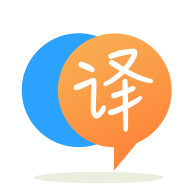
[英]Questions regarding the algorithm question in LeetCode - Remove Duplicates from Sorted Array using Java
[英]Leetcode : Remove Duplicates from Sorted Array
為什么這段代碼不被 Leetcode 編譯器接受? 我的程序在.netbeans 中運行。 但是 leetcode 編譯器不接受它。
這是我的代碼:
import java.util.Arrays;
public class RemoveDuplicateFromArray {
public static int[] removeDuplicates(int[] nums) {
int temp, count = 0 ,l = 0;
temp = nums[0];
for(int i = 1; i < nums.length - (1 + l); i++ ) {
if(temp == nums[i]) {
count = count + 1;
for(int j = i; j < nums.length - count; j++){
nums[j] = nums[j+1];
}
i = i - 1;
} else {
temp = nums[i];
i = i ;
}
l = count;
}
nums = Arrays.copyOfRange(nums, 0, nums.length-count);
if(nums.length == 2){
if(nums[0] == nums[1]){
nums = Arrays.copyOfRange(nums, 0, nums.length-1);
}
}
return nums;
}
這是我的主要方法:
public static void main(String[] args) {
int[] nums = new int[] {1,1,1}; // showing error here.
System.err.println("nums lenght: " +nums.length);
int new_nums[] = removeDuplicates(nums);
for(int i : new_nums) {
System.err.print(i + " ");
}
}
}
錯誤是:
不兼容的類型:int[] 無法轉換為 int。
我希望 leetcode 在處理這一行時遇到問題:
int new_nums[] = removeDuplicates(nums);
這不是定義 integer 數組的典型方法(這是 C 樣式)。 Java 確實支持語法,但有點神秘。 有關詳細信息,請參閱此答案。
試試這個:
int[] new_nums = removeDuplicates(nums);
我自己會這樣做:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
int[] nums = new int[]{1, 1, 1, 2, 2, 2, 3, 3, 3, 4, 5, 6, 7, 7, 7, 8, 9, 0, 0, 0, 0};
System.out.println("nums lenght: " + nums.length);
int[] new_nums = removeDuplicates(nums);
for (int i : new_nums) {
System.out.print(i + " ");
}
}
public static int[] removeDuplicates(int[] nums) {
List<Integer> found = new ArrayList();
for (int i = 1; i < nums.length; i++) {
if (!found.contains(nums[i])) {
found.add(nums[i]);
}
}
int[] ret = new int[found.size()];
for(int i = 0; i < found.size(); i++){
ret[i] = found.get(i);
}
return ret;
}
}
public class Solution {
public int removeDuplicates(int[] nums) {
int n = nums.length;
int i = 0;
int j = 1;
if (n <= 1) {
return n;
}
while (j <= n - 1) {
if (nums[i] != nums[j]) {
nums[i + 1] = nums[j];
i++;
}
j++;
}
return i + 1;
}
}
import static org.hamcrest.CoreMatchers.equalTo;
import static org.hamcrest.MatcherAssert.assertThat;
import java.util.Arrays;
import org.junit.jupiter.api.Test;
class SolutionTest {
@Test
void removeDuplicates() {
int[] array = new int[] {1, 1, 2};
int end = new Solution().removeDuplicates(array);
assertThat(Arrays.toString(Arrays.copyOfRange(array, 0, end)), equalTo("[1, 2]"));
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.