本行不通,而是使用ClipRRect並添加一個帶有 Inkwell 的材質 Widget,只需確保為ClipRRect
小部件提供足夠的邊框半徑😉。
ClipRRect(
borderRadius: BorderRadius.circular(50),
child : Material(
child : InkWell(
child : Padding(
padding : const EdgeInsets.all(5),
child : Icon(
Icons.favorite_border,
),
),
onTap : () {},
),
),
)
這是擺脫任何額外填充的解決方案,使用InkWell
代替IconButton
:
Widget backButtonContainer = InkWell(
child: Container(
child: const Icon(
Icons.arrow_upward,
color: Colors.white,
size: 35.0,
),
),
onTap: () {
Navigator.of(_context).pop();
});
您可以簡單地使用 Icon 並將其與 GestureDetector 或 InkWell 包裝在一起,而不是刪除 IconButton 周圍的填充
GestureDetector(
ontap:(){}
child:Icon(...)
);
如果你想要漣漪/墨水飛濺效果,因為 IconButton 在點擊時提供,用 InkWell 包裹它
InkWell(
splashColor: Colors.red,
child:Icon(...)
ontap:(){}
)
盡管第二種方法中在 Icon 上拋出的 Ink 不會像 IconButton 那樣准確,但您可能需要為此做一些自定義實現。
我在嘗試在用戶觸摸屏幕的位置呈現圖標時遇到了類似的問題。 不幸的是, Icon
類將您選擇的圖標包裝在SizedBox
。
閱讀一些 Icon 類源代碼,結果發現每個 Icon 都可以被視為文本:
Widget iconWidget = RichText(
overflow: TextOverflow.visible,
textDirection: textDirection,
text: TextSpan(
text: String.fromCharCode(icon.codePoint),
style: TextStyle(
inherit: false,
color: iconColor,
fontSize: iconSize,
fontFamily: icon.fontFamily,
package: icon.fontPackage,
),
),
);
因此,例如,如果我想渲染Icons.details
以指示我的用戶剛剛指向的位置,而沒有任何邊距,我可以執行以下操作:
Widget _pointer = Text(
String.fromCharCode(Icons.details.codePoint),
style: TextStyle(
fontFamily: Icons.details.fontFamily,
package: Icons.details.fontPackage,
fontSize: 24.0,
color: Colors.black
),
);
Dart/Flutter 源代碼非常平易近人,我強烈建議您深入研究一下!
更好的解決方案是像這樣使用Transform.scale
:
Transform.scale(
scale: 0.5, // set your value here
child: IconButton(icon: Icon(Icons.smartphone), onPressed: () {}),
)
您可以使用 ListTile 它為您提供適合您需要的文本和圖標之間的默認空間
ListTile(
leading: Icon(Icons.add), //Here Is The Icon You Want To Use
title: Text('GFG title',textScaleFactor: 1.5,), //Here Is The Text Also
trailing: Icon(Icons.done),
),
要顯示飛濺效果(波紋),請使用InkResponse
:
InkResponse(
Icon(Icons.volume_up),
onTap: ...,
)
如果需要,更改圖標大小或添加填充:
InkResponse(
child: Padding(
padding: ...,
child: Icon(Icons.volume_up, size: ...),
),
onTap: ...,
)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.