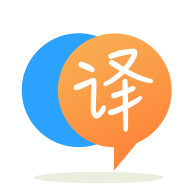
[英]printing out objects within objects stored in different arrays using toString (java)
[英]Printing toString information out of List made of different objects
我在應對該問題方面存在一些問題。 我有一個Boat類,其中包含toString()和getter和setters。 PowerBoat類可擴展功能並從其超類重寫toString()方法。 SailBoat類擴展功能並從其超類重寫toString()方法。 在測試類中,我在Boat類型的ArrayList中添加了不同的PowerBoat,SailBoats。 我需要找到最昂貴的船,並打印有關該船的toString()信息。
public class Boat {
String color;
int length;
public Boat(){
color = "white";
length = 20;
}
public Boat(String color, int length){
setColor(color);
setLength(length);
}
public String getColor() {
return color;
}
public boolean setColor(String color) {
switch (color){
case "white" : this.color = color;
case "red" : this.color = color;
case "blue" : this.color = color;
case "yellow" : this.color = color;
return true;
}
return false;
}
public int getLength() {
return length;
}
public boolean setLength(int length) {
if(length >= 20 && length <= 50) {
this.length = length;
return true;
}
return false;
}
@Override
public String toString() {
return "Boat{" +
"color='" + color + '\'' +
", length=" + length +
'}';
}
}
public class PowerBoat extends Boat {
int engineSize;
public PowerBoat(){
super();
setEngineSize(5);
}
public PowerBoat(String color, int length, int engineSize){
super(color, length);
setEngineSize(engineSize);
}
public boolean setEngineSize(int engineSize){
if(engineSize >= 5 && engineSize <= 350){
this.engineSize = engineSize;
return true;
}
return false;
}
public int getEngineSize() {
return engineSize;
}
public int calcPrice(){
return 5000 + length + 300 + engineSize * 20;
}
@Override
public String toString() {
return super.toString() +
"engineSize= " + engineSize +
'}' + " Price " + calcPrice();
}
}
public class SailBoat extends Boat {
int numSails = 0;
public SailBoat(){
numSails = 1;
}
public SailBoat(String color, int length, int numSails){
super(color, length);
setNumSails(numSails);
}
public int getNumSails() {
return numSails;
}
public boolean setNumSails(int numSails) {
if(numSails >= 1 && numSails <= 4){
this.numSails = numSails;
return true;
}
return false;
}
public int calcPrice(){
return length * 1000 + numSails * 2000;
}
@Override
public String toString() {
return super.toString() +
"numSails= " + numSails +
'}' + " price " + calcPrice();
}
}
public class Inventory {
public static void main(String[] args){
ArrayList<Boat> list = new ArrayList();
Boat powerBoat = new PowerBoat("blue", 46, 60);
Boat powerBoat1 = new PowerBoat("yellow", 42, 55);
Boat sailBoat = new SailBoat("white", 32, 1);
Boat sailBoat1 = new SailBoat("red", 24, 2);
list.add(powerBoat);
list.add(powerBoat1);
list.add(sailBoat);
list.add(sailBoat1);
int sumSailBoat = 0;
int sumPowerBoat = 0;
int largest = Integer.MIN_VALUE;
for(Boat b : list){
if (b instanceof SailBoat){
sumSailBoat+= ((SailBoat) b).calcPrice();
if (((SailBoat) b).calcPrice() > largest){
if(b instanceof SailBoat) {
largest = ((SailBoat) b).calcPrice();
}
}
}
if (b instanceof PowerBoat){
sumPowerBoat+= ((PowerBoat) b).calcPrice();
if(((PowerBoat) b).calcPrice() > largest){
if(b instanceof PowerBoat){
largest = ((PowerBoat) b).calcPrice();
}
}
}
}
int totalSum = sumSailBoat + sumPowerBoat;
System.out.println("Total price of sail boats is " + sumSailBoat);
System.out.println("Total price of sail boats is " + sumPowerBoat);
System.out.println("Total price of sail and power boats is " + totalSum);
System.out.println("Most expensive boat is " + largest);
}
}
我設法找到了最大的價格,但是我如何打印關於那條船的toString信息?
