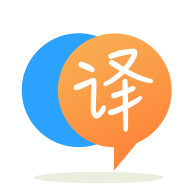
[英]How can i fix my code to put each paragraph in a new line, as I am trying to add paragraph before the given paragraph
[英]How can I add a paragraph, line break or horizontal rule tag to this code?
var userChoice = prompt('Choose between Rock,Paper or Scissors');
var computerChoiceArray = ['Rock','Paper','Scissors'];
var choiceNumber = Math.floor(Math.random()*3);
var compChoice = computerChoiceArray[choiceNumber];
document.write('Computer chose '+ compChoice)
var game = function(compChoice,userChoice) {
if (compChoice === userChoice) {
document.write("The result is a Tie!!")
}else if( compChoice === "Rock " && userChoice === "Scissors") {
document.write("Computer wins !!!")
}else if( compChoice === "Rock " && userChoice === "Paper") {
document.write("You won !!!")
}else if( compChoice === "Scissors" && userChoice === "Rock") {
document.write ("You won !!!")
}else if( compChoice === "Scissors" && userChoice === "Paper") {
document.write("Computer wins !!!")
}else if( compChoice === "Paper" && userChoice === "Rock") {
document.write("Computer wins !!!")
}else if( compChoice === "Paper" && userChoice === "Scissors") {
document.write ("You won !!!")
}else{
alert ("Error, "+userChoice+" is not applicable ");
}
}
game(compChoice,userChoice);
即使添加了所需的標簽之后,仍然存在錯誤。
請添加任何用於保留行或div標簽的標簽。 如果可能,請提出一些改進建議。
您的代碼最終出現在錯誤警報中的原因很可能是由於在比較文本時您在文本上添加了空格,例如if(userChoice === 'Rock ')
以及您忽略了JavaScript是區分大小寫。 含義Rock !== 'rock'
我將盡可能多地使用您的代碼,以確保它仍然是您的代碼,但是請注意,比起比較字符串值,還有許多更好的方法來編寫此代碼。
使用toUpperCase()
允許您比較字符串,無論用戶鍵入rock
還是rOcK
您可能還可以添加trim()
因為用戶可以選擇帶有空格的Rock
。 考慮將用戶選擇更改為下拉菜單以消除該問題。
關於換行符,因為您沒有指定確切的位置,所以我假設您的意思是在計算機選擇的內容和結果之間。
您可以通過添加<br/>
來添加換行符,例如, document.write("<br/>Computer wins !!!")
請注意,正如其他人所說,使用
document.write
不好,請嘗試使用document.createElement()
代替。 另外,請考慮將用戶選擇更改為下拉菜單,以消除字符串問題。 您可以比比較字符串更好地驗證選擇的數字表示形式。 不過,我將這項研究留給您。
無論如何,下面的代碼盡可能地接近您的代碼,並進行最少的更改,以使其符合您的預期結果並修復錯誤。
var userChoice = prompt('Choose between Rock,Paper or Scissors'); var computerChoiceArray = ['Rock', 'Paper', 'Scissors']; var choiceNumber = Math.floor(Math.random() * 3); var compChoice = computerChoiceArray[choiceNumber]; document.write('Computer chose ' + compChoice); var game = function(compChoice, userChoice) { if (compChoice.toUpperCase() === userChoice.toUpperCase()) { document.write("<br/>The result is a Tie!!") } else if (compChoice.toUpperCase() === "ROCK" && userChoice.toUpperCase() === "SCISSORS") { document.write("<br/>Computer wins !!!") } else if (compChoice.toUpperCase() === "ROCK" && userChoice.toUpperCase() === "PAPER") { document.write("<br/>You won !!!") } else if (compChoice.toUpperCase() === "SCISSORS" && userChoice.toUpperCase() === "ROCK") { document.write("<br/>You won !!!") } else if (compChoice.toUpperCase() === "SCISSORS" && userChoice.toUpperCase() === "PAPER") { document.write("<br/>Computer wins !!!") } else if (compChoice.toUpperCase() === "PAPER" && userChoice.toUpperCase() === "ROCK") { document.write("<br/>Computer wins !!!") } else if (compChoice.toUpperCase() === "PAPER" && userChoice.toUpperCase() === "SCISSORS") { document.write("<br/>You won !!!") } else { alert("Error, " + userChoice + " is not applicable "); } } game(compChoice, userChoice);
我已經更新了您的代碼。 我要說明的主要事情是,您必須將代碼拆分為邏輯部分。 在我的示例中有:
game
round
,開始新的回合並編寫游戲日志 writeLogs
顯示進度。 請注意,這不是實現此游戲的最佳方法。
var computerChoiceArray = ['rock', 'paper', 'scissors']; var MAX_NUMBER_OF_ROUNDS = 3; var game = { DOMElement: document.getElementById('game-wrapper'), roundsCounter: 0, logs: [] }; function round(game) { if (game.roundsCounter >= MAX_NUMBER_OF_ROUNDS) { game.logs.push("The End"); writeLogs(game); return; } var userChoice = prompt('Choose between: ' + computerChoiceArray.join(', ')) || ''; userChoice = userChoice.toLowerCase(); var compChoiceNumber = Math.floor(Math.random() * 3); var compChoice = computerChoiceArray[compChoiceNumber]; if (compChoice === userChoice) { game.logs.push("The result is a Tie!!"); } else if (compChoice === "rock" && userChoice === "scissors") { game.logs.push("Computer wins !!!"); } else if (compChoice === "rock" && userChoice === "paper") { game.logs.push("You won !!!"); } else if (compChoice === "scissors" && userChoice === "rock") { game.logs.push("You won !!!"); } else if (compChoice === "scissors" && userChoice === "paper") { game.logs.push("Computer wins !!!"); } else if (compChoice === "paper" && userChoice === "rock") { game.logs.push("Computer wins !!!"); } else if (compChoice === "paper" && userChoice === "scissors") { game.logs.push("You won !!!"); } else { game.logs.push("Error, " + userChoice + " is not applicable "); } game.roundsCounter += 1; writeLogs(game); // wait a sec to write logs and start a new round setTimeout(function() { round(game); }, 1000) } function writeLogs(game) { // clear logs game.DOMElement.innerHTML = ''; for (var log of game.logs) { var logHTMLElement = document.createElement('div'); logHTMLElement.innerHTML = log; game.DOMElement.appendChild(logHTMLElement); } } // start the first round round(game);
<div id="game-wrapper"></div>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.