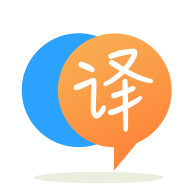
[英]How do you migrate an existing core data iOS 7 app's data into iCloud?
[英]iOS 11+ How to migrate existing Core Data to Shared App Group for use in extension?
當我使用核心數據模板創建 iOS 11 應用程序時,它會在 AppDelete.m 中自動生成以下代碼。
synthesize persistentContainer = _persistentContainer;
- (NSPersistentContainer *)persistentContainer {
// The persistent container for the application. This implementation creates and returns a container, having loaded the store for the application to it.
@synchronized (self) {
if (_persistentContainer == nil) {
_persistentContainer = [[NSPersistentContainer alloc] initWithName:@"My_History"];
[_persistentContainer loadPersistentStoresWithCompletionHandler:^(NSPersistentStoreDescription *storeDescription, NSError *error) {
if (error != nil) {
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
/*
Typical reasons for an error here include:
* The parent directory does not exist, cannot be created, or disallows writing.
* The persistent store is not accessible, due to permissions or data protection when the device is locked.
* The device is out of space.
* The store could not be migrated to the current model version.
Check the error message to determine what the actual problem was.
*/
NSLog(@"Unresolved error %@, %@", error, error.userInfo);
abort();
}
}];
}
}
return _persistentContainer;
}
- (void)saveContext {
NSManagedObjectContext *context = self.persistentContainer.viewContext;
NSError *error = nil;
if ([context hasChanges] && ![context save:&error]) {
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
NSLog(@"Unresolved error %@, %@", error, error.userInfo);
abort();
}
我想添加一個訪問核心數據歷史記錄的 Today 和 iMessage 擴展。 根據我閱讀的內容,如果此數據存在,我需要將其遷移到共享應用程序容器中。 我該怎么做?
代碼在目標 C 中。
我已經閱讀了其他涉及此問題的問題,但所有這些問題似乎都是在 Apple 改變核心數據的工作方式以使其更容易之前。 正如您在我的代碼中看到的那樣,我從未指定數據存儲的確切文件名是什么。 我看到的每個示例都有類似“My_History.sqllite”的內容。 我什至不知道我的是否是 sql lite 數據庫,它只是由該代碼創建的。
solidsnake4444的回答節省了我的時間。 這是 Swift 5.0 版本。
lazy var persistentContainer: NSPersistentContainer = {
let container = NSPersistentContainer(name: "MyApp")
let storeURL = FileManager.default.containerURL(forSecurityApplicationGroupIdentifier: "group.com.my.app")!.appendingPathComponent("MyApp.sqlite")
var defaultURL: URL?
if let storeDescription = container.persistentStoreDescriptions.first, let url = storeDescription.url {
defaultURL = FileManager.default.fileExists(atPath: url.path) ? url : nil
}
if defaultURL == nil {
container.persistentStoreDescriptions = [NSPersistentStoreDescription(url: storeURL)]
}
container.loadPersistentStores(completionHandler: { [unowned container] (storeDescription, error) in
if let error = error as NSError? {
fatalError("Unresolved error \(error), \(error.userInfo)")
}
if let url = defaultURL, url.absoluteString != storeURL.absoluteString {
let coordinator = container.persistentStoreCoordinator
if let oldStore = coordinator.persistentStore(for: url) {
do {
try coordinator.migratePersistentStore(oldStore, to: storeURL, options: nil, withType: NSSQLiteStoreType)
} catch {
print(error.localizedDescription)
}
// delete old store
let fileCoordinator = NSFileCoordinator(filePresenter: nil)
fileCoordinator.coordinate(writingItemAt: url, options: .forDeleting, error: nil, byAccessor: { url in
do {
try FileManager.default.removeItem(at: url)
} catch {
print(error.localizedDescription)
}
})
}
}
})
return container
}()
我最終得到它執行以下操作。 sqlite 文件實際上是我的 init 名稱加上最后的 .sqlite。
+ (NSPersistentContainer*) GetPersistentContainer {
//Init the store.
NSPersistentContainer *_persistentContainer = [[NSPersistentContainer alloc] initWithName:@"Test_App"];
//Define the store url that is located in the shared group.
