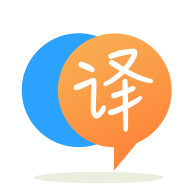
[英]How to convert two HashMap into single JSON array using node JS
[英]Convert js Array to Dictionary/Hashmap
我正在嘗試將對象數組轉換為哈希圖。 我只有 ES6 的某些部分可用,我也不能使用Map
。
數組中的對象非常簡單,例如{nation: {name: string, iso: string, scoringPoints: number}
。 我需要通過scoringPoints
對它們進行排序。 我現在想要一個按 iso -> {[iso:string]:number}
排序的“字典”。
我已經嘗試過(從這里 (SO) )
const dict = sortedData.reduce((prev, curr, index, array) => (
{ ...array, [curr.nation.iso]: ++index }
), {});
但dict
原來是一個索引以0
開頭的Object
。 希望只是我沒有看到的一件小事。 但目前我的腦子在思考如何將一個簡單的數組轉換成一個類似 hashmap 的對象。 也許Array.map
?
我還應該注意,我使用的是TypeScript
,在輸入不正確之前我也遇到了一些問題。
const test = [
{ nation: { name: "Germany", iso: "DE", rankingPoints: 293949 } },
{ nation: { name: "Hungary", iso: "HU", rankingPoints: 564161 } },
{ nation: { name: "Serbia", iso: "SR", rankingPoints: 231651 } }
];
const sorted = test.sort((a, b) => a.nation.rankingPoints - b.nation.rankingPoints);
const dict = sorted.reduce((prev, curr, index, array) => ({ ...array, [curr.nation.iso]: ++index }), {});
console.log(JSON.stringify(dict));
正在顯示
{
"0": {
"nation": {
"name": "Serbia",
"iso": "RS",
"rankingPoints": 231651
}
},
"1": {
"nation": {
"name": "Germany",
"iso": "DE",
"rankingPoints": 293949
}
},
"2": {
"nation": {
"name": "Hungary",
"iso": "HU",
"rankingPoints": 564161
}
},
"HU": 3
}
在控制台中。
根據評論,我想要的是一個類似哈希圖的對象
{
"HU": 1,
"DE": 2,
"RS": 3
}
其中屬性值是排序數據中的排名(+1),因此我可以通過訪問dict["DE"]
來簡單地獲得排名,這將返回2
。
使用forEach
或reduce
捕獲數據中每個鍵的位置:
const test = [ { nation: { name: "Germany", iso: "DE", rankingPoints: 293949 } }, { nation: { name: "Hungary", iso: "HU", rankingPoints: 564161 } }, { nation: { name: "Serbia", iso: "SR", rankingPoints: 231651 } } ]; const sorted = test.sort((a, b) => a.nation.rankingPoints - b.nation.rankingPoints); // Using forEach: var dict = {} sorted.forEach((el, index) => dict[el.nation.iso] = sorted.length - index); // Using reduce: dict = sorted.reduce( (dict, el, index) => (dict[el.nation.iso] = sorted.length - index, dict), {} ); console.log(dict) console.log("dict['DE'] = ", dict['DE'])
輸出:
{
"SR": 3,
"DE": 2,
"HU": 1
}
dict['DE'] = 2
(請注意,在用作映射的對象中,屬性的順序並不重要 - 如果您需要特定的順序,請使用數組。)
const test = [ { nation: { name: "Germany", iso: "DE", rankingPoints: 293949 } }, { nation: { name: "Hungary", iso: "HU", rankingPoints: 564161 } }, { nation: { name: "Serbia", iso: "SR", rankingPoints: 231651 } } ]; const sorted = test.sort((a, b) => b.nation.rankingPoints - a.nation.rankingPoints); const dict = sorted.reduce((result, curr, index, array) => ({ ...result, [curr.nation.iso]: ++index }), {}); console.log(JSON.stringify(dict));
也可以使用 Array.map 和 Object.fromEntries 來實現:
const test = [ { nation: { name: "Germany", iso: "DE", rankingPoints: 293949 } }, { nation: { name: "Hungary", iso: "HU", rankingPoints: 564161 } }, { nation: { name: "Serbia", iso: "SR", rankingPoints: 231651 } } ]; const sorted = test.sort((a, b) => a.nation.rankingPoints < b.nation.rankingPoints ? 1 : (a.nation.rankingPoints > b.nation.rankingPoints ? -1 : 0)); const dict = Object.fromEntries(sorted.map((c, index) => [c.nation.iso, index + 1])); console.log(dict);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.