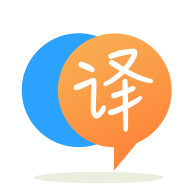
[英]How to retrieve documents in firestore order with timestamp with React
[英]Add timestamp in Firestore documents
我是 Firestore 的新手。 Firestore 文檔說...
重要提示:與 Firebase 實時數據庫中的“推送 ID”不同,Cloud Firestore 自動生成的 ID 不提供任何自動排序。 如果您希望能夠按創建日期對文檔進行排序,您應該將時間戳存儲為文檔中的一個字段。
參考:https://firebase.google.com/docs/firestore/manage-data/add-data
那么我是否必須在文檔中創建鍵名作為timestamp
? 或created
足以滿足 Firestore 文檔中的上述聲明。
{
"created": 1534183990,
"modified": 1534183990,
"timestamp":1534183990
}
firebase.firestore.FieldValue.serverTimestamp()
無論你想怎么稱呼它都很好afaik。 然后你可以使用 orderByChild('created')。
我在設置時間時也主要使用 firebase.database.ServerValue.TIMESTAMP
ref.child(key).set({
id: itemId,
content: itemContent,
user: uid,
created: firebase.database.ServerValue.TIMESTAMP
})
使用 firestore Timestamp 類firebase.firestore.Timestamp.now()
。
由於firebase.firestore.FieldValue.serverTimestamp()
不適用於來自 firestore 的add
方法。 參考
對於 Firestore
ref.doc(key).set({
created: firebase.firestore.FieldValue.serverTimestamp()
})
使用 FIRSTORE 的實時服務器時間戳
import firebase from "firebase/app";
const someFunctionToUploadProduct = () => {
firebase.firestore().collection("products").add({
name: name,
price : price,
color : color,
weight :weight,
size : size,
createdAt : firebase.firestore.FieldValue.serverTimestamp()
})
.then(function(docRef) {
console.log("Document written with ID: ", docRef.id);
})
.catch(function(error) {
console.error("Error adding document: ", error);
});
}
您只需要導入“ firebase ”,然后在需要的地方調用firebase.firestore.FieldValue.serverTimestamp() 即可。 不過要小心拼寫,它的“ serverTimestamp() ”。 在此示例中,它在上傳到 firestore 的產品集合時為“ createdAt ”提供時間戳值。
沒錯,就像大多數數據庫一樣,Firestore 不存儲創建時間。 為了按時間對對象進行排序:
在 Web 客戶端上創建時間戳(選項 1):
db.collection("messages").doc().set({
....
createdAt: firebase.firestore.Timestamp.now()
})
這里需要注意的是Timestamp.now()
使用本地機器時間。 因此,如果這是在客戶端計算機上運行,則無法保證時間戳是准確的。 如果您在服務器上設置此設置,或者保證順序不是那么重要,則可能沒問題。
您還可以使用時間戳標記(通常更好):
db.collection("messages").doc().set({
....
createdAt: firebase.firestore.FieldValue.serverTimestamp()
})
時間戳標記是一個令牌,它告訴 Firestore 服務器在第一次寫入時設置時間服務器端。
您可能會注意到我使用了doc().set()
。 這相當於add()
。 正式地,FieldValues 只支持 set() 和 update()。
此外,如果您在寫入之前閱讀哨兵(例如,在偵聽器中)它將為 NULL,除非您像這樣閱讀文檔:
doc.data({ serverTimestamps: 'estimate' })
使用以下內容設置您的查詢:
// quick and dirty way, but uses local machine time
const midnight = new Date(firebase.firestore.Timestamp.now().toDate().setHours(0, 0, 0, 0));
const todaysMessages = firebase
.firestore()
.collection(`users/${user.id}/messages`)
.orderBy('createdAt', 'desc')
.where('createdAt', '>=', midnight);
請注意,此查詢使用本地機器時間( Timestamp.now()
)。 如果您的應用在客戶端上使用正確的時間真的很重要,您可以利用 Firebase 的實時數據庫的此功能:
const serverTimeOffset = (await firebase.database().ref('/.info/serverTimeOffset').once('value')).val();
const midnightServerMilliseconds = new Date(serverTimeOffset + Date.now()).setHours(0, 0, 0, 0);
const midnightServer = new Date(midnightServerMilliseconds);
該文檔並未建議您的任何字段的名稱。 你引用的部分只是說兩件事:
這個解決方案對我有用:
Firestore.instance.collection("collectionName").add({'created': Timestamp.now()});
試試這個 Swift 4 Timestamp(date: Date())
let docData: [String: Any] = [
"stringExample": "Hello world!",
"booleanExample": true,
"numberExample": 3.14159265,
"dateExample": Timestamp(Date()),
"arrayExample": [5, true, "hello"],
"nullExample": NSNull(),
"objectExample": [
"a": 5,
"b": [
"nested": "foo"
]
]
]
db.collection("data").document("one").setData(docData) { err in
if let err = err {
print("Error writing document: \(err)")
} else {
print("Document successfully written!")
