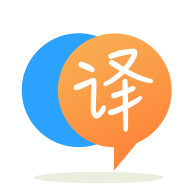
[英]JavaScript: Print as PDF with dynamic table and multiple columns and rows
[英]Searching through multiple rows and columns of a table in Javascript
我有一個包含多行和多列的表。 我寫了一個函數,目前只搜索我的表的第一列和最后一列,但我不確定為什么中間列被跳過。 我嘗試添加另一個for循環並出錯。
function function2(){ var input = document.getElementById("myInput"); var filter = input.value.toUpperCase(); var table = document.getElementById("table2c"); var tr = table.getElementsByTagName("tr"); var td, tdArr, i, j; for (i = 0; i < tr.length; i++) { tdArr = tr[i].getElementsByTagName("td"); for (j = 0; j < tdArr.length; j++){ td = tdArr [j]; if (td) { if (td.innerHTML.toUpperCase().indexOf(filter) > -1 ) { tr[i].style.display = ""; } else { tr[i].style.display = "none"; } } } } }
This is my table: <table className="table table-bordered" id="table2c"> <tbody> <tr> <th> Header Name: </th> <th> Description: </th> <th> Value: </th> </tr> <tr> <td> Authorization </td> <td> security token </td> <td> To be supplied </td> </tr> <tr> <td> Content-Type </td> <td> body of the request </td> <td> applicaiton </td> </tr> <tr> <td> API-KEY </td> <td> ID </td> <td> To be supplied </td> </tr> <tr> <td> correlation </td> <td> Unique identifier </td> <td> eg control number </td> </tr> <tr> <td> name </td> <td> system name </td> <td> One of: </td> </tr> </tbody> </table>
加分; 如果找到匹配則存在for循環。
function function2() { var input = document.getElementById("myInput"); var filter = input.value.toUpperCase(); var table = document.getElementById("table2c"); var tr = table.getElementsByTagName("tr"); var td, tdArr, i, j; for (i = 0; i < tr.length; i++) { tdArr = tr[i].getElementsByTagName("td"); for (j = 0; j < tdArr.length; j++) { td = tdArr[j]; if (td) { if (td.innerHTML.toUpperCase().indexOf(filter) > -1) { tr[i].style.display = ""; break; } else { tr[i].style.display = "none"; } } } } }
<input type="text" id="myInput" /> <input type="button" id="search" value="Search" onclick="function2()" /> <table className="table table-bordered" id="table2c"> <tbody> <tr> <th> Header Name: </th> <th> Description: </th> <th> Value: </th> </tr> <tr> <td> Authorization </td> <td> HMAC security token </td> <td> To be supplied by APIGEE </td> </tr> <tr> <td> Content-Type </td> <td> Media type of the body of the request </td> <td> applicaiton/xml </td> </tr> <tr> <td> X-AMEX-API-KEY </td> <td> HMAC ID </td> <td> To be supplied by APIGEE </td> </tr> <tr> <td> correlation_id </td> <td> Unique identifier to track the consumer request </td> <td> eg Process control number </td> </tr> <tr> <td> originator_name </td> <td> Originating consumer system name </td> <td> One of: GCAP, GDE </td> </tr> </tbody> </table>
你可以從第二行循環,因為一個發現會消失表頭。 然后,您可以提前關閉所有行,如果找到的值在行中,則刪除'none'
。 然后打破。
function function2() { var input = document.getElementById("myInput"), filter = input.value.toUpperCase(), table = document.getElementById("table2c"), tr = table.getElementsByTagName("tr"), td, tdArr, i, j; for (i = 1; i < tr.length; i++) { tr[i].style.display = "none"; tdArr = tr[i].getElementsByTagName("td"); for (j = 0; j < tdArr.length; j++) { td = tdArr[j]; if (td && td.innerHTML.toUpperCase().indexOf(filter) > -1) { tr[i].style.display = ""; break; } } } }
