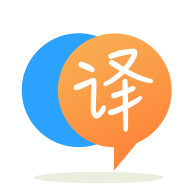
[英]Sort and print sequentially keys and values from a nested dictionary in Python
[英]How to print all the keys and values together from a nested dictionary in Python?
我仍然是Python
的新手,目前正在嘗試使用dictionaries
。 我有一個嵌套的dictionary
,看起來像:
nestedDic = {'FROM': {'SOME TEXT FROM': ['CHILD1', 'CHILD2']}}
我能夠通過迭代子節點來打印它們。
def print_nested(val, nesting = -5):
if type(val) == dict:
#print('')
nesting += 5
for k in val:
print(end='')
print(k)
print_nested(val[k],nesting)
else:
print(val)
但輸出看起來像:
但我需要的輸出是:
來自CHILD1 CHILD2的一些文字
我哪里錯了? 任何幫助都可以得到贊賞。
這個應該做你的工作:
nestedDict = {'FROM': {'SOME TEXT FROM': ['CHILD1', 'CHILD2']}}
def printDict(inDict):
print(k,end=" ")
for k,v in inDict.items():
if type(v) == dict:
printDict(v)
elif type(v) == list:
for i in v:
print(i, end=" ")
else:
print(v, end=" ")
輸出:
FROM SOME TEXT FROM CHILD1 CHILD2
而不是print(end=' '); print(k)
print(end=' '); print(k)
,你想要print(k, end=' ')
。 在調用函數時更改這樣的kwargs並不會在下次調用它時將它們設置好。
def printNestedDict(d: dict):
for k, v in d.items():
if (isinstance(v, dict)):
print(k, end = " ")
printNestedDict(v)
continue
if (any(isinstance(v, t) for t in [tuple, list])):
print(k, *v, sep = " ", end = " ")
continue
print(k, v, sep = " ", end = " ")
完全按照您的預期,檢查集合並輸出每個集合。
@Kulasangar ,你的問題很有趣也很好。 我想用遞歸來解決你的問題。
它適用於您提供的嵌套輸入字典和許多其他嵌套輸入字典,其中包含其他數據結構,如list , tuple , set , int , float等。
我想如果你將int , float , complex , long , tuple作為鍵,如果你有任何可迭代的set , list , tuple作為相應值中的項,該怎么辦?
set和dictionary是無序類型,因此最好將它們的長度限制為1個項目。 這就是為什么我將它們的長度限制為1來依次獲得最終字符串的單詞。
例如。 對於下面的INPUT字典。
nestedDic = {
('DATA', 'ANALYSIS'): {'IS': {'VERY': {'VERY': ['IMPORTANT.']}}},
4+4j: ['IS', ('A', 'Complex', 'number.')],
3.14: {
'IS': {'VALUE': {
'OF PI': {
'(A MATHEMATICAL': ['CONSTANT).']
}
}
}
},
1729: ['IS', 'KNOWN', ('AS', ('RAMANUJAN NUMBER.'))], # sets can only contain immutable values
'I AM RISHIKESH': {
'AGRAWANI.': 'A GREAT FAN',
('OF', 'PYTHON'): (' AND MATHEMATICS.', {'I WAS BORN ON'}),
1992: 'AND I LIKE THIS YEAR.'
}
}
您將期望以下字符串為OUTPUT 。
DATA ANALYSIS IS VERY VERY IMPORTANT. (4+4j) IS A Complex number. 3.14 IS VALUE OF PI (A MATHEMATICAL CONSTANT). 1729 IS KNOWN AS RAMANUJAN NUMBER. I AM RISHIKESH AGRAWANI. A GREAT FAN OF PYTHON AND MATHEMATICS. I WAS BORN ON 1992 AND I LIKE THIS YEAR.
現在,請看下面的Python代碼。 這里我有2個遞歸函數。
get_string()適用於list , tuple和set 。 如果字典的鍵是一個元組,它也可以工作。
get_string_from_nested_dict()以遞歸方式創建一個字符串。 如果它找到任何鍵作為元組,那么它調用另一個遞歸函數get_string()來獲得精確的單詞串(而不是使用元組本身)。
def get_string(l):
s = ''
for elem in l:
if type(elem) is str:
s += elem + ' '
elif type(elem) is tuple or type(elem) is list or type(elem) is set: # tuple, list, set
s += get_string(elem) + ' '
else: # int,float, complex, long
s += str(s) + ' '
return s.strip() # Remove leading or trailing white spaces
def get_string_from_nested_dict(nestedDic):
s = ''
for key in nestedDic:
if s is str:
s += key + ' '
elif type(key) is tuple:
s += get_string(key) + ' '
else: # int, complex, float, long
s += str(key) + ' '
item = nestedDic[key]
if type(item) is dict: # dict
s += get_string_from_nested_dict(nestedDic[key]) + ' '
elif type(item) is tuple or type(item) is list or type(item) is set: # list, tuple, set
s2 = get_string(item)
s += s2 + ' '
else: # int, float, comples, long
s += str(item) + ' '
return s.strip() # Remove leading or trailing white spaces
# Start
if __name__ == "__main__":
# *** TEST CASE 1 ***
nestedDic = {'FROM': {'SOME TEXT FROM': ['CHILD1', 'CHILD2']}}
s = get_string_from_nested_dict(nestedDic)
print(s, '\n') # FROM SOME TEXT FROM CHILD1 CHILD2
# *** TEST CASE 2 ***
nestedDic2 = {'HELLO,': {
'IT IS': ['VERY', {'NICE', 'PROGRAM.'}],
'PYTHON': {'IS', 'SUPER.'},
'YOU': ('CAN USE IT', 'FOR', ['DATA SCIENCE.'])
}
}
s2 = get_string_from_nested_dict(nestedDic2)
print(s2, '\n')
# *** TEST CASE 3 ***
nestedDic3 = {
('DATA', 'ANALYSIS'): {'IS': {'VERY': {'VERY': ['IMPORTANT.']}}},
4+4j: ['IS', ('A', 'Complex', 'number.')],
3.14: {
'IS': {'VALUE': {
'OF PI': {
'(A MATHEMATICAL': ['CONSTANT).']
}
}
}
},
1729: ['IS', 'KNOWN', ('AS', ('RAMANUJAN NUMBER.'))], # sets can only contain immutable values
'I AM RISHIKESH': {
'AGRAWANI.': 'A GREAT FAN',
('OF', 'PYTHON'): (' AND MATHEMATICS.', {'I WAS BORN ON'}),
1992: 'AND I LIKE THIS YEAR.'
}
}
s3 = get_string_from_nested_dict(nestedDic3)
print(s3)
這是一種可能的解決方案,但僅在像您這樣的情況下有效:
output = []
def print_nested(val):
if type(val) == dict:
for k, v in val.items():
output.append(k)
print_nested(v)
elif type(val) == list:
for k in val:
output.append(k)
print_nested(k)
nestedDic = {'FROM': {'SOME TEXT FROM': ['CHILD1', 'CHILD']}}
print_nested(nestedDic)
output = " ".join(output)
print(output)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.