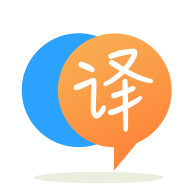
[英]Show values from a MySQL database table inside a HTML table on a webpage
[英]How to show values from a MySQL database table inside HTML via PHP?
這段代碼有效,但我想顯示數據但不使用echo,我希望index.php包含HTML來顯示它,我不想像下面的代碼那樣回顯所有內容。 這是PHP代碼:
<?php
try{
$pdo = new PDO("mysql:host=localhost;dbname=demo", "root", "");
// Set the PDO error mode to exception
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch(PDOException $e){
die("ERROR: Could not connect. " . $e->getMessage());
}
// Attempt select query execution
try{
$sql = "SELECT * FROM persons";
$result = $pdo->query($sql);
if($result->rowCount() > 0){
echo "<table>";
echo "<tr>";
echo "<th>id</th>";
echo "<th>first_name</th>";
echo "<th>last_name</th>";
echo "<th>email</th>";
echo "</tr>";
while($row = $result->fetch()){
echo "<tr>";
echo "<td>" . $row['id'] . "</td>";
echo "<td>" . $row['first_name'] . "</td>";
echo "<td>" . $row['last_name'] . "</td>";
echo "<td>" . $row['email'] . "</td>";
echo "</tr>";
}
echo "</table>";
// Free result set
unset($result);
} else{
echo "No records matching your query were found.";
}
} catch(PDOException $e){
die("ERROR: Could not able to execute $sql. " . $e->getMessage());
}
// Close connection
unset($pdo);
?>
將業務邏輯與演示文稿分開是Symfony或Laravel之類的框架做得很好的事情。
如果您不想使用其中一個框架,那么Twig是PHP的模板引擎,可能正是您想要的。
他們的文檔非常好。
https://twig.symfony.com/doc/2.x/intro.html
使用樹枝的一個簡單示例-更改路徑以適合您的系統。 這是在linux環境下進行的。
首先,安裝樹枝。 這會將樹枝下載到主目錄中的目錄呼叫供應商。 就我而言/home/john/vendor
php composer require "twig/twig:^2.0"
在public_html中創建以下內容
twig
├── bootstrap.php
├── index.php
└── templates
└── index.php
bootstrap.php
<?php
//load the autoloader from the vendor directory
require_once '/home/john/vendor/autoload.php';
//Tell twig where to look for the template files
$loader = new Twig_Loader_Filesystem('/home/john/public_html/twig/templates');
//load twig
$twig = new Twig_Environment($loader);`
index.php
<?php
require_once 'bootstrap.php';
//Your database logic could go here
//Your results. Could be from a database, but I'm using a array for simplicity
$result_set = [
[
'id' => 1,
'first_name' => 'Edmund',
'last_name' => 'Blackadder',
'email' => 'eblackadder@blackadder.com'
],
[
'id' => 2,
'first_name' => 'Baldrick',
'last_name' => 'Does he have one?',
'email' => 'dogsbody@baldrick.com'
]
];
//Render the index.php template in the templates directory, assigning the $result_set to result_set
echo $twig->render('index.php', ['result_set' => $result_set]);
template / index.php
<table>
<tr>
<th>id</th>
<th>first_name</th>
<th>last_name</th>
<th>email</th>
</tr>
{% for result in result_set %}
<tr>
<td> {{ result.id }} </td>
<td> {{ result.first_name }} </td>
<td> {{ result.last_name }} </td>
<td> {{ result.email }} </td>
</tr>
{% endfor %}
</table>
這既分離了后端,又避免了使用回聲
您將必須使用字符串方法來輸出打印或回顯,以將數據從數據庫獲取到HTML。 但是您當然可以通過幾種不同的方式將HTML與程序PHP分離。
答:您可以使用jQuery和XHR(AJAX)從PHP中提取數據,並使用jQuery .html()、. clone()、. append()等填充空HTML外殼。
B.您將數據庫結果放入一個數組,稍后可以在HTML中遍歷,例如:
// backend code
while($row = $result->fetch()){
$myData[] = $row; // makes a multi-dimensional array of your data
}
// then later in your HTML
<!DOCTYPE html>
...
<table>
<tr>
<th>id</th>
<th>first_name</th>
<th>last_name</th>
<th>email</th>
</tr>
<?php foreach($myData as $values){ ?>
<tr>
<td><?=$values['id'];?></td>
<td><?=$values['first_name'];?></td>
<td><?=$values['last_name'];?></td>
<td><?=$values['email'];?></td>
</tr>
<?php } ?>
</table>
就像其他人所說的那樣,如果不使用某些模板引擎或框架,則必須使用echo或print之類的東西。
如果您想將其保存在一個文件中並以這種方式分離邏輯,其他答案將幫助您。
不管怎么說,如果您希望將邏輯分為2個文件以進行更多清理,則可以這樣做:
mysql.php
<?php
try {
$pdo = new PDO("mysql:host=localhost;dbname=demo", "root", "");
// Set the PDO error mode to exception
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch(PDOException $e){
die("ERROR: Could not connect. " . $e->getMessage());
}
// Attempt select query execution
try{
$sql = "SELECT * FROM persons";
$result = $pdo->query($sql);
// Close connection
unset($pdo);
if ($result->rowCount() > 0){
return $result->fetchAll();
} else{
die("No records matching your query were found.");
}
} catch(PDOException $e){
die("ERROR: Could not able to execute $sql. " . $e->getMessage());
}
?>
index.php
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<table>
<thead>
<tr>
<th>id</th>
<th>first_name</th>
<th>last_name</th>
<th>email</th>
</tr>
</thead>
<tbody>
<?php $rows = (include "mysql.php"); foreach ($rows as $row) { ?>
<tr>
<td><?= $row["id"] ?></td>
<td><?= $row["first_name"] ?></td>
<td><?= $row["last_name"] ?></td>
<td><?= $row["email"] ?></td>
</tr>
<?php } ?>
</tbody>
</table>
</body>
</html>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.