<input id="myInput" type="text" onchange="function2()"> <table className="table table-bordered" id="table2c"> <tbody> <tr><th> Header Name: </th><th> Description: </th><th> Value: </th></tr> <tr><td> Authorization </td><td> HMAC security token </td><td> To be supplied by APIGEE </td></tr> <tr><td> Content-Type </td><td> Media type of the body of the request </td><td> applicaiton/xml </td></tr> <tr><td> X-AMEX-API-KEY </td><td> HMAC ID </td><td> To be supplied by APIGEE </td></tr> <tr><td> correlation_id </td><td> Unique identifier to track the consumer request </td><td> eg Process control number </td></tr> <tr><td> originator_name </td><td> Originating consumer system name </td><td> One of: GCAP, GDE </td></tr> </tbody> </table>
如果(td.innerHTML.toUpperCase()。indexOf(filter)> -1){tr [i] .style.display =“”; 打破; 一旦找到結果,你需要停止第二個循環,否則如果在第三列中找不到該元素,它將隱藏整行
function function2(){ var input = document.getElementById("myInput"); var filter = input.value.toUpperCase(); var table = document.getElementById("table2c"); var tr = table.getElementsByTagName("tr"); var td, tdArr, i, j; for (i = 0; i < tr.length; i++) { tdArr = tr[i].getElementsByTagName("td"); for (j = 0; j < tdArr.length; j++){ td = tdArr [j]; if (td) { if (td.innerHTML.toUpperCase().indexOf(filter) > -1 ) { tr[i].style.display = ""; break; } else { tr[i].style.display = "none"; } } } } }
<input type="text" id="myInput" /> <button onclick="function2()">search</button> This is my table: <table className="table table-bordered" id="table2c"> <tbody> <tr> <th> Header Name: </th> <th> Description: </th> <th> Value: </th> </tr> <tr> <td> Authorization </td> <td> security token </td> <td> To be supplied </td> </tr> <tr> <td> Content-Type </td> <td> body of the request </td> <td> applicaiton </td> </tr> <tr> <td> API-KEY </td> <td> ID </td> <td> To be supplied </td> </tr> <tr> <td> correlation </td> <td> Unique identifier </td> <td> eg control number </td> </tr> <tr> <td> name </td> <td> system name </td> <td> One </td> </tr> </tbody> </table>
僅供參考,這是一個使用函數方法和一些ES6特性( 箭頭函數 , 擴展語法和.reduce
方法)的實現:
const filter = () => { const input = document.querySelector('#myInput'); const filter = input.value.toUpperCase(); const table = document.querySelector('#table2c'); const trs = table.querySelectorAll('tr:not(:first-child)'); // loop through all <tr> except the first one (header) trs.forEach(tr => { // reduce all the row's content (header, description and value) to a single string) const content = [...tr.children].reduce((content, td) => content + ' ' + td.textContent, '') // search if the filter is in content if(content.toUpperCase().includes(filter)) { tr.style.display = ""; } else { tr.style.display = "none"; } }); } // add an event listener to the input element document.querySelector('#myInput').addEventListener('keyup', filter);
<input id="myInput" /> <table className="table table-bordered" id="table2c"> <tbody> <tr> <th> Header Name: </th> <th> Description: </th> <th> Value: </th> </tr> <tr> <td> Authorization </td> <td> HMAC security token </td> <td> To be supplied by APIGEE </td> </tr> <tr> <td> Content-Type </td> <td> Media type of the body of the request </td> <td> applicaiton/xml </td> </tr> <tr> <td> X-AMEX-API-KEY </td> <td> HMAC ID </td> <td> To be supplied by APIGEE </td> </tr> <tr> <td> correlation_id </td> <td> Unique identifier to track the consumer request </td> <td> eg Process control number </td> </tr> <tr> <td> originator_name </td> <td> Originating consumer system name </td> <td> One of: GCAP, GDE </td> </tr> </tbody> </table>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.