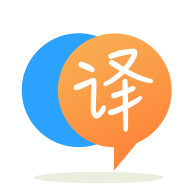
[英]How to extract a string between the first two and last two characters in java?
[英]how do I extract data between two characters in java
String text = "/'Team1 = 6', while /'Team2 = 4', and /'Team3 = 2'";
String[] body = text.split("/|,");
String b1 = body[1];
String b2 = body[2];
String b3 = body[3];
所需結果:
b1 = 'Team1 = 6'
b2 = 'Team2 = 4'
b3 = 'Team3 = 2'
使用正則表達式。 像這樣:
String text = "/'Team1 = 6', while /'Team2 = 4', and /'Team3 = 2'";
Matcher m = Pattern.compile("(\\w+\\s=\\s\\d+)").matcher(text);
// \w+ matches the team name (eg: Team1). \s=\s matches " = " and \d+ matches the score.
while (m.find()){
System.out.print(m.group(1)+"\n");
}
打印:
團隊1 = 6
小組2 = 4
團隊3 = 2
有幾種方法可以執行此操作,但是在您的情況下,我將使用regex 。
我不懂Java,但認為像這樣的正則表達式模式應該可以工作:
Pattern compile("\\/'(.*?)'")
帶有此模式的隨機正則表達式測試器網站位於: https : //regex101.com/r/MCRfMm/1
我要說“朋友不要讓朋友使用正則表達式”,並建議對此進行解析。 內置類StreamTokenizer
將處理該作業。
private static void testTok( String in ) throws Exception {
System.out.println( "Input: " + in );
StreamTokenizer tok = new StreamTokenizer( new StringReader( in ) );
tok.resetSyntax();
tok.wordChars( 'a', 'z' );
tok.wordChars( 'A', 'Z' );
tok.wordChars( '0', '9' );
tok.whitespaceChars( 0, ' ' );
String prevToken = null;
for( int type; (type = tok.nextToken()) != StreamTokenizer.TT_EOF; ) {
// System.out.println( tokString( type ) + ": nval=" + tok.nval + ", sval=" + tok.sval );
if( type == '=' ) {
tok.nextToken();
System.out.println( prevToken + "=" + tok.sval );
}
prevToken = tok.sval;
}
}
輸出:
Input: /'Team1 = 6', while /'Team2 = 4', and /'Team3 = 2'
Team1=6
Team2=4
Team3=2
BUILD SUCCESSFUL (total time: 0 seconds)
這種技術的一個優點是,像“ Team1”,“ =”和“ 6”之類的各個標記都被分別解析,而到目前為止展示的正則表達式已經很復雜了,要隔離每個正則表達式必須變得更加復雜。這些令牌分別。
您可以在“一個斜杠上進行分割,還可以在前面加一個逗號,后跟零個或多個非斜杠字符”:
String[] body = text.split("(?:,[^/]*)?/");
public class MyClass {
public static void main(String args[]) {
String text = "/'Team1 = 6', while /'Team2 = 4', and /'Team3 = 2'";
char []textArr = text.toCharArray();
char st = '/';
char ed = ',';
boolean lookForEnd = false;
int st_idx =0;
for(int i =0; i < textArr.length; i++){
if(textArr[i] == st){
st_idx = i+1;
lookForEnd = true;
}
else if(lookForEnd && textArr[i] == ed){
System.out.println(text.substring(st_idx,i));
lookForEnd = false;
}
}
// we still didn't find ',' therefore print everything from lastFoundIdx of '/'
if(lookForEnd){
System.out.println(text.substring(st_idx));
}
}
}
/*
'Team1 = 6'
'Team2 = 4'
'Team3 = 2'
*/
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.