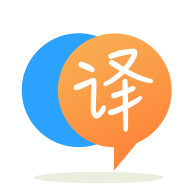
[英]How to get data from Secure REST API in lambda for Alexa skill?
[英]How to make an asynchronous api call for Alexa Skill application with a Lambda function?
我想從Lambda函數調用api。 我的處理程序由包含兩個必需插槽的意圖觸發。 因此,我事先不知道我將返回Dialog.Delegate
指令還是來自api請求的響應。 在調用Intent處理程序時,如何保證這些返回值?
這是我的處理程序:
const FlightDelayIntentHandler = {
canHandle(handlerInput) {
return handlerInput.requestEnvelope.request.type === 'IntentRequest'
&& handlerInput.requestEnvelope.request.intent.name === 'MyIntent';
},
handle(handlerInput) {
const request = handlerInput.requestEnvelope.request;
if (request.dialogState != "COMPLETED"){
return handlerInput.responseBuilder
.addDelegateDirective(request.intent)
.getResponse();
} else {
// Make asynchronous api call, wait for the response and return.
var query = 'someTestStringFromASlot';
httpGet(query, (result) => {
return handlerInput.responseBuilder
.speak('I found something' + result)
.reprompt('test')
.withSimpleCard('Hello World', 'test')
.getResponse();
});
}
},
};
這是我的幫助器函數,它發出請求:
const https = require('https');
function httpGet(query, callback) {
var options = {
host: 'myHost',
path: 'someTestPath/' + query,
method: 'GET',
headers: {
'theId': 'myId'
}
};
var req = https.request(options, res => {
res.setEncoding('utf8');
var responseString = "";
//accept incoming data asynchronously
res.on('data', chunk => {
responseString = responseString + chunk;
});
//return the data when streaming is complete
res.on('end', () => {
console.log('==> Answering: ');
callback(responseString);
});
});
req.end();
}
因此,我懷疑我將不得不使用Promise並在我的handle函數前面放置一個“異步”嗎? 我對這一切都很陌生,所以我不知道這意味着什么,尤其是考慮到我有兩個不同的返回值,一個是直接返回值,另一個是延遲值。 我該如何解決?
先感謝您。
正如您所懷疑的那樣,您的處理程序代碼在異步調用http.request之前已經完成,因此Alexa SDK沒有從handle
函數接收任何返回值,並且將向Alexa返回無效的響應。
我稍加修改了您的代碼,以在筆記本電腦上本地運行它來說明問題:
const https = require('https');
function httpGet(query, callback) {
var options = {
host: 'httpbin.org',
path: 'anything/' + query,
method: 'GET',
headers: {
'theId': 'myId'
}
};
var req = https.request(options, res => {
res.setEncoding('utf8');
var responseString = "";
//accept incoming data asynchronously
res.on('data', chunk => {
responseString = responseString + chunk;
});
//return the data when streaming is complete
res.on('end', () => {
console.log('==> Answering: ');
callback(responseString);
});
});
req.end();
}
function FlightDelayIntentHandler() {
// canHandle(handlerInput) {
// return handlerInput.requestEnvelope.request.type === 'IntentRequest'
// && handlerInput.requestEnvelope.request.intent.name === 'MyIntent';
// },
// handle(handlerInput) {
// const request = handlerInput.requestEnvelope.request;
// if (request.dialogState != "COMPLETED"){
// return handlerInput.responseBuilder
// .addDelegateDirective(request.intent)
// .getResponse();
// } else {
// Make asynchronous api call, wait for the response and return.
var query = 'someTestStringFromASlot';
httpGet(query, (result) => {
console.log("I found something " + result);
// return handlerInput.responseBuilder
// .speak('I found something' + result)
// .reprompt('test')
// .withSimpleCard('Hello World', 'test')
// .getResponse();
});
console.log("end of function reached before httpGet will return");
// }
// }
}
FlightDelayIntentHandler();
要運行此代碼,請不要忘記npm install http
,然后是node test.js
它產生
stormacq:~/Desktop/temp $ node test.js
end of function reached before httpGet will return
==> Answering:
I found something {
"args": {},
"data": "",
...
因此,關鍵是要等待http get
返回,然后再將響應返回給Alexa。 為此,我建議修改您的httpGet
函數以返回承諾,而不是使用回調。
修改后的代碼是這樣的(我保留了原始代碼作為注釋)
const https = require('https');
async function httpGet(query) {
return new Promise( (resolve, reject) => {
var options = {
host: 'httpbin.org',
path: 'anything/' + query,
method: 'GET',
headers: {
'theId': 'myId'
}
};
var req = https.request(options, res => {
res.setEncoding('utf8');
var responseString = "";
//accept incoming data asynchronously
res.on('data', chunk => {
responseString = responseString + chunk;
});
//return the data when streaming is complete
res.on('end', () => {
console.log('==> Answering: ');
resolve(responseString);
});
//should handle errors as well and call reject()!
});
req.end();
});
}
async function FlightDelayIntentHandler() {
// canHandle(handlerInput) {
// return handlerInput.requestEnvelope.request.type === 'IntentRequest'
// && handlerInput.requestEnvelope.request.intent.name === 'MyIntent';
// },
// handle(handlerInput) {
// const request = handlerInput.requestEnvelope.request;
// if (request.dialogState != "COMPLETED"){
// return handlerInput.responseBuilder
// .addDelegateDirective(request.intent)
// .getResponse();
// } else {
// Make asynchronous api call, wait for the response and return.
var query = 'someTestStringFromASlot';
var result = await httpGet(query);
console.log("I found something " + result);
// return handlerInput.responseBuilder
// .speak('I found something' + result)
// .reprompt('test')
// .withSimpleCard('Hello World', 'test')
// .getResponse();
//});
console.log("end of function reached AFTER httpGet will return");
// }
// }
}
FlightDelayIntentHandler();
運行此代碼將產生:
stormacq:~/Desktop/temp $ node test.js
==> Answering:
I found something{
"args": {},
"data": "",
...
end of function reached AFTER httpGet will return
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.