NSURL* storeURL = [[[NSFileManager defaultManager] containerURLForSecurityApplicationGroupIdentifier:@"group.Test_App"] URLByAppendingPathComponent:@"Test_App.sqlite"];
//Determine if we already have a store saved in the default app location.
BOOL hasDefaultAppLocation = [[NSFileManager defaultManager] fileExistsAtPath: _persistentContainer.persistentStoreDescriptions[0].URL.path];
//Check if the store needs migration.
BOOL storeNeedsMigration = hasDefaultAppLocation && ![_persistentContainer.persistentStoreDescriptions[0].URL.absoluteString isEqualToString:storeURL.absoluteString];
//Check if the store in the default location does not exist.
if (!hasDefaultAppLocation) {
//Create a description to use for the app group store.
NSPersistentStoreDescription *description = [[NSPersistentStoreDescription alloc] init];
//set the automatic properties for the store.
description.shouldMigrateStoreAutomatically = true;
description.shouldInferMappingModelAutomatically = true;
//Set the url for the store.
description.URL = storeURL;
//Replace the coordinator store description with this description.
_persistentContainer.persistentStoreDescriptions = [NSArray arrayWithObjects:description, nil];
}
//Load the store.
[_persistentContainer loadPersistentStoresWithCompletionHandler:^(NSPersistentStoreDescription *storeDescription, NSError *error) {
//Check that we do not have an error.
if (error == nil) {
//Check if we need to migrate the store.
if (storeNeedsMigration) {
//Create errors to track migration and deleting errors.
NSError *migrateError;
NSError *deleteError;
//Store the old location URL.
NSURL *oldStoreURL = storeDescription.URL;
//Get the store we want to migrate.
NSPersistentStore *store = [_persistentContainer.persistentStoreCoordinator persistentStoreForURL: oldStoreURL];
//Set the store options.
NSDictionary *storeOptions = @{ NSSQLitePragmasOption : @{ @"journal_mode" : @"WAL" } };
//Migrate the store.
NSPersistentStore *newStore = [_persistentContainer.persistentStoreCoordinator migratePersistentStore: store toURL:storeURL options:storeOptions withType:NSSQLiteStoreType error:&migrateError];
//Check that the store was migrated.
if (newStore && !migrateError) {
//Remove the old SQLLite database.
[[[NSFileCoordinator alloc] init] coordinateWritingItemAtURL: oldStoreURL options: NSFileCoordinatorWritingForDeleting error: &deleteError byAccessor: ^(NSURL *urlForModifying) {
//Create a remove error.
NSError *removeError;
//Delete the file.
[[NSFileManager defaultManager] removeItemAtURL: urlForModifying error: &removeError];
//If there was an error. Output it.
if (removeError) {
NSLog(@"%@", [removeError localizedDescription]);
}
}
];
//If there was an error. Output it.
if (deleteError) {
NSLog(@"%@", [deleteError localizedDescription]);
}
}
}
} else {
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
/*
Typical reasons for an error here include:
* The parent directory does not exist, cannot be created, or disallows writing.
* The persistent store is not accessible, due to permissions or data protection when the device is locked.
* The device is out of space.
* The store could not be migrated to the current model version.
Check the error message to determine what the actual problem was.
*/
NSLog(@"Unresolved error %@, %@", error, error.userInfo);
abort();
}
}];
//Return the container.
