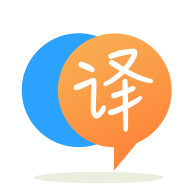
[英]How can i use the output of a php page to import data on a database?
[英]How can I import XML data in our database? (PHP)
我正在嘗試將XML數據導入我們的數據庫(mariadb)。 我對php很陌生。
我們的XML結構:
<?xml version="1.0" encoding="UTF-8"?>
<webexport>
<article key="98112" status="active">
<productattributes>
<group1>
<feature key="number">
<en name="Number" value="98112"></en>
<fr name="Nombre" value="98112"></fr>
<ger name="Nummer" value="98112"></ger>
</feature>
<feature key="description">
<en name="Item description" value="VKK-12-8m-11"></en>
<fr name="Désignation" value="VKK-12-8m-11"></fr>
<ger name="Artikelbezeichnung" value="VKK-12-8m-11"></ger>
</feature>
</group1>
</productattributes>
</article>
</webexport>
這就是我想要的數據庫中的內容:
+----+---------------+-----------+------+--------------------+--------------+
| id | articleid_des | articleid | lang | description_des | description |
+----+---------------+-----------+------+--------------------+--------------+
| 1 | Number | 98112 | en | Item description | VKK-12-8m-11 |
| 2 | Nombre | 98112 | fr | Désignation | VKK-12-8m-11 |
| 3 | Nummer | 98112 | de | Artikelbezeichnung | VKK-12-8m-11 |
+----+---------------+-----------+------+--------------------+--------------+
這是我當前的功能,我正在嘗試獲取屬性並將其放入變量($ en_des,$ en_val)。 但是之后,我不知道如何在我們的數據庫結構中正確獲取這些變量。
<?php
$xml=simplexml_load_file("exported.xml");
foreach($xml->children() as $article) {
foreach($article->children() as $productattr) {
foreach($productattr->children() as $group) {
foreach($group->children() as $feature) {
foreach($feature->children() as $en) {
$en_des=$en['name'];
$en_val=$en['value'];
echo $en_des;
echo "\n";
echo $en_val;
echo "\n";
}
}
}
}
}
這是我的功能的輸出:
Number 98112 Nombre 98112 Nummer 98112 Item description VKK-12-8m-11 Désignation VKK-12-8m-11 Artikelbezeichnung VKK-12-8m-11
考慮一下MySQL和MariaDB中可用的LOAD XML ,它需要XSLT , XSLT是一種專用的聲明性語言,例如SQL,由於需要的結構,它用於轉換XML文件:
<row>
<column1>value1</column1>
<column2>value2</column2>
</row>
XSLT (另存為.xsl文件-特殊的.xml文件)
使用Meunchian方法按<en>
, <fr>
和<ger>
標記分組。
<?xml version="1.0" encoding="UTF-8"?>
<xsl:stylesheet xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
version="1.0">
<xsl:output method="xml" indent="yes"/>
<xsl:strip-space elements="*"/>
<xsl:key name="lang_key" match="feature/*" use="name()"/>
<xsl:template match="/webexport">
<xsl:apply-templates select="article"/>
</xsl:template>
<xsl:template match="article|productattributes|group1">
<xsl:apply-templates select="*"/>
</xsl:template>
<xsl:template match="feature[position()=1]">
<table>
<xsl:for-each select="*[count(. | key('lang_key', name())[1]) = 1]">
<xsl:variable select="name()" name="curr_key"/>
<row>
<id><xsl:value-of select="position()"/></id>
<articleid_des><xsl:value-of select=".[name()=$curr_key]/@name"/></articleid_des>
<articleid><xsl:value-of select=".[name()=$curr_key]/@value"/></articleid>
<lang><xsl:value-of select="$curr_key"/></lang>
<description_des><xsl:value-of select="../following-sibling::feature/*[name()=$curr_key]/@name"/></description_des>
<description><xsl:value-of select="../following-sibling::feature/*[name()=$curr_key]/@value"/></description>
</row>
</xsl:for-each>
</table>
</xsl:template>
</xsl:stylesheet>
PHP (無需foreach
循環或if
邏輯)
// IMPORT XML
$xml = new DOMDocument('1.0', 'UTF-8');
$xml->load('/path/to/Input.xml');
// IMPORT XSLT
$xsl = new DOMDocument('1.0', 'UTF-8');
$xsl->load('/path/to/XSLT_Script.xsl');
// INITIALIZE TRANSFORMER
$proc = new XSLTProcessor;
$proc->importStyleSheet($xsl);
// TRANSFORM SOURCE
$newXML = $proc->transformToDoc($xml);
// SAVE TO FILE
file_put_contents('/path/to/Output.xml', $newXML);
// RUN MARIADB COMMAND (MAY NEED TO ALLOW --local-infile IN SETTINGS)
try {
$conn = new mysqli($servername, $username, $password, $dbname);
$conn->query("LOAD XML DATA INFILE '/path/to/Output.xml'
INTO TABLE myFinalTable
ROWS IDENTIFIED BY '<row>';");
} catch(Exception $e) {
echo $e->getMessage();
}
$conn->close();
XML輸出(用於數據庫導入)
<?xml version="1.0" encoding="UTF-8"?>
<table>
<row>
<id>1</id>
<articleid_des>Number</articleid_des>
<articleid>98112</articleid>
<lang>en</lang>
<description_des>Item description</description_des>
<description>VKK-12-8m-11</description>
</row>
<row>
<id>2</id>
<articleid_des>Nombre</articleid_des>
<articleid>98112</articleid>
<lang>fr</lang>
<description_des>Désignation</description_des>
<description>VKK-12-8m-11</description>
</row>
<row>
<id>3</id>
<articleid_des>Nummer</articleid_des>
<articleid>98112</articleid>
<lang>ger</lang>
<description_des>Artikelbezeichnung</description_des>
<description>VKK-12-8m-11</description>
</row>
</table>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.