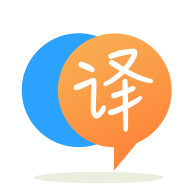
[英]How can I left join two javascript objects of arrays using properties as primary keys?
[英]How can I join two arrays of objects in JavaScript?
在JavaScript中聯接數據的最佳方法是什么? 是否有Python
類的庫(例如Pandas
)或迭代方式? 我有兩個帶有不同對象的數組。 列表orders
包含有關常規orders
信息,列表orders
已支付包含訂單是否已ordersPayed
和金額等信息。
const orders = [ { orderId: 1, orderDate: '2018-01-01', orderAmount: 100 }, { orderId: 2, orderDate: '2018-02-01', orderAmount: 100 }, { orderId: 3, orderDate: '2018-03-01', orderAmount: 100 }, { orderId: 4, orderDate: '2018-03-01', orderAmount: 100 }]; const ordersPayed = [ { orderId: 1, payedAmount: 90, debitorName: 'abc' }, { orderId: 3, payedAmount: 80, debitorName: 'abc' }, { orderId: 6, payedAmount: 90, debitorName: 'abc' }]; let newOrderList = []; for (i = 0; i < orders.length; i++) { for (j = 0; j < ordersPayed.length; j++) { if (orders[i].orderId == ordersPayed[j].orderId) { newOrderList.push(orders[i].orderId); newOrderList.push(orders[i].orderDate); newOrderList.push(orders[i].orderAmount); newOrderList.push(ordersPayed[j].payedAmount); newOrderList.push(ordersPayed[j].debitorName); } else if (j == (ordersPayed.length-1)) { newOrderList.push(orders[i].orderId); newOrderList.push(orders[i].orderDate); newOrderList.push(orders[i].orderAmount); newOrderList.push('not_payed_yet'); newOrderList.push('not_known_yet'); } } } console.log(newOrderList);
匹配通過鍵orderId
。 最后,我想創建一個包含所有訂單和相應信息的新列表,無論它們是否已經付款。
上面的代碼是我要采用的方法,但是由於性能原因以及是否存在更多陷阱,我不知道這是否很好。 所以我想到了一個匹配的庫或類似的東西。
不幸的是,這不能100%正確地工作。 結果應如下所示:
[{
orderId: 1,
orderDate: '2018-01-01',
orderAmount: 100,
payedAmount: 90
},
{
orderId: 2,
orderDate: '2018-02-01',
orderAmount: 100,
payedAmount: 'not_payed_yet'
},
{
orderId: 3,
orderDate: '2018-03-01',
orderAmount: 100,
payedAmount: 80
},
{
orderId: 4,
orderDate: '2018-03-01',
orderAmount: 100,
payedAmount: 'not_payed_yet'
}]
有人有提示嗎?
const newOrderList = orders.map((order, index) => {
let payedOrder = ordersPayed.find(o => o.orderId === order.orderId);
return Object.assign({}, order, payedOrder)
});
您可以嘗試以下解決方案:
// Returns an array with order objects, which contain all information
let newOrderList = orders.map((order, index) => {
let payedOrder = ordersPayed.find(o => o.orderId === order.orderId);
// Returns a new object to not manipulate the original one
return {
orderId: order.orderId,
orderDate: order.orderDate,
orderAmount: order.orderAmount,
payedAmount: payedOrder ? payedOrder.payedAmount : 'not_payed_yet',
debitorName: payedOrder ? payedOrder.debitorName: 'not_known_yet'
}
});
使用lodash和ES6箭頭表示法,解決方案可能會變得很短:
// Array of Javascript Objects 1: const orders = [{ orderId: 1, orderDate: '2018-01-01', orderAmount: 100 }, { orderId: 2, orderDate: '2018-02-01', orderAmount: 100 }, { orderId: 3, orderDate: '2018-03-01', orderAmount: 100 }, { orderId: 4, orderDate: '2018-03-01', orderAmount: 100 } ]; // Array of Javascript Objects 2: const ordersPayed = [{ orderId: 1, payedAmount: 90, debitorName: 'abc' }, { orderId: 3, payedAmount: 80, debitorName: 'abc' }, { orderId: 6, payedAmount: 90, debitorName: 'abc' } ]; var merged = _.merge(_.keyBy(orders, 'orderId'), _.keyBy(ordersPayed, 'orderId')); const newArr = _.map(merged, o => _.assign({ "payedAmount": "not_payed_yet", "debitorName": "not_known_yet" }, o)); var result = _.values(newArr); console.log(result);
.as-console-wrapper { max-height: 100% !important; top: 0; }
<script src="https://cdn.jsdelivr.net/npm/lodash@4.17.10/lodash.min.js"></script>
對於您的問題,我將Array.reduce
與Array.find
:
const newOrderList = orders.reduce((acc, order) => {
const { orderId } = order;
const orderPayed = ordersPayed.find((orderPayed) => orderPayed.orderId === orderId);
if (orderPayed) {
return [
...acc,
{
...order,
...orderPayed,
}
];
}
return [
...acc,
{
...order,
},
];
}, []);
您的代碼中有一個小錯誤。 else if
將無法按您希望的方式工作,因為每當最后一次匹配失敗時,您始終會將not found
條目推入新數組。 您可以嘗試調整后的代碼版本:
const orders = [ { orderId: 1, orderDate: '2018-01-01', orderAmount: 100 }, { orderId: 2, orderDate: '2018-02-01', orderAmount: 100 }, { orderId: 3, orderDate: '2018-03-01', orderAmount: 100 }, { orderId: 4, orderDate: '2018-03-01', orderAmount: 100 }]; const ordersPayed = [ { orderId: 1, payedAmount: 90, debitorName: 'abc' }, { orderId: 3, payedAmount: 80, debitorName: 'abc' }, { orderId: 6, payedAmount: 90, debitorName: 'abc' }]; let newOrderList = []; for (i = 0; i < orders.length; i++) { let payed = false; for (j = 0; j < ordersPayed.length; j++) { if (orders[i].orderId == ordersPayed[j].orderId) { newOrderList.push({ orderId: orders[i].orderId, orderDate: orders[i].orderDate, orderAmount: orders[i].orderAmount, payedAmount: ordersPayed[j].payedAmount, debitorName: ordersPayed[j].debitorName }); payed = true; } } if (!payed) { newOrderList.push({ orderId: orders[i].orderId, orderDate: orders[i].orderDate, orderAmount: orders[i].orderAmount, payedAmount: 'not_payed_yet', debitorName: 'not_known_yet' }); } } console.log(newOrderList);
但是請記住,只有當數據集之間的關系為1:1
,此方法才起作用。 這意味着如果你可以有多個條目ordersPayed
在一個條目orders
,結果也將具有這些訂單的多個條目。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.