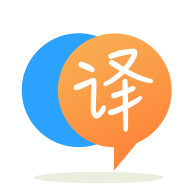
[英]how to read File from a particular line till the end of the File in java
[英]Java - read a file line by line till the end of the file
我正在開發一個項目,該項目需要我用Java查找給定圖的最小生成樹。 我是一個Java新手,因為我主要使用C ++。
我已經編寫了算法,但是有一種獨特的輸入格式,就像這樣:
0 1:33 2:0 3:12 4:29 5:32 6:22 7:13 8:45 9:21
1 0:6 2:18 3:2 4:26 5:41 6:8 7:47 8:13 9:19
2 0:22 1:28 3:49 4:47 5:5 6:16 7:32 8:5 9:34
3 0:21 1:24 2:2 4:29 5:20 6:39 7:17 8:21 9:3
4 0:27 1:20 2:38 3:4 5:14 6:25 7:0 8:24 9:11
5 0:20 1:7 2:29 3:15 4:3 6:19 7:11 8:19 9:41
6 0:14 1:36 2:6 3:45 4:18 5:33 7:43 8:22 9:36
7 0:25 1:12 2:15 3:45 4:18 5:43 6:41 8:37 9:26
8 0:29 1:44 2:23 3:15 4:34 5:45 6:27 7:29 9:4
9 0:18 1:41 2:20 3:25 4:18 5:10 6:10 7:49 8:42
或者,更清楚地說:
[source-vertex] [destination-vertex]:[weight] [destination-vertex]:[weight] ...
我想知道如何讀取這種輸入格式?
我正在考慮讀取每一行,直到文件結束,然后解析每一行中的數字。
所以您基本上有兩個問題:
Reader
並以適當的文本編碼從InputStream
讀取。 根據您的格式讀取數據的代碼:
File f = ... // file to read
try (BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(f), StandardCharsets.UTF_8))) {
// regular expression checking the format of each line
Pattern linePattern = Pattern.compile("(\\d+)(?:\\s+(\\d+):(\\d+))*");
// regular expression to find the index (first number) in a line
Pattern indexPattern = Pattern.compile("(\\d+)");
// regular expression to find the vertices (a:b) in a line
Pattern relationPattern = Pattern.compile("(\\d+):(\\d+)");
String line;
while ((line = reader.readLine()) != null) {
if (linePattern.matcher(line).matches()) {
Matcher indexMatcher = indexPattern.matcher(line);
if (indexMatcher.find()) {
int sourceVertex = Integer.parseInt(indexMatcher.group(1));
// do something with the sourceVertex
Matcher relationMatcher = relationPattern.matcher(line);
while (relationMatcher.find()) {
int destinationVertex = Integer.parseInt(relationMatcher.group(1));
int weight = Integer.parseInt(relationMatcher.group(2));
// do something with destinationVertex and weight
}
}
}
}
}
我建議使用Steam API並使用BuferedReader加載文件並使用lines()方法。 這將產生一個Stream,您可以根據需要對其進行操作。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.