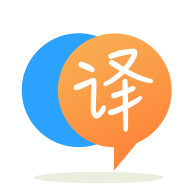
[英]How can I make this calculation function with a lot of parameters more readable?
[英]How to get a lot of if statements more readable in Python
我最近練習了python,發現自己參與了很多關於簡單剪刀石頭布游戲的if語句,看起來像這樣:我的問題是如何使我的代碼更高效,更友好地編寫和閱讀
while True:
player_choice = raw_input("\n1-Rock\n2-Paper\n3-Scissors\n{} choose a number:".format(name))
player_choice = int(player_choice)
if player_choice == 1:
player_choice = Choices.rock
if player_choice == 2:
player_choice = Choices.paper
if player_choice == 3:
player_choice = Choices.scissors
# Getting the cpu choice.
cpu_choice = random.randint(1, 3)
if cpu_choice == 1:
cpu_choice = Choices.rock
if cpu_choice == 2:
cpu_choice = Choices.paper
if cpu_choice == 3:
cpu_choice = Choices.scissors
if player_choice == cpu_choice:
print"\n Its a Tie!\n!"
if player_choice == Choices.paper and cpu_choice == Choices.rock:
print"\n Congratulations!\n{} you won!".format(name)
if player_choice == Choices.scissors and cpu_choice == Choices.paper:
print"\n Congratulations!\n{} you won!".format(name)
if player_choice == Choices.rock and cpu_choice == Choices.scissors:
print"\n Congratulations!\n{} you won!".format(name)
if cpu_choice == Choices.scissors and player_choice == Choices.paper:
print"\n Too bad!\n{} you lost!".format(name)
if cpu_choice == Choices.paper and player_choice == Choices.rock:
print"\n Too bad!\n{} you lost!".format(name)
if cpu_choice == Choices.rock and player_choice == Choices.scissors:
print"\n Too bad!\n{} you lost!".format(name)*
您的if
語句可以用字典代替。 例如,可以使用如下字典將整數映射到特定的Choices
屬性:
choices = {1: Choices.rock, 2: Choices.paper, 3: Choices.scissors}
現在您可以使用
player_choice = choices[player_choice]
和
cpu_choice = random.choice(choices.values())
從封裝的角度來看,處理此映射實際上應該是Choices
對象的責任。 如果要使用實際的enum.Enum
對象 (需要Python 3或enum34
backport軟件包的安裝),則可以使用:
player_choice = Choices(player_choice)
但是根據您定義Choices
,您可以給它一個__call__
方法,該方法基本上使用上述映射為您提供相同的結果。
接下來,您可以使用字典來確定獲獎者:
# if a player picks the key, and the opponent has picked the value,
# then the player wins.
wins_against = {
Choices.rock: Choices.scissors,
Choices.paper: Choices.rock,
Choices.scissors: Choices.paper,
}
然后確定獲勝者:
if player_choice == cpu_choice:
print"\n Its a Tie!\n!"
elif wins_against[player_choice] == cpu_choice:
print"\n Congratulations!\n{} you won!".format(name)
else: # not the same, and not a win, so the player lost
print"\n Too bad!\n{} you lost!".format(name)
但是,該映射也可以是您的Choices
枚舉對象的一部分; 給那些設置一個wins_against
屬性:
if player_choice == cpu_choice:
print"\n Its a Tie!\n!"
elif player_choice.wins_against == cpu_choice:
print"\n Congratulations!\n{} you won!".format(name)
else:
print"\n Too bad!\n{} you lost!".format(name)
如果要使用enum
庫,則代碼可能變為:
from enum import Enum
class Choices(Enum):
rock = 1, 'scissors'
paper = 2, 'rock'
scissors = 3, 'paper'
def __new__(cls, value, win_against):
instance = object.__new__(cls)
instance._value_ = value
instance._win_against = win_against
return instance
@property
def win_against(self):
return type(self)[self._win_against]
while True:
options = '\n'.join(['{}-{}'.format(c.value, c.name) for c in choices])
player_choice = raw_input("\n\n{} choose a number:".format(
options, name))
try:
player_choice = int(player_choice)
player_choice = Choices(player_choice)
except ValueError:
print "Not a valid option, try again"
continue
cpu_choice = random.choice(list(Choices))
if player_choice is cpu_choice:
print"\n Its a Tie!\n!"
elif player_choice.wins_against is cpu_choice:
print"\n Congratulations!\n{} you won!".format(name)
else: # not the same, and not a win, so the player lost
print"\n Too bad!\n{} you lost!".format(name)
您應該使用if / elif / else語句-它只會比較條件語句,直到出現真值為止。 如果用戶輸入其他值,則可能要顯示一條錯誤消息
if player_choice == 1:
player_choice = Choices.rock
elif player_choice == 2:
player_choice = Choices.paper
elif player_choice == 3:
player_choice = Choices.scissors
else:
print "Invalid choice"
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.