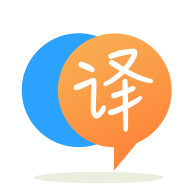
[英]CSRF Token Missing When Posting Request To DVWA Using Python Requests Library
[英]Python Request; logging in to DVWA
我正在嘗試登錄 Damn Vulnerable Web 應用程序,因為我嘗試編寫我的第一個漏洞利用程序。 然而,第一個障礙是登錄頁面。 如果沒有有效的登錄,我將無法訪問其他目錄。
我試圖分析哪些標題等我必須發送,但我似乎仍然無法使登錄工作。
POST /dvwa/login.php HTTP/1.1
Host: 192.168.26.129
User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:52.0) Gecko/20100101 Firefox/52.0
Accept: Mozilla/5.0 (X11; Linux x86_64; rv:52.0) Gecko/20100101 Firefox/52.0
Accept-Language: en-US,en;q=0.5
Accept-Encoding: gzip, deflate
Referer: http://192.168.26.129/dvwa/login.php
Cookie: security=high; PHPSESSID=c4bb8820be21ea83e6545eff0a2cb53b
Connection: close
Upgrade-Insecure-Requests: 1
Content-Type: application/x-www-form-urlencoded
Content-Length: 44
username=admin&password=password&Login=Login
當我嘗試使用瀏覽器登錄時,這是來自 burp 的全部請求。
import requests
payload = {
'username': 'admin',
'password': 'password',
'Login': 'Login'
}
headers = {
'Host': '192.168.26.129',
'User-Agent': 'Mozilla/5.0 (X11; Linux x86_64; rv:52.0) Gecko/20100101 Firefox/52.0',
'Accept': 'Mozilla/5.0 (X11; Linux x86_64; rv:52.0) Gecko/20100101 Firefox/52.0',
'Accept-Language': 'en-US,en;q=0.5',
'Referer': 'http://192.168.26.129/dvwa/login.php',
'Connection': 'close',
'Content-Type': 'application/x-www-form-urlencoded',
'Content-Length': '44'
}
cookies = dict(security='high', PHPSESSID='c4bb8820be21ea83e6545eff0a2cb53b')
with requests.Session() as c:
p = c.post('http://192.168.26.129/dvwa/login.php', headers=headers, data=payload, cookies=cookies)
print(p)
r = c.get('http://192.168.26.129/dvwa/vulnerabilities/exec')
print(r.text)
這就是我嘗試使用 python 請求登錄的方式。 我兩次得到 200 OK,但它總是返回登錄屏幕的 HTML 代碼。
有人能告訴我我的錯誤是什么嗎?
如果您有有效的 cookie,您可以將它們添加到您的會話中,
cookies = dict(security='high', PHPSESSID='o9m7jgcspe02h9rffj9g7cv0t5')
with requests.Session() as c:
c.cookies.update(cookies)
現在您可以訪問/dvwa/vulnerabilities/exec
。
如果您沒有 cookie,那么您可以使用登錄頁面來獲取它們。
在登錄表單中,您必須提交的隱藏輸入字段中有令牌。 您可以使用bs4
或re
獲取其值。
import requests
import re
payload = {
'username': 'admin',
'password': 'password',
'Login': 'Login'
}
with requests.Session() as c:
r = c.get('http://127.0.0.1/dvwa/login.php')
token = re.search("user_token'\s*value='(.*?)'", r.text).group(1)
payload['user_token'] = token
p = c.post('http://127.0.0.1/dvwa/login.php', data=payload)
r = c.get('http://127.0.0.1/dvwa/vulnerabilities/exec')
print(r.text)
我使用re
是因為它是一個標准庫,但最好使用bs4
進行 html 解析。 如果您安裝了bs4
,則可以使用此選擇器獲取令牌: 'input[name=user_token]'
。
使用 docker 時,請確保首先創建數據庫
我剛剛經歷過這個(我實際上是用 Ansible 做的,但步驟是一樣的)。 當您第一次訪問 login.php 時,它會在正文中為您提供一個 user_token,如上所述,以及 2 個 cookie 值,PHPSESSID 和安全性。 您需要提交所有這些才能登錄。然后您可以在后續請求中繼續使用 cookie 值。
所以步驟是:
上述答案之間的主要區別是您需要執行以下操作:
p = c.post('http://127.0.0.1/dvwa/login.php', cookies=cookies, data=payload)
這是一個 python 解決方案:
import requests
dvwa_ip = "192.168.1.86"
resp = requests.get(f"http://{dvwa_ip}/DVWA/login.php")
print(resp.text)
# Get the session id from cookies
phpsessid = resp.cookies.get('PHPSESSID')
# Find the html containing user_token in the page text
user_token_html = [x for x in resp.text.split('\n') if 'user_token' in x]
user_token = [x for x in user_token_html[0].split(' ') if 'value' in x]
# user_token is now ["value='2fc5744b8190bae45673e6b91b825178'"], get value using exec
value=''
exec(user_token[0])
# manually set security low as this defaults to impossible
cookies_json = {
'PHPSESSID': phpsessid,
'security': 'low'
}
body = {
'username': 'admin',
'password': 'password',
'Login': 'Login',
'user_token': value
}
post_resp = requests.post(f"http://{dvwa_ip}/DVWA/login.php", data=body, cookies=cookies_json)
print(post_resp.text) # prints out the "logged in" page (actually index.php)
print("OK") if "Welcome to Damn Vulnerable Web Application" in post_resp.text else print("ERROR")
為了完整起見,這是我的 Ansible 解決方案:
- name: "Browse to DVWA login screen to get the user_token from the content and the cookies"
uri:
url: "http://{{dvwa_ip}}/DVWA/login.php"
return_content: yes
method: GET
register: login_form
# Get the user_token from the page content - ugly but works
- set_fact:
user_token: "{{ login_form.content.split(\"name='user_token'\")[1].split('/>')[0].split('=')[1].replace(\"'\",'').replace(' ','') }}"
- name: Show user_token
debug:
var: user_token
- name: "Login to DVWA {{ dvwa_ip }} using the username, password, Login, user_token and the Cookie"
uri:
# index.php will redirect to login.php
url: "http://{{dvwa_ip}}/DVWA/login.php"
return_content: yes
follow_redirects: all
method: POST
body_format: form-urlencoded
body:
username: admin
password: password
Login: Login
user_token: "{{ user_token }}"
headers:
Cookie: "{{ login_form.cookies_string }}"
status_code: [ 200, 302 ]
register: login
failed_when: "'Welcome to Damn Vulnerable Web Application!' is not in login.content"
- name: "Do SQL Injection"
command: "sqlmap -u \"http://{{ dvwa_ip }}/DVWA/vulnerabilities/sqli_blind/?id=2&Submit=Submit#\" --cookie=\"{{ login_form.cookies_string }}\" --dbs --batch --flush-session"
ignore_errors: true
register: sqlmap_output
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.