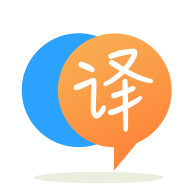
[英]Tools and best practices for organizing large javascript applications
[英]JavaScript: Organizing a Matrix By Best Fit
一個人如何組織一個動態矩陣以獲得最佳擬合? 因此,假設您試圖始終顯示最適合顯示器的顯示,並且需要組織所有單元,以使每個項目之間沒有空隙。 每個項目的大小可以為1-12,每行的最大寬度為12。使用示例數據集,如何動態排序並生成最適合顯示的新數組?
let matrixExample = [{
size: 10,
type: 'card'
}, {
size: 4,
type: 'card'
}, {
size: 2,
type: 'card'
}, {
size: 11,
type: 'card'
}, {
size: 6,
type: 'card'
}];
let endingResult = [
[{
size: 10,
type: 'card'
}, {
size: 2,
type: 'card'
}],
[{
size: 4,
type: 'card'
}, {
size: 6,
type: 'card'
}],
[{
size: 11,
type: 'card'
}]
];
用戶的目的是什么? 在為UI生成動態數據時,UI需要針對組件空間進行優化。
這似乎是垃圾箱包裝問題的一個示例。
這不是特別容易解決的問題,更精確的擬合可能會更復雜。
以下是一個貪婪算法,該算法應該可以粗略地解決您的問題。 可能會獲得更好的匹配,但是,就像這樣做一樣,會使事情變得更復雜且計算量更大。
該解決方案恰好是遞歸的,並且具有一定的功能,但這只是我的偏愛。 如果您不希望使代碼具有功能性或遞歸性,則可以使用更整潔,更便宜的算法。
const matrixExample = [{ size: 10, type: 'card' }, { size: 4, type: 'card' }, { size: 2, type: 'card' }, { size: 11, type: 'card' }, { size: 6, type: 'card' }]; const sumCardList = cardList => cardList.reduce((prev, curr) => prev + curr.size, 0); const packNextToBin = (cards, bins, max) => { if (cards.length === 0) { // there are no more cards to pack, use bins as is return bins; } // get the next card to pack into the bins const cardToPack = cards[0]; // get the indices of bins which can still be filled const availableBinIndices = bins .map((bin, i) => ({sum: sumCardList(bin), index: i})) .filter(binData => binData.sum + cardToPack.size < max) .map(binData => binData.index); // if there are no more bins which can fit this card, makea new bin if (availableBinIndices.length === 0) { const updatedBins = [ ...bins, [ cardToPack ] ]; return packNextToBin( cards.slice(1), updatedBins, max ); } // get the first available bin which can accept the card const binToPack = availableBinIndices[0]; // get a version of the matched bin with the new card added const binWithInsertion = [ ...bins[binToPack], cardToPack, ]; // get the bins with the updated bin updated const updatedBins = bins .map((bin, i) => i === binToPack ? binWithInsertion : bin ); // pack the next card into the bins return packNextToBin( cards.slice(1), updatedBins, max ); } const results = packNextToBin(matrixExample, [[]], 12) console.dir(results)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.