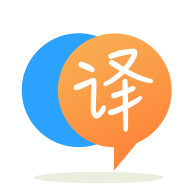
[英]How to place elements proportionally in the HBox/VBox using javafx/fxml
[英]How to align HBox by using BorderPane alignment in FXML?
我在嘗試將HBox按鈕對齊到底部的對話框中心。 我想在fxml中執行此操作。但是,BorderPane對齊在標簽中工作。 這是我方的代碼。我認為即使標簽是Bottom,BorderPane.alignment =“BOTTOM_CENTER”也必須正常工作。
班級檔案:
package application;
import java.io.IOException;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class HBoxDialog extends Application {
@Override
public void start(Stage primaryStage) {
try {
Parent root = FXMLLoader.load(getClass().getResource("HBoxDialog.fxml"));
primaryStage.setScene(new Scene(root, 500, 100));
primaryStage.show();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
launch(args);
}
}
FXML文件:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.text.Font?>
<?import javafx.scene.layout.BorderPane?>
<BorderPane xmlns:fx="http://javafx.com/fxml/1">
<top>
<Label text="this is dialogbox" BorderPane.alignment="TOP_CENTER"/>
<font>
<Font size="35"/>
</font>
</top>
<bottom>
<HBox spacing="10">
<Button text="Okay" prefWidth="90" BorderPane.alignment="BOTTOM_CENTER"/>
<Button text="Cancel" prefWidth="90" BorderPane.alignment="BOTTOM_CENTER"/>
<Button text="Help" prefWidth="90" BorderPane.alignment="BASELINE_RIGHT"/>
</HBox>
</bottom>
</BorderPane>
BorderPane.alignment
靜態屬性僅對父級為BorderPane
節點有意義。 FXML文件中定義的Button
有一個HBox
作為父項,因此在按鈕上設置BorderPane.alignment
屬性將不起作用。
可以實現通過居中內的按鈕所需的效果HBox
,簡單地通過使用alignment
的的屬性HBox
(其定位所述HBox
其范圍內的子節點):
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.text.Font?>
<?import javafx.scene.layout.BorderPane?>
<BorderPane xmlns:fx="http://javafx.com/fxml/1">
<top>
<Label text="this is dialogbox"
BorderPane.alignment="TOP_CENTER" />
<font>
<Font size="35" />
</font>
</top>
<bottom>
<HBox spacing="10" alignment="CENTER">
<Button text="Okay" prefWidth="90" />
<Button text="Cancel" prefWidth="90" />
<Button text="Help" prefWidth="90" />
</HBox>
</bottom>
</BorderPane>
這給了
Label
需要BorderPane.alignment="CENTER"
但HBox
需要alignment="CENTER"
的原因是因為它們具有不同的可調整范圍 ,特別是它們的最大寬度不同。 默認情況下,標簽的最大寬度是其首選寬度,而HBox
的最大寬度是無限大。 你可以通過在它們上面設置背景顏色來看到這一點:
<Label text="this is dialogbox"
BorderPane.alignment="TOP_CENTER"
style="-fx-background-color: aquamarine;"/>
<!-- ... -->
<HBox spacing="10" alignment="CENTER"
style="-fx-background-color: lightskyblue;">
alignment
屬性將節點的內容定位在其邊界內。 由於標簽在其文本邊界內沒有額外空間,因此使用默認設置時, alignment
屬性將不起作用。 另一方面,標簽不如邊框窗格的頂部區域寬,因此有空間將其定位在該區域內。 BorderPane.alignment="CENTER"
屬性將整個標簽置於邊框窗格的頂部區域中。
相比之下, HBox
本身已經填充了邊框窗格底部區域的整個寬度。 因此,在該區域內沒有額外的空間來對齊它,因此對於HBox
,設置BorderPane.alignment="CENTER"
將不起作用。 在另一方面,存在越多的空間HBox
本身比所需的按鈕,所以按鈕(的內容HBox
)可以在范圍內排列HBox
自身使用alignment="CENTER"
的財產HBox
。
如果需要,可以更改最大寬度以達到相同的效果。 例如:
<?xml version="1.0" encoding="UTF-8"?>
<?import java.lang.Double?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.text.Font?>
<?import javafx.scene.layout.BorderPane?>
<BorderPane xmlns:fx="http://javafx.com/fxml/1">
<top>
<Label text="this is dialogbox"
alignment="CENTER"
style="-fx-background-color: aquamarine;">
<maxWidth>
<Double fx:constant="MAX_VALUE"/>
</maxWidth>
</Label>
<font>
<Font size="35" />
</font>
</top>
<bottom>
<HBox spacing="10" alignment="CENTER"
style="-fx-background-color: lightskyblue;">
<Button text="Okay" prefWidth="90" />
<Button text="Cancel" prefWidth="90" />
<Button text="Help" prefWidth="90" />
</HBox>
</bottom>
</BorderPane>
允許標簽增長(就像HBox
的默認值),所以現在它的alignment
屬性具有所需的效果:
要么
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.text.Font?>
<?import javafx.scene.layout.BorderPane?>
<?import javafx.scene.layout.Region?>
<BorderPane xmlns:fx="http://javafx.com/fxml/1">
<top>
<Label text="this is dialogbox"
BorderPane.alignment="CENTER"
style="-fx-background-color: aquamarine;" />
<font>
<Font size="35" />
</font>
</top>
<bottom>
<HBox spacing="10" BorderPane.alignment="CENTER"
style="-fx-background-color: lightskyblue;">
<maxWidth>
<Region fx:constant="USE_PREF_SIZE" />
</maxWidth>
<Button text="Okay" prefWidth="90" />
<Button text="Cancel" prefWidth="90" />
<Button text="Help" prefWidth="90" />
</HBox>
</bottom>
</BorderPane>
使HBox
表現得像按鈕,所以現在它的BorderPane.alignment
提供了所需的效果:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.