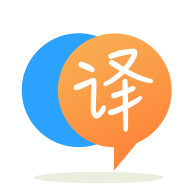
[英]What is the best way to implement a generic range as a function argument in Java?
[英]What is the best way to implement the python count function in java?
我正在學習如何在Java中使用流,並且我想知道將python count功能復制到Java中的最有效方法。
對於不熟悉python計數的人,請參見此處 。
我已經完成了一個簡單的實現,但是我懷疑這是否會添加到生產級環境中:
private List<String> countMessages(List<String> messages) {
Map<String, Integer> messageOccurrences = new HashMap<>();
List<String> stackedMessages = new LinkedList<String>();
this.messages.stream().filter((message) -> (messageOccurrences.containsKey(message))).forEachOrdered((message) -> {
int new_occ = messageOccurrences.get(message) + 1;
messageOccurrences.put(message, new_occ);
});
messageOccurrences.keySet().forEach((key) -> {
stackedMessages.add(key + "(" + messageOccurrences.get(key) + "times)" );
});
return stackedMessages;
}
任何改進或指針將不勝感激。
要回答“在Java中實現python count函數的最佳方法是什么?”的問題。
Java已經有Collections.frequency可以做到這一點。
但是,如果您想使用流API來做到這一點,那么我相信通用解決方案將是:
public static <T> long count(Collection<T> source, T element) {
return source.stream().filter(e -> Objects.equals(e, element)).count();
}
那么用例將是:
long countHellp = count(myStringList, "hello");
long countJohn = count(peopleList, new Person("John"));
long count101 = count(integerList, 101);
...
...
或者,如果您願意,甚至可以傳遞謂詞:
public static <T> long count(Collection<T> source, Predicate<? super T> predicate) {
return source.stream().filter(predicate).count();
}
然后,用例將是:
long stringsGreaterThanTen = count(myStringList, s -> s.length() > 10);
long malesCount = count(peopleList, Person::isMale);
long evens = count(integerList, i -> i % 2 == 0);
...
...
鑒於您對帖子的評論,您似乎想對它進行“分組”並獲得每個分組的計數。
public Map<String, Long> countMessages(List<String> messages) {
return messages.stream()
.collect(groupingBy(Function.identity(), counting()));
}
這將從messages
列表中創建一個流,然后對其進行分組,並傳遞counting()
作為下游收集器,這意味着我們將檢索Map<String, Long>
,其中鍵是元素,值是該特定字符串的出現。
確保您已導入:
import static java.util.stream.Collectors.*;
對於后一種解決方案。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.