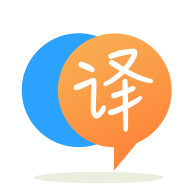
[英]JS/ES6/lodash find index of missing elements of two multidimensional arrays
[英]Javascript find index of missing elements of two arrays
我有以下JavaScript,其中有一些列表:
var deleteLinks = $(".remove-button .remove-from-cart");
deleteLinks.on('click', function(ev){
ev.preventDefault();
console.log("registered: " + deleteLinks);
var currentHTML = $('.product');
var currentText = $('.product .product-details .name-header');
var newHTML ;
$.ajax({
url: this.href,
type: "GET",
dataType: "html",
success: function(data) {
newHTML = $(data).find('.product .product-details .name-header');
for(i = 0; i < newHTML.length; i++){
console.log("new: " + newHTML[i].innerText);
console.log("old: " + currentText[i].innerText);
}
}
});
});
變量currentHTML
包含一個作為容器的div數組。 currentText
包含每個容器名稱的數組。 AJAX響應( newHTML
)中收到的變量包含一個名稱數組。 某些用戶交互后,此數組將更新。 currentText
變量的一個或多個條目可能會丟失,我想找到它們的索引,以便將其從容器( currentHTML
)中刪除。
有人可以幫我找到currentText
和檢索到的數據( newHTML
)之間缺少元素的索引嗎?
為了比較兩個數組的值,有許多不同的方法可以簡單地查看一個值是否在另一個數組中。 您可以使用array.indexOf(value)
返回元素在另一個數組中的位置,如果結果大於預期的-1
,則表示存在該元素或相反。 您也可以使用array.includes(value)
,另一種方法是使用!array.includes(value)
來簡單查看是否不存在!array.includes(value)
。
因此,知道我們可以使用!array.includes(value)
來查看數組是否不包含值。 現在,我們必須遍歷一個數組以獲取條目,並與另一個數組進行比較以查找不在另一個數組中的項目。 我們將array.forEach()
使用array.forEach()
。 我們可以使用array.some()
設計更多的遞歸函數,但是我只是想給你一些簡單的例子。
范例1。
// Data let a = [1, 2, 3, 4, 5]; // Array A let b = [1, 0, 9, 3, 5]; // Array B // Basic principals function check(a, b) { // Loop through A using array.some() => value a.forEach(value => { // B did not include value if (!b.includes(value)) { // Output console.log("B doesn't have", value, "at position", a.indexOf(value), "in A") } }); // Loop through B using array.some() => value b.forEach(value => { // A did not include value if (!a.includes(value)) { // Output console.log("A doesn't have", value, "at position", b.indexOf(value), "in B") } }); } // We are checking both Arrays A and B check([1, 2, 3, 4, 5], [1, 0, 9, 3, 5]);
例子2
讓我們為數組元素制作一個原型,然后針對數組B調用比較函數,而不僅僅是輸出到控制台進行演示。 如果該值不在數組B中,但實際上在數組A中 [value, position]
我們將返回一個帶有[value, position]
數組。
// Data let a = [1, 2, 3, 4, 5]; // Array A let b = [1, 0, 9, 3, 5]; // Array B /* Check an array for non-duplicates against another array. If the value of item in array A is present in array B, return [false, -1]. If value of item in array A is not present in B, return value and position of value in array A. */ Array.prototype.check = function(b) { /* * return if true * [value, position] * else * [false, -1] * */ return a.map(v => { return (!b.includes(v) ? [v, a.indexOf(v)] : [false, -1]) }) }; // Array A is checking against array B console.log(a.check(b)); /* Output: (A checking against B) [ [ false, -1 ], // Array element 1 found in B [ 2, 1 ], // Array element 2 not found in B, and has position 1 in A [ false, -1 ], // Array element 3 found in B [ 4, 3 ], // Array element 4 not found in B, and has position 3 in A [ false, -1 ] // Array element 5 found in B ] */
假設您在數組A
具有唯一元素,而在數組B
只有一個元素。 我們可以使用set來添加數組A
所有元素,並在遍歷Array B
時從集合中刪除這些元素。 使用findIndex
查找集合中剩余的元素。
const a = [1,2,3,4,5];
const b = [2,1,5,4];
let set = new Set();
a.forEach(ele => set.add(ele));
b.forEach(ele => {
if(set.has(ele)){
set.remove(ele);
}
});
let res = [];
for(let s of set) {
if(a.findIndex(s) !== -1) {
res.push({
arr: a,
pos: a.findeIndex(s)
});
}else {
res.push({
arr: b,
pos: b.findeIndex(s)
});
}
}
res
數組包含索引和元素所在的數組。
如果可以訪問lodash ,則可以使用_.difference一行解決此問題。
var a = ['a', 'b', 'c'], b = ['b'], result = []; _.difference(a, b).forEach(function(t) {result.push(a.indexOf(t))}); console.log(result);
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.11/lodash.js"></script>
從您的代碼中可以看到,您需要比較兩個對象的innerText並找到丟失的元素的索引。
var missing_indices = [];
for(var i=0;i<currentText.length;i++){
var found = false;
for(var j=0;j<newHTML.length;j++){
if(currentText[i].innerText == newHTML[j].innerText){
found = true;
break;
}
}
if(!found){
missing_indices.push(i);
}
}
然后使用missing_indices從currentHTML中刪除丟失的元素
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.