}
}
它與我合作的方式只是從快照參數snapshot.updateTime 中獲取時間戳
exports.newUserCreated = functions.firestore.document('users/{userId}').onCreate(async (snapshot, context) => {
console.log('started! v1.7');
const userID = context.params['userId'];
firestore.collection(`users/${userID}/lists`).add({
'created_time': snapshot.updateTime,
'name':'Products I ♥',
}).then(documentReference => {
console.log("initial public list created");
return null;
}).catch(error => {
console.error('Error creating initial list', error);
process.exit(1);
});
});
我正在使用 Firestore 存儲來自帶有 Python 的 Raspberry PI 的數據。 管道是這樣的:
Raspberry PI(使用 paho-mqtt 的 Python) -> Google Cloud IoT -> Google Cloud Pub/Sub -> Firebase Functions -> Firestore。
設備中的數據是一個 Python 字典。 我將其轉換為 JSON。 我遇到的問題是 paho-mqtt 只會將數據作為字符串發送(發布),而我的數據字段之一是時間戳。 該時間戳從設備中保存,因為它准確地說明了何時進行測量,而不管數據最終何時存儲在數據庫中。
當我發送 JSON 結構時,Firestore 會將我的字段“時間戳”存儲為字符串。 這不方便。 所以這是解決方案。
我在 Cloud Function 中進行了一個由 Pub/Sub 觸發的轉換,以使用 Moment 庫寫入 Firestore 進行轉換。
注意:我在 python 中獲取時間戳:
currenttime = datetime.datetime.utcnow()
var moment = require('moment'); // require Moment
function toTimestamp(strDate){
return parsedTime = moment(strDate, "YYYY-MM-DD HH:mm:ss:SS");
}
exports.myFunctionPubSub = functions.pubsub.topic('my-topic-name').onPublish((message, context) => {
let parsedMessage = null;
try {
parsedMessage = message.json;
// Convert timestamp string to timestamp object
parsedMessage.date = toTimestamp(parsedMessage.date);
// Get the Device ID from the message. Useful when you have multiple IoT devices
deviceID = parsedMessage._deviceID;
let addDoc = db.collection('MyDevices')
.doc(deviceID)
.collection('DeviceData')
.add(parsedMessage)
.then ( (ref) => {
console.log('Added document ID: ', ref.id);
return null;
}).catch ( (error) => {
console.error('Failed to write database', error);
return null;
});
} catch (e) {
console.error('PubSub message was not JSON', e);
}
// // Expected return or a warning will be triggered in the Firebase Function logs.
return null;
});
火石方法不起作用。 使用 java.sql.Timestamp 中的 Timestamp 並且不要轉換為字符串.. 然后 firestone 正確格式化它。 例如標記 now() 使用:
val timestamp = Timestamp(System.currentTimeMillis())
在 Firestore 中存儲時間的多種方式
firebaseAdmin.firestore.FieldValue.serverTimestamp()
方法。 將文檔寫入 Firestore 時將計算實際時間戳。firebaseAdmin.firestore.Timestamp.now()
方法。對於這兩種方法,下次獲取數據時,它將返回 Firestore Timestamp 對象:
因此,您首先需要將其轉換為原生 js Date
對象,然后您可以對其執行方法,例如toISOString()
。
export function FStimestampToDate(
timestamp:
| FirebaseFirestore.Timestamp
| FirebaseFirestore.FieldValue
): Date {
return (timestamp as FirebaseFirestore.Timestamp).toDate();
}
存儲為 unix 時間戳Date.now
,它將存儲為數字,即1627235565028
但您將無法在 firestore db 中將其視為可讀日期。
要查詢此 Firestore 字段,您需要將日期轉換為時間戳,然后進行查詢。
存儲為new Date().toISOString()
即"2021-07-25T17:56:40.373Z"
但您將無法對此執行日期范圍查詢。
我更喜歡第二種或第三種方式。
根據文檔,您可以“將文檔中的一個字段設置為服務器時間戳,用於跟蹤服務器何時收到更新”。
例子:
import { updateDoc, serverTimestamp } from "firebase/firestore";
const docRef = doc(db, 'objects', 'some-id');
// Update the timestamp field with the value from the server
const updateTimestamp = await updateDoc(docRef, {
timestamp: serverTimestamp() // this does the trick!
});
谷歌搜索 2 小時后分享對我有用的內容,適用於 firebase 9+
import { serverTimestamp } from "firebase/firestore";
export const postData = ({ name, points }: any) => {
const scoresRef = collection(db, "scores");
return addDoc(scoresRef, {
name,
points
date: serverTimestamp(),
});
};
斯威夫特 5.1
...
"dateExample": Timestamp(date: Date()),
...
來自 Firestore 的最新版本你應該使用它如下
import { doc, setDoc, Timestamp } from "firebase/firestore";
const docData = {
...
dateExample: Timestamp.fromDate(new Date("December 10, 1815"))
};
await setDoc(doc(db, "data", "one"), docData);
或服務器時間戳
import { updateDoc, serverTimestamp } from "firebase/firestore";
const docRef = doc(db, 'objects', 'some-id');
const updateTimestamp = await updateDoc(docRef, {
timestamp: serverTimestamp()
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.