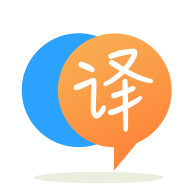
[英]How to get the value of Firebase storage download url and store it in firebase storage…,
[英]How to get the download Url of firebase file storage
我無法理解獲取下載 URl 的過程,有人可以很好地分解給我嗎? 所以我在這里有這個上傳組件:
import { Component, OnInit } from '@angular/core';
import { AngularFireStorage, AngularFireUploadTask } from
'angularfire2/storage';
import { AngularFirestore } from 'angularfire2/firestore';
import { Observable } from 'rxjs/Observable';
import { tap, filter, switchMap } from 'rxjs/operators';
import { storage } from 'firebase/storage';
@Component({
selector: 'file-upload',
templateUrl: './file-upload.component.html',
styleUrls: ['./file-upload.component.scss']
})
export class FileUploadComponent {
// Main task
task: AngularFireUploadTask;
// Progress monitoring
percentage: Observable<number>;
snapshot: Observable<any>;
// Download URL
downloadURL: Observable<string>;
// State for dropzone CSS toggling
isHovering: boolean;
constructor(private storage: AngularFireStorage, private db: AngularFirestore) { }
toggleHover(event: boolean) {
this.isHovering = event;
}
startUpload(event: FileList) {
// The File object
const file = event.item(0)
// Client-side validation example
if (file.type.split('/')[0] !== 'image') {
console.error('unsupported file type :( ')
return;
}
// The storage path
const path = `test/${new Date().getTime()}_${file.name}`;
// Totally optional metadata
const customMetadata = { app: 'My AngularFire-powered PWA!' };
// The main task
this.task = this.storage.upload(path, file, { customMetadata })
// Progress monitoring
this.percentage = this.task.percentageChanges();
this.snapshot = this.task.snapshotChanges().pipe(
tap(snap => {
console.log(snap)
if (snap.bytesTransferred === snap.totalBytes) {
// Update firestore on completion
this.db.collection('photos').add( { path, size: snap.totalBytes })
}
})
)
// The file's download URL
this.downloadURL = this.task.downloadURL();
console.log(this.downloadURL)
const ref = this.storage.ref(path);
this.task = ref.put(file, {customMetadata});
this.downloadURL = this.task.snapshotChanges().pipe(
filter(snap => snap.state === storage.TaskState.SUCCESS),
switchMap(() => ref.getDownloadURL())
)
console.log(this.downloadURL);
}
// Determines if the upload task is active
isActive(snapshot) {
return snapshot.state === 'running' && snapshot.bytesTransferred < snapshot.totalBytes
}}
我嘗試安慰獲取下載 URL 的假設方式,但它是空的,我已經看到了其他一些方法來完成它,但似乎無法正確完成。 即使使用snapshot.downloadURL,下載URL 也始終為空。 以下是 package.json:
{
"name": "storage-app",
"version": "0.0.0",
"license": "MIT",
"scripts": {
"ng": "ng",
"start": "ng serve",
"build": "ng build",
"test": "ng test",
"lint": "ng lint",
"e2e": "ng e2e"
},
"private": true,
"dependencies": {
"@angular/animations": "^6.0.3",
"@angular/common": "^6.0.3",
"@angular/compiler": "^6.0.3",
"@angular/core": "^6.0.3",
"@angular/forms": "^6.0.3",
"@angular/http": "^6.0.3",
"@angular/platform-browser": "^6.0.3",
"@angular/platform-browser-dynamic": "^6.0.3",
"@angular/platform-server": "^6.0.3",
"@angular/router": "^6.0.3",
"angularfire2": "5.0.0-rc.6",
"core-js": "^2.5.4",
"firebase": "4.12.1",
"rxjs": "^6.0.0",
"rxjs-compat": "^6.2.2",
"zone.js": "^0.8.26"
},
"devDependencies": {
"@angular/cli": "^6.0.8",
"@angular/compiler-cli": "^6.0.3",
"@angular/language-service": "^6.0.3",
"@types/jasmine": "~2.5.53",
"@types/jasminewd2": "~2.0.2",
"@types/node": "~6.0.60",
"codelyzer": "^4.0.1",
"jasmine-core": "~2.6.2",
"jasmine-spec-reporter": "~4.1.0",
"karma": "~1.7.0",
"karma-chrome-launcher": "~2.1.1",
"karma-cli": "~1.0.1",
"karma-coverage-istanbul-reporter": "^1.2.1",
"karma-jasmine": "~1.1.0",
"karma-jasmine-html-reporter": "^0.2.2",
"protractor": "~5.1.2",
"ts-node": "~3.2.0",
"tslint": "~5.7.0",
"typescript": "~2.7.2",
"@angular-devkit/build-angular": "~0.6.8"
}
}
提前致謝
您可以從存儲引用中檢索下載 url:
loading = false;
uploadFile(event) {
this.loading = true;
const file = event.target.files[0];
// give it a random file name
const path = Math.random().toString(36).substring(7);
const storageRef = this.storage.ref(path);
const task = this.storage.upload(path, file);
return from(task).pipe(
switchMap(() => storageRef.getDownloadURL()),
tap(url => {
// use url here, e.g. assign it to a model
}),
mergeMap(() => {
// e.g. make api call, e.g. save the model
}),
finalize(() => this.loading = false)
).subscribe(() => {
// success
}, error => {
// failure
});
}
我正在使用 angularfire 5.0.0-rc.11
這是一個簡單的例子來幫助你理解如何(取自 AngularFire2 GitHub):
uploadPercent: Observable < number > ;
downloadURL: Observable < string > ;
constructor(
private storage: AngularFireStorage
) {
}
uploadFile(event) {
const file = event.target.files[0];
const filePath = 'files';
const fileRef = this.storage.ref(filePath);
const task = this.storage.upload(filePath, file);
// observe percentage changes
this.uploadPercent = task.percentageChanges();
// get notified when the download URL is available
task.snapshotChanges().pipe(
finalize(() => this.downloadURL = fileRef.getDownloadURL())
)
.subscribe()
}
這是用於此的模板:
<input type="file" (change)="uploadFile($event)" />
<div>{{ uploadPercent | async }}</div>
<a [href]="downloadURL | async">{{ downloadURL | async }}</a>
這是發生了什么:
我們正在處理文件輸入的change
事件。 完成后,我們將獲取要上傳的文件。 然后,我們通過調用ref
並將路徑傳遞給我們的文件,在 Firebase 存儲上創建文件引用。 這將有助於稍后檢索文件下載 URL。
之后,我們通過在storage
上調用upload 並將文件路徑和要上傳的文件傳遞給它來創建一個AngularFireUplaodTask。
在此任務中,我們可以通過在上傳任務上調用percentageChanges
來檢查文件上傳百分比。 這又是一個 Observable,因此我們正在使用async
管道監聽和打印 DOM 上的更改。
當上傳任務完成時, finalize
將被觸發。 那時我們將能夠通過在我們之前創建的fileRef
上調用getDownloadURL
來獲取下載 url。
您可以查看此StackBlitz以獲取更多信息。
現在無法在上傳的即時結果中訪問下載 URL。 此更改是在幾個月前對 Firebase 客戶端 SDK 進行的。
相反,您必須調用 getDownloadURL(或該 JavaScript 函數的任何 Angular 綁定)來獲取 URL 作為上傳完成后的第二個請求。
Angularfire 提供了這個超級方便的管道,getDownloadURL:
<img [src]="'users/davideast.jpg' | getDownloadURL" />
官方文檔。
結束。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.