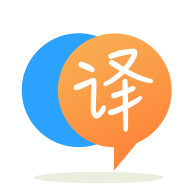
[英]AngularJS: display a select box from JSON data retrieved by http GET (from REST service)
[英]Display data in return() from JSON retrieved render()
所以我在顯示從API檢索到的數據作為JSON時遇到問題。 下面是代碼。 當我嘗試在render函數中顯示值時,由於未定義,所以不會顯示。
import React, { Component } from 'react';
class Test extends Component {
render() {
var request = require("request");
var urlCardName = encodeURIComponent(this.props.card.card_name);
var url = "./query.php?cardName=" + urlCardName;
request({
url: url,
json: true
}, function (error, response, body) {
if (!error && response.statusCode === 200) {
var cardResponse = JSON.stringify(body);
var cardName = body.cardName.toString();
var cardCount = body.cardCount.toString();
console.log(cardCount);
}
})
return(<div>
Card Count: {cardCount}
Card Name: {cardName}
</div>);
}
}
export default Test;
問題是我不完全了解如何從JSON中獲取變量並將其顯示為render函數中的字符串。
如何顯示這些值?
謝謝!
1-您將需要將api中的數據保存到組件狀態,並且在更新組件狀態后做出反應,組件將再次呈現,您將能夠看到您的數據。
最好閱讀更多有關React和組件狀態的信息
2-您需要將請求調用移至componentDidMount
生命周期函數,該函數將在組件安裝后直接調用,並且您可以在此函數內更新組件狀態,避免在render函數內更新狀態也很重要,因為最終將導致無限次的渲染調用
閱讀更多有關組件生命周期的信息也很好
最后,您可以嘗試以下操作:
import React, { Component } from 'react';
class Test extends Component {
constructor(props) {
super(props);
this.state = {
cardName: '',
cardCount: '',
};
}
componentDidMount() {
var request = require("request");
var urlCardName = encodeURIComponent(this.props.card.card_name);
var url = "./query.php?cardName=" + urlCardName;
request({
url: url,
json: true
}, function (error, response, body) {
if (!error && response.statusCode === 200) {
var cardResponse = JSON.stringify(body);
this.setState( cardName: body.cardName.toString());
this.setState( cardCount: body.cardCount.toString());
}
})
}
render() {
return(<div>
Card Count: {this.state.cardCount}
Card Name: {this.state.cardName}
</div>);
}
}
export default Test;
按照標准,您將AJAX請求放入componentDidMount
並從那里更新狀態。
import React, {Component} from 'react';
import request from 'request';
class Test extends Component {
constructor(props) {
super(props);
this.state = {
data: null // empty data
}
}
componentDidMount(){
var urlCardName = encodeURIComponent(this.props.card.card_name);
var url = "./query.php?cardName=" + urlCardName;
request({
url: url,
json: true
}, (error, response, body) => {
if (!error && response.statusCode === 200) {
var cardResponse = JSON.stringify(body);
var cardName = body.cardName.toString();
var cardCount = body.cardCount.toString();
// Update the state
this.setState( { cardName, cardCount } );
}
})
}
render() {
// Object destructing
let { cardName, cardCount } = this.state;
return (
<div>
Card Count: {cardCount}
Card Name: {cardName}
</div>
);
}
}
export default Test;
這取決於。 如果您不希望這些數據存在於其自身的組件中(例如<CardCount />
),則可以將cardCount
包裝在<h1>
標記中,例如: <h1>{cardCount}</h1>
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.