return _persistentContainer;
}
更新:
為了遷移現有的持久化存儲, NSPersistentContainer
包含了persistentStoreCoordinator
,它是NSPersistentStoreCoordinator
的一個實例。 這公開了migratePersistentStore:toURL:options:withType:error:
方法來遷移持久存儲。
我會做以下事情:
// Get the reference to the persistent store coordinator
let coordinator = persistentContainer.persistentStoreCoordinator
// Get the URL of the persistent store
let oldURL = persistentContainer.persistentStoreDescriptions.url
// Get the URL of the new App Group location
let newURL = NSFileManager.defaultManager().containerURLForSecurityApplicationGroupIdentifier("YOUR_APP_GROUP")
// Get the reference to the current persistent store
let oldStore = coordinator.persistentStore(for: oldURL)
// Migrate the persistent store
do {
try coordinator.migratePersistentStore(oldStore, to: newURL, options: nil, withType: NSSQLiteStoreType)
} catch {
// ERROR
}
請注意,以上內容尚未經過測試,並且我沒有處理 Optionals,因此它不完整。 另外,我很抱歉它在 Swift 中。 希望你可以很容易地用 Objective-C 編寫等價的代碼。
原來的:
下面概述了如何創建一個NSPersistentContainer
接口到一個非默認位置的持久存儲。
NSPersistentContainer
公開了defaultDirectoryURL
,並聲明:
此方法返回一個依賴於平台的
NSURL
,持久存儲將位於或當前位於該 NSURL。 可以在NSPersistentContainer
的子類中重寫此方法。
如果您將NSPersistentContainer
子類化並使用containerURLForSecurityApplicationGroupIdentifier
將defaultDirectoryURL
定義為 App Group 目錄,那么您應該能夠訪問您的應用程序和擴展之間的容器(假設它們具有相同的 App Group 權利)。
NSPersistentContainer
還公開了還具有 URL 實例的persistentStoreDescriptions
。 同樣,您可以在調用loadPersistentStoresWithCompletionHandler:
之前將其更新為 App Group URL。
請注意,我沒有使用NSPersistentContainer
,並且不知道這種共享是否會導致任何並發問題。
移動NSPersistentCloudKitContainer
時,這對我有用。 有幾點需要注意:
cloudKitContainerOptions
否則同步將停止工作migratePersistentStore
否則你會得到重復的記錄,而是使用replacePersistentStore
- 有關更多信息,請參閱此線程destroyPersistentStore
聽起來很有希望,但似乎並沒有真正刪除文件 - 請參閱此問題struct PersistenceController {
static let shared = PersistenceController()
let container: NSPersistentCloudKitContainer
init(inMemory: Bool = false) {
container = NSPersistentCloudKitContainer(name: "MyCuteDB")
if inMemory {
container.persistentStoreDescriptions.first!.url = URL(fileURLWithPath: "/dev/null")
} else {
// Use App Groups so app and extensions can access database
let sharedStoreURL = FileManager.default.containerURL(forSecurityApplicationGroupIdentifier: "group.com.yourdomain.yourapp")!.appendingPathComponent("\(container.name).sqlite")
let defaultStoreURL = container.persistentStoreDescriptions.first!.url! //the URL always starts out in the default location
// move database to shared location if needed
if FileManager.default.fileExists(atPath: defaultStoreURL.path) && !FileManager.default.fileExists(atPath: sharedStoreURL.path) {
let coordinator = container.persistentStoreCoordinator
do {
try coordinator.replacePersistentStore(at: sharedStoreURL, destinationOptions: nil, withPersistentStoreFrom: defaultStoreURL, sourceOptions: nil, ofType: NSSQLiteStoreType)
try? coordinator.destroyPersistentStore(at: defaultStoreURL, ofType: NSSQLiteStoreType, options: nil)
// destroyPersistentStore says it deletes the old store but it actually truncates so we'll manually delete the files
NSFileCoordinator(filePresenter: nil).coordinate(writingItemAt: defaultStoreURL.deletingLastPathComponent(), options: .forDeleting, error: nil, byAccessor: { url in
try? FileManager.default.removeItem(at: defaultStoreURL)
try? FileManager.default.removeItem(at: defaultStoreURL.deletingLastPathComponent().appendingPathComponent("\(container.name).sqlite-shm"))
try? FileManager.default.removeItem(at: defaultStoreURL.deletingLastPathComponent().appendingPathComponent("\(container.name).sqlite-wal"))
try? FileManager.default.removeItem(at: defaultStoreURL.deletingLastPathComponent().appendingPathComponent("ckAssetFiles"))
})
} catch {
//TODO: Handle error
}
}
// change URL from default to shared location
container.persistentStoreDescriptions.first!.url = sharedStoreURL
let description = container.persistentStoreDescriptions.first!
description.cloudKitContainerOptions = NSPersistentCloudKitContainerOptions(containerIdentifier: "iCloud.com.yourdomain.yourapp")
}
container.loadPersistentStores { description, error in
//TODO: Handle error
}
container.viewContext.mergePolicy = NSMergeByPropertyObjectTrumpMergePolicy
container.viewContext.automaticallyMergesChangesFromParent = true
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.