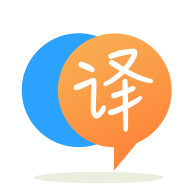
[英]In Javascript, is Array.join really Array.prototype.join?
[英]Map or flatmap that works like Array.prototype.join in Javascript
我發現Array.prototype.join
的功能非常有用,因為它僅將聯接值應用於數組元素的“內部”連接。 像這樣:
['Hey', 'there'].join('-') // Hey-there
在此示例中, Array.protoype.map
產生“剩余”破折號:
['Hey', 'there'].map(value => value + '-') // Hey-there-
我一直在尋找一種簡潔的方式來映射數組,而不將其轉換為字符串,可能轉換為新數組,如下所示:
// Intended behaviour ['Hey', 'there'].mapJoin('-') // ['Hey', '-', 'there']
我並不是在尋找一種當務之急的解決方案,因為我可以自己寫一個,然后將其放在全局中。 我正在尋找一種本機表達方式(ES6很好)來優雅地表達它,以便可以在我的所有項目中編寫它。
您可以使用所需的分隔符進行連接,並用加號逗號分隔(或其他任何值,如果用於連接)。
var array = ['Hey', 'there'], separator = '-', result = array.join(',' + separator + ',').split(','); console.log(result);
另一個解決方案是采用新索引,並用分隔符填充previos索引。
var array = ['Hey', 'there'], separator = '-', result = Object.assign( [], ...array.map((v, i) => ({ [i * 2 - 1]: separator, [i * 2]: v })) ); console.log(result);
它不完全漂亮或優雅,但是
['Hey', 'there'].reduce(
(acc, value, i, arr) => (acc.push(value), i < arr.length - 1 ? acc.push('-') : 0, acc),
[],
)
@ AKX答案的替代版本。
const data = ['Hey', 'there']; const output = data.reduce((p, c, i, a) => p.concat(i < a.length -1 ? [c, '-'] : [c]), []); console.log(output)
你可以試試這個
const mapJoin = (arr, joiner) => { return arr.reduce( (curr, t) => curr.concat(t, joiner), []).slice(0, arr.length*2-1) } const data = ["Hey", "There"] console.log(mapJoin(data, "-"))
一個簡單的遞歸編碼
const intersperse = (sep, [ x, ...rest ]) => // base case; return empty result x === undefined ? [] // one remaining x, return singleton : rest.length === 0 ? [ x ] // default case; return pair of x and sep and recur : [ x, sep ] .concat (intersperse (sep, rest)) console.log ( intersperse ("~", []) // [] , intersperse ("~", [ 1 ]) // [ 1 ] , intersperse ("~", [ 1, 2 ]) // [ 1, ~, 2 ] , intersperse ("~", [ 1, 2, 3 ]) // [ 1, ~, 2, ~, 3 ] , intersperse ("~", [ 1, 2, 3, 4 ]) // [ 1, ~, 2, ~, 3, ~, 4 ] )
您正在尋找易於定義的intersperse
函數:
const intersperse = (x, ys) => [].concat(...ys.map(y => [x, y])).slice(1); console.log(intersperse("-", ["Hey", "there"])); // ["Hey", "-", "there"] console.log(intersperse(0, [1, 2, 3])); // [1, 0, 2, 0, 3] console.log(intersperse(0, [])); // []
或者,您可以將其分解為較小的函數:
const concat = xss => [].concat(...xss); const concatMap = (f, xs) => concat(xs.map(f)); const intersperse = (x, ys) => concatMap(y => [x, y], ys).slice(1); console.log(intersperse("-", ["Hey", "there"])); // ["Hey", "-", "there"] console.log(intersperse(0, [1, 2, 3])); // [1, 0, 2, 0, 3] console.log(intersperse(0, [])); // []
您甚至可以將它們安裝在Array.prototype
:
Object.assign(Array.prototype, { concatenate() { return [].concat(...this); }, concatMap(f) { return this.map(f).concatenate(); }, intersperse(x) { return this.concatMap(y => [x, y]).slice(1); } }); console.log(["Hey", "there"].intersperse("-")); // ["Hey", "-", "there"] console.log([1, 2, 3].intersperse(0)); // [1, 0, 2, 0, 3] console.log([].intersperse(0)); // []
在Haskell中,您可以這樣編寫:
intersperse :: a -> [a] -> [a]
intersperse x = drop 1 . concatMap (\y -> [x, y])
您能看到相似之處嗎?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.