如果您知道類型,則可以使用:
System.out.println("My PowerBoat " + ((PowerBoat) b));
System.out.println("My SailBoat " + ((SailBoat) b));
如果已經找到該對象,則需要打印該對象並將其存儲在變量中,然后只需對其調用toString。
Boat b = //whichever one you found here from the arraylist
System.out.println(b.toString())
由於java中的類使用動態綁定,因此即使您的引用是超類的,該方法的內容也應相同。
[teach-me]
您似乎對OO設計和Java方法調用有一些誤解。 請與您的老師交談或閱讀一些OO / Java書籍。 從Java的abstract
和final
概念開始-應該使您對如何調用方法以及如何使用繼承有所了解。
話雖如此,您需要做兩件事。
(1)要回答你的問題,除了int largest
,還留着Boat mostExpensiveBoat
,在同一時間更新更新largest
。 然后在最后打印mostExpensiveBoat.toString()
。
(2)您需要將calcPrice()作為Boat
的抽象方法。 然后,當您調用b.calcPrice()
,將“神奇地”調用與b
引用的對象類型匹配的方法。 無需檢查instanceof
或強制轉換。
將calcPrice()作為Boat接口的一部分會更好:
abstract class Boat {
String color;
int length;
public Boat(){
color = "white";
length = 20;
}
public Boat(String color, int length){
setColor(color);
setLength(length);
}
public String getColor() {
return color;
}
public boolean setColor(String color) {
switch (color){
case "white" : this.color = color;
case "red" : this.color = color;
case "blue" : this.color = color;
case "yellow" : this.color = color;
return true;
}
return false;
}
public int getLength() {
return length;
}
public boolean setLength(int length) {
if(length >= 20 && length <= 50) {
this.length = length;
return true;
}
return false;
}
public abstract int calcPrice();
@Override
public String toString() {
return "Boat{" +
"color='" + color + '\'' +
", length=" + length +
'}';
}
}
然后,您可以通過以下方式進行搜索:
int sumSailBoat = 0;
int sumPowerBoat = 0;
int largest = Integer.MIN_VALUE;
Boat boatWithLargestPrice = null;
for (Boat b : list) {
int boatPrice = b.calcPrice();
if (boatPrice > largest) {
largest = boatPrice;
boatWithLargestPrice = b;
}
if (b instanceof SailBoat) {
sumSailBoat += boatPrice;
} else {
sumPowerBoat += boatPrice;
}
}
int totalSum = sumSailBoat + sumPowerBoat;
System.out.println("Total price of sail boats is " + sumSailBoat);
System.out.println("Total price of power boats is " + sumPowerBoat);
System.out.println("Total price of sail and power boats is " + totalSum);
System.out.println("Most expensive boat is " + boatWithLargestPrice + " with price " + largest);
通過使Boat
類abstract
您可以要求所有Boat
都實現calcPrice
。 然后,您可以將所有Boat
都一樣。
public abstract class Boat {
final String color;
final int length;
public Boat(String color, int length) {
this.color = color;
this.length = length;
}
public abstract int calcPrice();
@Override
public String toString() {
return "Boat{" +
"color='" + color + '\'' +
", length=" + length +
'}';
}
}
public class PowerBoat extends Boat {
final int engineSize;
public PowerBoat(String color, int length, int engineSize) {
super(color, length);
this.engineSize = engineSize;
}
@Override
public int calcPrice() {
return 5000 + length + 300 + engineSize * 20;
}
@Override
public String toString() {
return super.toString() +
"engineSize= " + engineSize +
'}' + " Price " + calcPrice();
}
}
public class SailBoat extends Boat {
final int numSails;
public SailBoat(String color, int length, int numSails) {
super(color, length);
this.numSails = numSails;
}
@Override
public int calcPrice() {
return length * 1000 + numSails * 2000;
}
@Override
public String toString() {
return super.toString() +
"numSails= " + numSails +
'}' + " price " + calcPrice();
}
}
public void test() {
ArrayList<Boat> list = new ArrayList();
list.add(new PowerBoat("blue", 46, 60));
list.add(new PowerBoat("yellow", 42, 55));
list.add(new SailBoat("white", 32, 1));
list.add(new SailBoat("red", 24, 2));
Boat mostExpensiveBoat = null;
int totalSum = 0;
for (Boat boat : list) {
totalSum += boat.calcPrice();
if(mostExpensiveBoat == null || boat.calcPrice() > mostExpensiveBoat.calcPrice() ) {
mostExpensiveBoat = boat;
}
}
System.out.println("Total price of sail and power boats is " + totalSum);
System.out.println("Most expensive boat is " + mostExpensiveBoat);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.