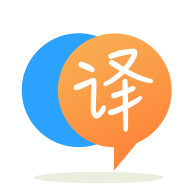
[英]Java: is this way of finding the maximum value in an integer array correct?
[英]Maximum Integer Value java
我正在嘗試從Geeksforgeeks解決最大整數值問題。
問題如下:給定數字S(0-9),您的任務是通過在行進時在數字之間放置'*'或'+'運算符來找到可從字符串獲得的最大值從字符串的左到右,一次拾取一個數字。
輸入:輸入的第一行包含T,表示測試用例的數量。 隨后是T個測試用例。 每個測試用例包含一行表示該字符串的輸入。
輸出:對於每個測試用例,打印獲得的最大值。
這是我所做的:
class GFG
{
public static void sort(int[] numbers)
{
int n = numbers.length;
for (int i = 1; i < n; ++i)
{
int key = numbers[i];
int j = i - 1;
while (j >= 0 && numbers[j] > key)
{
numbers[j + 1] = numbers[j];
j = j -1 ;
}
numbers[j + 1] = key;
}
System.out.println(numbers.length - 1);
}
public static void main (String[] args)
{
Scanner sc = new Scanner(System.in);
int testCases = sc.nextInt();
int [] maxNum;
for(int i = 0; i< testCases; i++)
{
String numbers = sc.nextLine();
char[] cNumbers = numbers.toCharArray();
maxNum = new int [cNumbers.length];
for(int j = 0; j + 1 < cNumbers.length; j++)
{
int sum = 0;
int mult = 0;
sum = cNumbers[j] + cNumbers[j + 1];
mult = cNumbers[j] * cNumbers[j + 1];
int maxNumber = Math.max(sum, mult);
maxNum[i] = maxNumber;
}
sort(maxNum);
}
}
}
輸入示例:2 01230 891我的輸出:-1 4正確輸出:9 73
我的代碼有什么問題?
請快速瀏覽一下,如果您的數字少於兩位,則應該添加。 2或更大應該相乘。 不在PC上進行測試。
我閱讀了您的描述,但您做錯了。 請在參考站點上仔細仔細閱讀問題示例。
如moilejter的注釋中所述,您使用的sc.nextInt()
不會讀取'\\ n'並造成問題。 下一個sc.nextLine()
將僅讀取一個空字符串,並且您的程序將引發異常。
第二個問題是您必須連續計算max,並且不需要int數組(您可以計算兩個連續數字之間的max運算結果,並將它們保存在與最大整數值不對應的數組中。您只能在每個數字之間找到max兩位數,但不是所有位數的最大值)。
第三個問題是將字符用作數字會導致錯誤的結果。 (必須將它們轉換為整數),因此有一個適用於您的輸出的代碼:
public class GFG
{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int testCases = Integer.valueOf(sc.nextLine());
for (int i = 0; i < testCases; i++)
{
String numbers = sc.nextLine();
char[] cNumbers = numbers.toCharArray();
long maxUntilNow = cNumbers[0] - '0';
for (int j = 1; j < cNumbers.length; j++)
{
int numberOfThisPlace = cNumbers[j] - '0';
maxUntilNow = Math.max(maxUntilNow + numberOfThisPlace,
maxUntilNow * numberOfThisPlace);
}
System.out.println(maxUntilNow);
}
}
}
我希望這就是你想要的。
想法是讓操作員交替使用,並選擇最大的結果。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int testCases = Integer.parseInt(sc.nextLine());
for (int i = 0; i < testCases; i++) {
String numbers = sc.nextLine();
int max = 0;
for (int j = 0; j + 1 < numbers.length(); j++) {
int next = Integer.parseInt(numbers.substring(j, j+1));
if (max + next > max * next)
max = max + next;
else
max = max * next;
}
System.out.println(max);
}
sc.close();
}
}
執行后
int testCases = sc.nextInt();
緩沖區包含換行符。 因此,當執行該行時
String numbers = sc.nextLine();
它將'\\ n'讀為數字,因此您將-1作為第一個輸出。 另外,在使用任何算術運算之前,還需要將字符轉換為Integer。
sum = cNumbers[j] + cNumbers[j+1];
mult = cNumbers[j] * cNumbers[j+1];
因此,以上代碼將給您錯誤的結果。
我嘗試了以下示例並工作。
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String inputAsString = sc.nextLine();
int testCases = Integer.parseInt(inputAsString);
int maxNumber = 0;
for (int i = 0; i < testCases; i++) {
String numbers = sc.nextLine();
if(!numbers.matches("\\d+")){
System.out.println("Only numeric values are expected.");
continue;
}
char[] cNumbers = numbers.toCharArray();
int sum = 0;
int mult = 1;
for (int j = 0; j < cNumbers.length; j++) {
int nextNumber = Character.getNumericValue(cNumbers[j]);
sum = sum + nextNumber;
mult = mult * nextNumber;
maxNumber = mult > sum ? mult : sum;
sum = maxNumber;
mult = maxNumber;
}
System.out.println(maxNumber);
}
sc.close();
}
根據問題陳述,我們需要通過在字符串之間從左到右遍歷並一次拾取單個數字的方式,在數字之間放置*或+運算符來從字符串中獲取最大值。 因此,可以在O(n)中解決此問題,而無需使用任何排序算法。 解決方案背后的簡單邏輯是,只要在任何一個操作數中找到“ 0”或“ 1”,就使用“ +”,其余位置使用“ *”。 這是我成功提交的解決方案:
import java.util.*;
import java.lang.*;
import java.io.*;
class GFG {
public static void main (String[] args) {
Scanner scan = new Scanner(System.in);
int T = Integer.parseInt(scan.nextLine());
while(T-- > 0) {
String str = scan.nextLine();
maxValue(str);
}
}
static void maxValue(String str) {
long maxNumber = 0;
for(int i = 0; i < str.length(); i++) {
int n = Character.getNumericValue(str.charAt(i));
if (maxNumber == 0 || maxNumber == 1 ||
n == 0 || n == 1) {
maxNumber += n;
} else {
maxNumber *= n;
}
}
System.out.println(maxNumber);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.