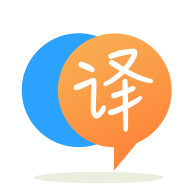
[英]Passing function to child component this.props.children method undefined
[英]Running method of component child from this.props.children array
import React from "react";
import ReactDOM from "react-dom";
class NestedComponent extends React.Component {
constructor(props) {
super(props);
this.childMethod = this.childMethod.bind(this);
}
childMethod() {
alert("Child method one ran");
}
render() {
return <div>NestedComponent</div>;
}
}
class NestedComponentTwo extends React.Component {
constructor(props) {
super(props);
this.childMethod = this.childMethod.bind(this);
}
childMethod() {
alert("Child method two ran");
}
render() {
return <div>NestedComponentTwo</div>;
}
}
class WrappingComponent extends React.Component {
constructor(props) {
super(props);
this.runMethod = this.runMethod.bind(this);
}
runMethod() {
let child = this.props.children[0];
/** Always returns as undefined */
//if (typeof child.childMethod == "function") {
// child.childMethod();
//}
/**
* EDIT: Close, however the this binding seems to not be working. I can however provide the childs props to the childMethod and work with that.
*/
if(typeof child.type.prototype.childMethod == "funciton"){
child.type.prototype.childMethod();
}
}
render() {
return (
<div>
{this.props.children}
<button onClick={this.runMethod}>run</button>
</div>
);
}
}
const App = ({}) => {
return (
<div>
<WrappingComponent>
<NestedComponent />
<NestedComponentTwo />
</WrappingComponent>
</div>
);
};
if (document.getElementById("example")) {
ReactDOM.render(<App />, document.getElementById("example"));
}
因此,目標是將可選方法附加到嵌套組件上,該方法可以從包裝組件執行,就像事件發射器一樣。 但是由於某種原因,子組件上存在的方法聲稱不存在。 但是,每當我記錄從this.props.children
數組中提取的子組件時,原型都會列出該方法。
我是否缺少通過方法變量訪問子組件方法的特殊方法?
找到了可用於訪問它的變量。 如果有人對此有更多的了解,或者我做不到的原因是不好的作法,請告訴我。
在需要的地方編輯問題,但是下面的項目正在訪問子功能:
child.type.prototype.childMethod
似乎不維護this
綁定。 傳遞道具確實可以。
您應該在頂層組件( App
組件)中管理所有這些邏輯
class NestedComponent extends React.Component { constructor(props) { super(props); this.childMethod = this.childMethod.bind(this); } childMethod() { alert("Child method one ran"); } render() { return <div>NestedComponent</div>; } } class NestedComponentTwo extends React.Component { constructor(props) { super(props); this.childMethod = this.childMethod.bind(this); } childMethod() { alert("Child method two ran"); } render() { return <div>NestedComponentTwo</div>; } } class WrappingComponent extends React.Component { render() { return ( <div> {this.props.children} <button onClick={this.props.onClick}>run</button> </div> ); } } class App extends React.Component { constructor(props) { super(props); this.runMethod = this.runMethod.bind(this); } runMethod() { if (this.nestedComponent) { this.nestedComponent.childMethod(); } } render() { return ( <div> <WrappingComponent onClick={this.runMethod}> <NestedComponent ref={el => this.nestedComponent = el} /> <NestedComponentTwo /> </WrappingComponent> </div> ); } }; if (document.getElementById("example")) { ReactDOM.render(<App />, document.getElementById("example")); }
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script> <div id="example"></div>
而且不建議使用帶字符串屬性的ref
https://reactjs.org/docs/refs-and-the-dom.html#legacy-api-string-refs
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.